Mastering Data Science – June 2023
Mastering Data Science Course - JUNE 2023
# Python is case sensitive language
print(5 + 5) # inbuilt function – prints whatever you give to it on the screen
print(“5 + 5”) # “” – used for repeating by print
print(“5 + 5 =”,5+5)
print(‘3 + 5 =’,5+5)
# variables
x = 777
y = 75
print(x+y)
print(x,“+”,y)
print(x,“+”,y,“=”,x+y)
print(x,‘+’,y,‘=’,x+y)
# variables naming rule: it should start with an alphabet, it can have numbers and _
num = 10
num1 = 101
n1m2 = 56
first_number = 56
# write a program to add 2 numbers
num1 = 45
num2 = 66
print(“Sum of two numbers is”,num1+num2)
x = 45
y = 66
print(“Sum of two numbers is”,x+y)
sdfdsagtarebgdvdzdczvdv = 45
dfsgdfsgbsysbsdfhnjdjshff = 66
print(“Sum of two numbers is”,sdfdsagtarebgdvdzdczvdv+dfsgdfsgbsysbsdfhnjdjshff)
# write a program to calculate area and perimeter of a rectangle, when their sides are given
# input -> process -> output
# area = length * breadth
# perimeter = 2 * (length + breadth)
# input:
length = 52
breadth = 31
#process:
area = length * breadth;
perimeter = 2*(length + breadth);
#output
print(“Area of the rectangle is”,area);print(“The perimeter is”,perimeter)
########### Assignment ################
# WAP to calculate area and circunference of a circle when radius is given
# WAP to calculate perimeter of a triangle who 3 sides are given
##########################################
# Basic data types – 5 types
length = 30 # int=integer = numbers without decimal values
print(“type of data = “,type(length)) # <class ‘int’>
# type() – gives the type of the data that is in the variable
length = 29.8 # float = decimal values
print(“type of data = “,type(length)) # <class ‘float’>
number1 = 5+2j
print(“type of data = “,type(number1)) #<class ‘complex’>
# imaginary numbers are the root of -ve numbers = i
print(“Doing a calculation = “, number1 * (5–2j)) # (a+b)(a-b) = a square – b square
# 25 – (-4) = 29 + 0j
# bool (boolean)- True (1) & False (0)
val1 = True # False
print(“type of data = “,type(val1))
#text -str = string
name = “Sachin Tendulkar”
print(“type of data = “,type(name))
########### Assignment ################
# WAP to calculate area and circunference of a circle when radius is given
# WAP to calculate perimeter of a triangle who 3 sides are given
##########################################
# Basic data types – 5 types
cost_price = 45
selling_price = 67
quantity = 78
profit = (selling_price – cost_price) * quantity
print(“Total profit after selling”,quantity,“items bought at”,cost_price,
“and sold at”,selling_price,“is”,profit,end=“\n“)
print(f”Total profit after selling {quantity} items bought at {cost_price} “
f”and sold at {selling_price} is {profit}“,end=“\n“) #f string
print(“Total profit after selling {} items bought at {} and sold at “
“{} is {}”.format(quantity, cost_price,selling_price,profit))
print(“Total profit after selling {0} items bought at {2} and “
“sold at {3} is {1}”.format(quantity, profit,cost_price,selling_price))
quantity = int(“14”)
# int(), str(), bool(), float(), complex()
total_cost = 500
cost_per_quantity = total_cost/quantity
# implicit and explicit conversion
print(f”Cost per items for quantity {quantity} and total cost {total_cost} is {cost_per_quantity:.2f}“)
player = “Kohli”
country = “India”
position =“Opener”
print(f”This is {player:<15} who plays for {country:>15} as {position:^20} in cricket.”)
player,country,position = “Mubwangwa”, “Zimbabwe”,“Wicket-Keeper”
print(f”This is {player:<15} who plays for {country:_>15} as {position:.^20} in cricket.”)
print(“I am fine \nhow are \nyou”) #escape character (works only inside quotes) \n, \t
# \n will create newline
print(“\\n will create newline”)
# \\n will create newline
print(“\\\\n will create newline”)
print(f”{player} \n{country} \n{position}“)
print(“First line content”,end=” – “)
print(“Second line content”,end=“\n“)
value = 50
print(“Decimal number = “,int(value))
print(“Hexa number = “,hex(value)) #0x
print(“Octal number = “,oct(value)) #0o
print(“Binary number = “,bin(value)) #0b
value = 0b110011001100
print(“Decimal number = “,int(value))
print(“Hexa number = “,hex(value)) #0x
print(“Octal number = “,oct(value)) #0o
print(“Binary number = “,bin(value)) #0b
#### Operations –
##Arithmematic operations
val1, val2 = 54, 5
print(val1 + val2) # 55
print(val1 – val2) #
print(val1 * val2) #
print(val1 / val2) #
print(val1 // val2) #integer division – will return only the integer part
print(val1 ** val2) # power (raise to)- 54 to the power of 5
print(54 ** (1/2)) #square root of 54
print(val1 % val2) # modulo – remainder
#Relational (comparison operator): Output is always a bool value
# == != < > <= >=
val1,val2 = 15, 15
print(val1 == val2) # is val1 equal to val2 ? – T
print(val1 != val2) # F
print(val1 < val2) # F
print(val1 > val2) # F
print(val1 <= val2) # T
print(val1 >= val2) # T
## Logical operations
#Comparison: > < >= <= == !=
#Logical: and or not: input and output both values are boolean
# Prediction 1: Sachin or Sehwag will open the batting
# Prediction 2: Sachin and Sourav will open the batting
# Actual: Sachin and Rahul opened the batting
print(True or False) # OR gives True even if one value is True
print(True and False) # AND gives False even if one value is False
print(5 > 6 and 8<10) # False and True
print(3 + 4 * 4)
val1,val2,val3 = 10,15,10
print(val1 > val2 and val2 ==val3 or val3==val1 and val1!=val3 and val2>=val3 or val3 !=val1)
print(3 + 5 * 2 – 6 * 3 + 5 – 3 * 4 / 3)
#BIT WISE Operators: and (&) or (|), left shift and right shift
print(50 & 30)
print(bin(50), bin(30))
print(int(0b10010))
print(50 | 30)
print(int(0b111110))
print(312 >> 3)
print(12 << 3)
########### Refer the document for assignment ########
# Conditions – if, elif else
# Pass or Fail
print(“PASS”)
print(“FAIL”)
avg = 5
if avg >=35:
print(“PASS”)
print(“Congratulations on great result”)
else:
print(“Result: FAIL”)
#if avg > 90 – A+, 80 -A, 70: B+, 60: B-, 50: C, >35: D, <35: E
avg = float(input(“Enter the average value of the student: “))
if avg>=90:
print(“Super Duper Result: A+”)
elif avg>=80:
print(“Grade: A-“)
elif avg>=70:
print(“Grade: B+”)
elif avg >=60:
print(“Grade: B-“)
elif avg >=50:
print(“Grade: C”)
elif avg >=35:
print(“Grade: D”)
else:
print(“Grade: E”)
### input()
val = int(input(“Enter a number: “)) #takes value from the user
print(val)
print(type(val))
# by default input() takes in value as string
#### Take marks of 5 subjects from the user and calculate sum and average and then assign the grade
# based on above discussion
Write a program to input a value from the user and check if its positive, negative or zero.
if its positive, then check if its odd or even. For even numbers, check if they are multiple of 4
”’
#Nested if conditions
num = int(input(“Enter a number: “))
if num >0:
print(num,“is positive”,end=“”)
if num %2 ==0:
print(” and also even”)
if num%4==0:
print(num,“is divisible by 4.”)
else:
print(” and also odd”)
elif num<0:
print(num, “is negative”)
else:
print(num,“is neither positive not negative”)
#Assignment: Write a program to input 3 sides of a triangle anc check
#if they are: right angled, isoceles, scalene or equilateral
# short form of one line if-else condition
a,b = 10,5
if a>b:
print(a,” is greater”)
else:
print(b, ” is greater”)
#short
result = a if a>b else b
print(result,“is greater”)
## let’s take example of 3 values and check which number is highest
a,b,c = 500,100,500
if a>b:
if a>c:
print(a,“is highest”)
else:
print(c, “is highest”)
else: # b is greater than a
if b>c:
print(b,“is highest”)
else:
print(c, “is highest”)
result = a if a>b else b
result = result if result>c else c
print(result,“is highest – II”)
if a>=b and a>=c:
print(a,“is highest – III”)
elif b>=a and b>=c:
print(b,“is highest – III”)
else:
print(c,“is highest – III”)
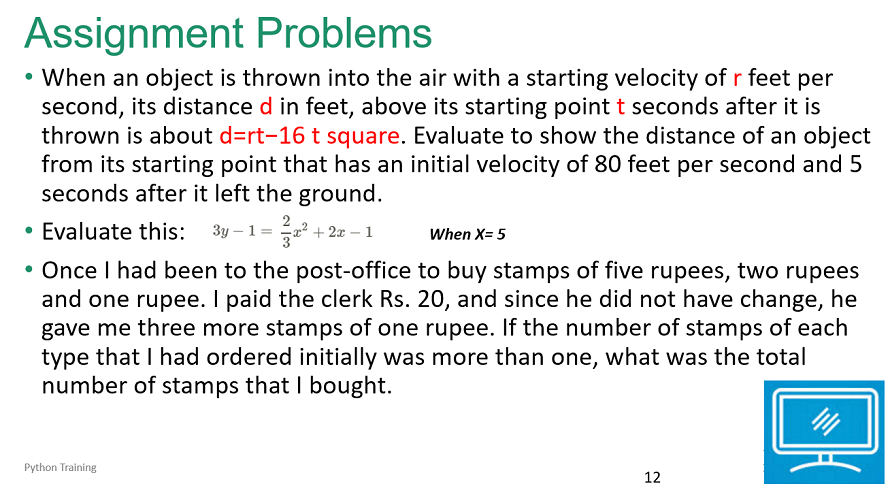
#1. FOR – when you know exactly how many times to repeat
#2. WHILE – when you dont know exactly how many times but you know when to stop
# range() generates range of values from given =position to the <final position with given increment
range(1,49,6) #range(=start,<end,increment): 1,7,13,19,25,31,37,43
range(5,11) #range(start,end), increment is default = 1: 5,6,7,8,9,10
range(5) # range(end), start default = 0, increment=1: 0,1,2,3,4
# for loop using range
for variable in range(1,49,6):
print(“=================”)
print(“HELLO : “,variable )
for variable in range(5,11):
print(“xxxxxxxxxxxxxxxxx”)
print(“HELLO : “,variable )
for variable in range(5):
print(“………..”)
print(“HELLO : “,variable )
# for counter in range_of_values
# while true condition
final,start = 5,0
while start< final:
print(“I am in while now, start = “,start)
start +=1 #start = start+1
print(“Thats it for today”)
“””
* * * * *
* * * * *
* * * * *
* * * * *
* * * * *
“””
for j in range(n):
for i in range(n):
print(“*”,end=” “)
print()
”’
*
* *
* * *
* * * *
* * * * *
”’
#z=0
for j in range(n):
#z+=1 # z=z+1
for i in range(j+1):
print(“*”,end=” “)
print()
“””
* * * * *
* * * *
* * *
* *
*
“””
for j in range(n):
for i in range(n-j):
print(“*”,end=” “)
print()
”’
*
* *
* * *
* * * *
* * * * *
”’
for j in range(n):
for k in range(n-j-1):
print(” “,end=“”)
for i in range(j+1):
print(“*”,end=” “)
print()
##### Assignment ##########
#Print below pattern:
“””
* * * * *
* * * *
* * *
* *
*
“””
## assignment code
”’
A pattern
”’
n=15
for j in range(n):
for k in range(n-j-1):
print(” “,end=“”)
if j==0 or j==int(round(n/2)):
for i in range(j+1):
print(“* “,end=“”)
else:
print(“*”,end=“”)
print(” “*j,end=“”)
print(“*”,end=“”)
print()
#### Assignment
# Practice patterns A to Z
for i in range(1,11):
print(f”{i:>2} * {num:>2} = {num*i: >2}“,end=” “)
print()
user_says = “y”
while user_says ==“y”:
print(“Hello”)
user_says = input(“Enter y to print again or any other key to stop:”)
check = True
while check:
print(“Hello Again”)
user_says = input(“Enter y to print again or any other key to stop:”)
if user_says!=“y”:
check = False
while True:
print(“Hello Again Again”)
user_says = input(“Enter y to print again or any other key to stop:”)
if user_says!=“y”:
break
user_says = input(“Enter y to print again or any other key to stop:”)
while user_says ==“y”:
print(“Hello”)
user_says = input(“Enter y to print again or any other key to stop:”)
st,end,co=11,100,11
for i in range(st,end,co):
if end%co==0:
if i == (end // co-1) * st:
print(i)
else:
print(i, end=“, “)
else:
if i==end//co*st:
print(i)
else:
print(i, end=“, “)
st,end,co=11,100,11
for i in range(st,end,co):
if i+co>=end:
print(i)
else:
print(i, end=“, “)
”’
Find 10 multiples of even number
If the number is negative then do not execute
if you come across multiple value as a multiple of 12 then skip it
if you come across multiple value as a multiple of 20 then stop it
”’
while True:
num = int(input(“Enter a number: “))
if num>=0:
for i in range(1,11):
if num*i%12 ==0:
continue
if num*i%20 ==0:
break
print(f”{num} * {i} = {num*i}“)
ch=input(“Hit any key to stop: “)
if len(ch)!=0:
break
#ATM Transaction
while True:
print(“Enter your choice from below menu:”)
print(“1. Deposit \n2. Withdraw \n3. Account Transfer \n4. Statement \n5. Quit”)
ch = input(“Enter your option here: “)
if ch==“1”:
ch=input(“Enter the amount: “)
print(“… continue witht he logic”)
elif ch==“2”:
print(“Logic is not ready”)
elif ch==“3”:
print(“Logic is not ready”)
elif ch==“4”:
print(“Logic is not ready”)
elif ch==“5”:
print(“Thank you for Banking with us, Have a Good Day!”)
break
else:
print(“Invalid Option, please try again!”)
continue
# Check for Prime
is_prime = True
for i in range(2,num//2+1):
if num %i==0:
is_prime = False
break
if is_prime:
print(f”{num} is a Prime number”)
else:
print(f”{num} is not a Prime number”)
# generate prime numbers between 5000 and 10000
for num in range(5000,10001):
is_prime = True
for i in range(2,num//2+1):
if num %i==0:
is_prime = False
break
if is_prime:
print(f”{num} “,end=“, “)
# Assignments:
## 1. WAP to generate first 5 fibonacci numbers: 0,1, 1, 2,3,5,8,13,21…
import random
num = random.randint(1,100)
#print(“Number = “,num)
#print(“===============”)
counter = 0
while True:
guess = int(input(“Guess the number (1-100): “))
if guess <1 or guess>100:
print(“Invalid number! Try again…”)
continue
counter+=1 #counter = counter + 1
if guess == num:
print(f”Congratulations! You have successfully guessed the number is {counter} steps.”)
break
elif guess < num:
print(“Sorry! Your guess is lower. Try again…”)
else:
print(“Sorry! Your guess is higher. Try again…”)
############
# guess the number: Computer v Computer
import random
num = random.randint(1,100)
print(“Number = “,num)
print(“===============”)
counter = 0
st,en = 1,100
while True:
#guess = int(input(“Guess the number (1-100): “))
guess = random.randint(st,en)
print(“Guess number = “,guess)
if guess <1 or guess>100:
print(“Invalid number! Try again…”)
continue
counter+=1 #counter = counter + 1
if guess == num:
print(f”Congratulations! You have successfully guessed the number is {counter} steps.”)
break
elif guess < num:
print(“Sorry! Your guess is lower. Try again…”)
st=guess+1
else:
print(“Sorry! Your guess is higher. Try again…”)
en=guess-1
################
# guess the number: Computer v Human (automation)
import random
num = random.randint(1,100)
print(“Number = “,num)
print(“===============”)
counter = 0
st,en = 1,100
while True:
guess = (st+en)//2
#guess = random.randint(st,en)
print(“Guess number = “,guess)
if guess <1 or guess>100:
print(“Invalid number! Try again…”)
continue
counter+=1 #counter = counter + 1
if guess == num:
print(f”Congratulations! You have successfully guessed the number is {counter} steps.”)
break
elif guess < num:
print(“Sorry! Your guess is lower. Try again…”)
st=guess+1
else:
print(“Sorry! Your guess is higher. Try again…”)
en=guess-1
###########################
# guess the number: Computer v Human (automation)
import random
import time
TOTAL = 0
max = 100000
start_time = time.time()
for i in range(max):
num = random.randint(1,100)
#print(“Number = “,num)
#print(“===============”)
counter = 0
st,en = 1,100
while True:
guess = (st+en)//2
#guess = random.randint(st,en)
print(“Guess number = “,guess)
if guess <1 or guess>100:
#print(“Invalid number! Try again…”)
continue
counter+=1 #counter = counter + 1
if guess == num:
print(f”Congratulations! You have successfully guessed the number is {counter} steps.”)
TOTAL +=counter
break
elif guess < num:
#print(“Sorry! Your guess is lower. Try again…”)
st=guess+1
else:
#print(“Sorry! Your guess is higher. Try again…”)
en=guess-1
end_time = time.time()
print(“Total steps = “,TOTAL)
print(“Average number of steps = “,TOTAL/max,” total time taken to run”,max,“steps is”,(end_time – start_time))
# Average number of steps = 5.80429 total time taken to run 100000 steps is 36.42937159538269
####################################
# guess the number: Computer v Computer (measure steps)
import random
import time
TOTAL = 0
max = 100000
start_time = time.time()
for i in range(max):
num = random.randint(1,100)
#print(“Number = “,num)
#print(“===============”)
counter = 0
st,en = 1,100
while True:
#guess = (st+en)//2
guess = random.randint(st,en)
print(“Guess number = “,guess)
if guess <1 or guess>100:
#print(“Invalid number! Try again…”)
continue
counter+=1 #counter = counter + 1
if guess == num:
print(f”Congratulations! You have successfully guessed the number is {counter} steps.”)
TOTAL +=counter
break
elif guess < num:
#print(“Sorry! Your guess is lower. Try again…”)
st=guess+1
else:
#print(“Sorry! Your guess is higher. Try again…”)
en=guess-1
end_time = time.time()
print(“Total steps = “,TOTAL)
print(“Average number of steps = “,TOTAL/max,” total time taken to run”,max,“steps is”,(end_time – start_time))
# Average number of steps = 7.4783 total time taken to run 100000 steps is 28.571797370910645
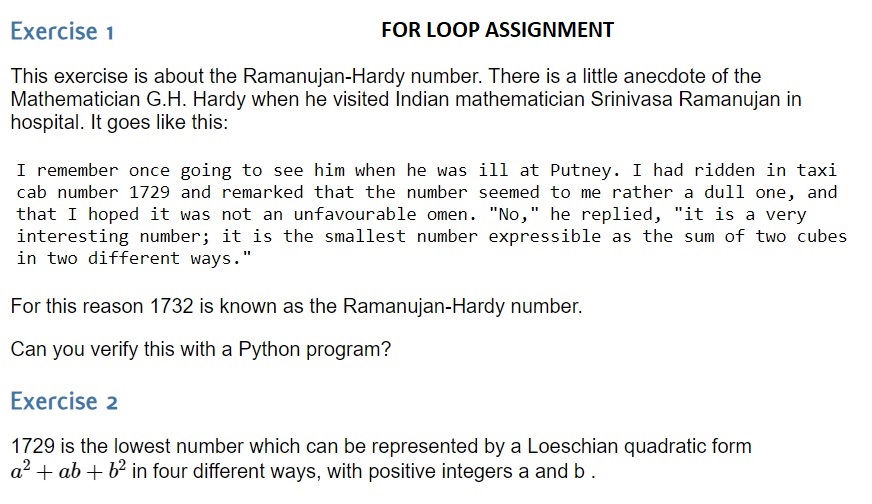
txt1 = “HELLO”
txt2 = ‘HI’
print(txt1, txt2)
txt3 = ”’I am fine”’
txt4 = “””I am here”””
print(txt3, txt4)
txt5 = ”’I am fine here
Hope you are
doing well
too. So how are you”’
txt6 = “””I am here
Where are you?
How are you?”””
print(txt5)
print(txt6)
# I’m fine
print(“I’m fine”)
# He asked,”How are you?”
print(‘He asked,”How are you?”‘)
# \ – escape sequence
print(“I am \nfine”)
print(‘I\’m fine’)
txt7 = “HELLO”
print(len(txt7))
print(txt7[1])
print(txt7[1],txt7[4])
print(txt7[1:4]) #ELL
print(txt7[:4]) #HELL
print(txt7[2:]) #LLO
print(txt7[:]) #HELLO
txt8 = input(“Enter a text:”)
length = len(txt8)
print(txt8[-1], txt8[length-1]) #last character
print(txt8[0], txt8[-length]) #first character
print(txt8[1:4], txt8[-4:-1]) # ELL
print(txt8[:4], txt8[-5:-1], txt8[:-1]) # HELL
print(txt8[1:], txt8[1:5], txt8[-4:]) # ELLO
a = “HELLO”
b = “THERE”
print(a +” “+ b)
c = (a+” “) * 4
print(c)
print(c[:-1])
#a[0]=”h” #’str’ object does not support item assignment – IMMUTABLE
a = “h”+a[1:]
print(a)
for i in b:
print(i)
for j in range(len(b)):
print(j, b[j])
### ### String Methods #####
a= “heLLLLLLLLLlllo”
## Refer String Methods – https://docs.python.org/3.11/library/stdtypes.html#string-methods
print(a.islower())
print(a.lower())
ch = input(“Enter Yes to continue: “)
if ch.upper()==“YES”:
print(“YES”)
num1 = input(“Enter a number: “)
if num1.isdigit():
num1 = int(num1)
else:
print(“Exiting because of invalid number”)
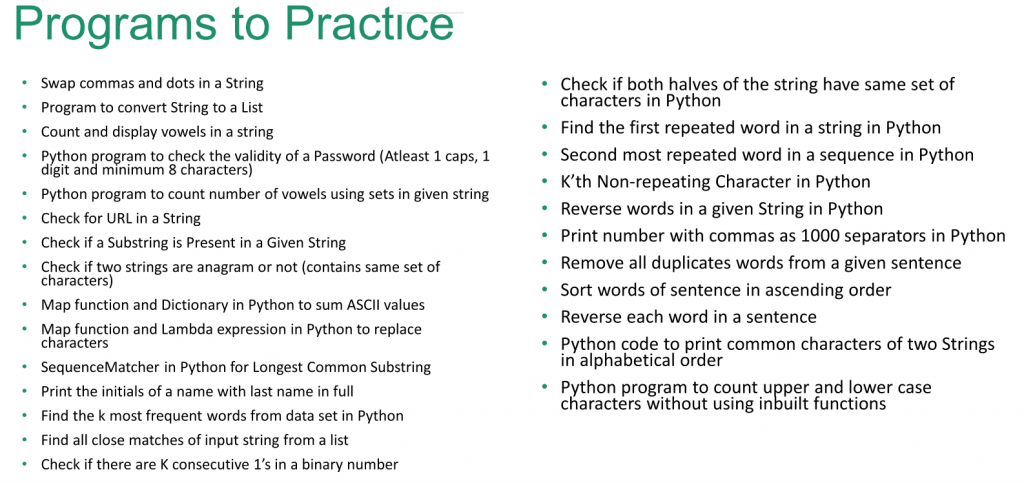
str1 = “Hello How Are You?”
print(“Capitalize the content: “,str1.capitalize())
print(str1.isalpha())
print(“”.join(str1.split(” “)))
str1_1 = “”.join(str1.split(” “))
print(“is alpha for joined text: “,str1_1.isalpha())
if str1_1.isalpha():
print(“Your name has been saved into the database”)
else:
print(“Invalid name, try again!”)
str2 = ” name “
print(str2.isspace())
print(“Strip txt:”,str2.strip())
print(“Strip txt:”+str2.strip())
str3 = “Hello How Are You are you doing you are?”
print(“count of are:”,str3.lower().count(“are”, 0, 21))
print(“Finding find:”, str3.find(“find”)) #returns the position of first occurrence
print(“Finding find:”, str3.find(“you”))
print(“Finding find:”, str3.find(“you”,23,35))
## Assignment – find the position of all the occurrences and print the output in below format:
# found 2 words and they are at positions 22 and 32
print(str3.startswith(“Hello How”))
print(str3.endswith(“?”))
# Replace –
print(str3.replace(“you”,“YOUR”))
print(str3.replace(“you”,“YOUR”, 1))
print(str3[:24]+str3[24:].replace(“you”,“YOUR”, 1))
print(str3) # original text will not change becasue of any method as strings are immutable
list1 = [20,30.0, True, “Hello”, [2,3,5]]
print(“Datatype: “, type(list1))
print(“Number of values = “,len(list1))
print(list1[0], type(list1[0]))
print(list1[-1], type(list1[-1]))
print([1,2,3]+[10,20,30])
print([1,2,3] *3)
for i in list1:
print(i)
for i in range(len(list1)):
print(i, “: “,list1[i])
for i in list1[-1]:
print(i)
list1[0] = “Twenty”
print(list1)
#Tuple: linear ordered immutable collection
t1 = (20,30.0, True, “Hello”, [2,3,5])
print(type(t1))
print(t1[0],t1[-1])
#t1[0]= 5 # ‘tuple’ object does not support item assignment
# you can convert list to tuple and tuple to list
l1 = [3,5,9]
print(type(l1))
l1 = tuple(l1)
print(type(l1))
l1 = list(l1)
print(type(l1))
t1 = (2,100,1000,10000,100)
t2 = (2,100,1000,10000,100000)
if t1 > t2:
print(“T1”)
elif t2 > t1:
print(“T2”)
else:
print(“They are equal”)
print(“Count = “,t1.count(100))
print(“Index = “,t1.index(100))
l1 = list(t1)
print(“Count = “,l1.count(100))
print(“Index = “,l1.index(100))
l1.append(99)
l1.append(199)
l1.append(299)
print(“L1 after append= “,l1)
#insert & append – both will add members to the list
l1.insert(2,555)
print(l1)
l1.insert(200,444)
print(l1)
total = 0
marks=[]
for i in range(5):
m1 = int(input())
marks.append(m1)
total += m1
print(“Marks obtained are:”,marks)
print(“Total = “,total)
#Marks obtained are: [50, 60, 70, 80, 90]
# LIFO – stack
total = 0
marks=[]
for i in range(5):
m1 = int(input())
marks.insert(0,m1)
total += m1
print(“Marks obtained are:”,marks)
print(“Total = “,total)
#Marks obtained are: [90, 80, 70, 60, 50]
# using sum() to do total
marks=[]
for i in range(5):
m1 = int(input())
marks.append(m1)
total = sum(marks)
print(“Marks obtained are:”,marks)
print(“Total = “,total)
l1 = [90, 80, 70, 60, 50]
idx =10
if len(l1) >idx:
l1.pop(10) #default is -1: pop works on index
print(l1)
if 90 in l1:
l1.remove(90) #remove works on value
print(l1)
if l1.count(90) > 0:
l1.remove(90)
l2 = [90, 80, 70, 60, 50]
print(sum(l2))
l2 = [80, 90, 60, 50, 70]
l2.reverse()
print(l2)
l2.sort()
l2.sort(reverse=True)
print(l2)
# extend
l1 = [2,4,6]
l2 = [4,5,9]
l3 = l1 + l2
print(l3)
l1.extend(l2) # l1 = l1 + l2
print(l1)
l3 = [2, 4, 6, 4, 5, 9]
l4 = l3 # deep copy [renaming= duplicate name]
l5 = l3.copy() #shallow copy [taking a photocopy]
print(“l3 = “,l3)
print(“l4 = “,l4)
print(“l5 = “,l5)
l3.append(11)
l4.append(22)
l5.append(33)
print(“After append:”)
print(“l3 = “,l3)
print(“l4 = “,l4)
print(“l5 = “,l5)
”’
Assignment: Write a program using List to read the marks of
5 subjects for 5 students and find the highest marks for:
i) Each Student
ii) Each Subject
e.g. [[44,77,66,55,88],[64,54,74,84,94],[99,66,44,77,55],[83,74,65,56,67],[91,81,71,61,51]]
Highest for Student 1: 88
2: 94
3: 99
4: 83
5: 91
Highest for Subject 1: 99
2: 81
3: 74
4: 84
5: 94
”’
all_marks = []
for i in range(5):
students=[]
print(“Marks for Student “,i+1,“: “)
for j in range(5):
m = int(input(“Marks obtained in Subject “+str(j+1)+“: “))
students.append(m)
all_marks.append(students)
print(“All marks = “,all_marks)
# [[44,77,66,55,88],[64,54,74,84,94],[99,66,44,77,55],[83,74,65,56,67],[91,81,71,61,51]]
#All marks = [[44, 77, 66, 55, 88], [64, 54, 74, 84, 94],
# [99, 66, 44, 77, 55], [83, 74, 65, 56, 67], [91, 81, 71, 61, 51]]
l3 = [2, 4, 6, 4, 5, 9]
l3.clear()
print(l3)
#Tuple
t1 = ()
print(type(t1))
t1 = (100,)
print(type(t1))
t1 = (100,200)
print(type(t1))
######### DICTIONARY ##########
# dictionary -> unordered mutable collection
# dict = {key:value}
dict1 = {}
print(type(dict1))
dict1 = {1:500,“Two”: “Sachin Tendulkar”,“some index”:999,“City”:“Mumbai”, 10:1000}
#Key shouldnt be duplicated
print(dict1[1])
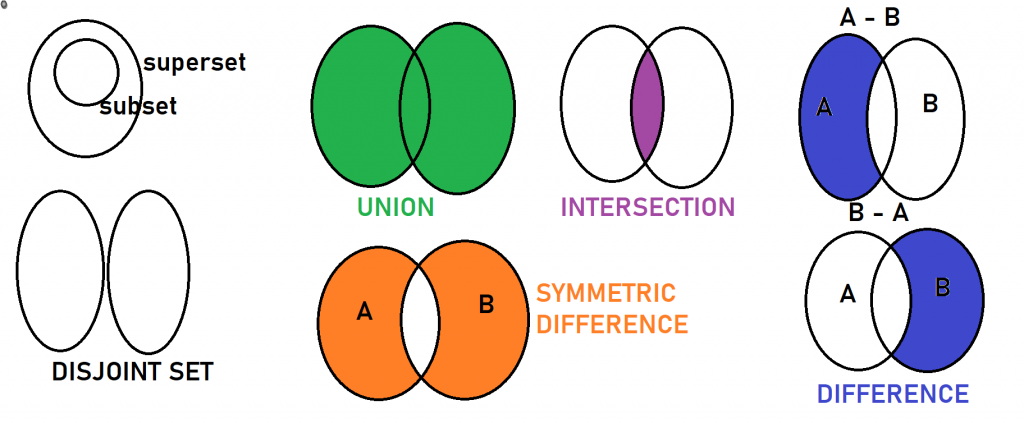
dict1 = {}
print(type(dict1))
all_info= {}
# all_info= {“”:[],””:[],””:[]}
for i in range(3):
rno = input(“Enter the Roll No. “)
marks=[]
for j in range(3):
m = float(input(“Enter the marks: “))
marks.append(m)
t_dict = {rno:marks}
all_info.update(t_dict)
print(“All Info: \n“,all_info)
t1 = (3,6,9)
a,b,c = t1
#a,b= t1 ValueError: too many values to unpack (expected 2)
# a,b,c,d = t1 ValueError: not enough values to unpack (expected 4, got 3)
all_info = {‘101’: [3.0, 4.0, 5.0], ‘102’: [7.0, 8.0, 9.0], ‘103’: [4.0, 5.0, 6.0],
‘104’:[2.0, 4.0, 6.0], ‘105’:[3.0,6.0, 9.0]}
chk_rno = input(“Enter the roll number for information: “)
print(all_info.keys())
print(all_info.values())
print(all_info.items())
for k in all_info.items():
if k[0]==chk_rno:
print(k[1])
found = False
for k,v in all_info.items():
if k==chk_rno:
print(v)
found = True
if not found:
print(“Sorry, roll no is not in the list”)
chk_rno = input(“Enter the roll number to delete: “)
for k in all_info.items():
if k[0]==chk_rno:
all_info.pop(chk_rno)
break
print(“After removal: \n“,all_info)
all_info.popitem() #removes last updated value
print(“After popitem: \n“,all_info)
all_info = {‘101’: [3.0, 4.0, 5.0], ‘102’: [7.0, 8.0, 9.0], ‘103’: [4.0, 5.0, 6.0],
‘104’:[2.0, 4.0, 6.0], ‘105’:[3.0,6.0, 9.0]}
#copy
all_info1 = all_info #Deep copy
all_info2 = all_info.copy() # Shallow copy
print(“First:”)
print(all_info)
print(all_info1)
print(all_info2)
all_info.update({9:“Orange”})
all_info1.update({10:“Apple”})
all_info2.update({11:“Mango”})
print(“Second:”)
print(all_info)
print(all_info1)
print(all_info2)
### SETS : linear unordered collection
set1 = {3,5,6,8}
print(type(set1))
list1 = [‘Mango’,‘Mango’,‘Mango’,‘Mango’,‘Mango’]
set1 = {‘Mango’,‘Mango’,‘Mango’,‘Mango’,‘Mango’}
print(len(list1))
print(len(set1)) # set will have unique values
list1 = [“A”,“B”,“C”,“D”,“E”]
list2 = [“C”,“D”,“A”,“B”,“E”]
set2 = {“C”,“D”,“A”,“B”,“E”}
print(set2) # order doesnt matter in set
# SETs are used to represent values
set2 = {“C”,“D”,“A”,“B”,“E”}
set1 = {“C”,“D”,“A”,“F”,“G”}
#frozen_set is the immutable version of set
#sets are mutable
set1.add(“Z”)
set3 = {“P”,“Q”}
set2.update(set3)
print(“Set1: “,set1)
print(“Set2: “,set2)
set2.pop()
print(“Set2: “,set2)
set2.remove(“Q”)
#Operations on sets: Union, Intersection, Difference, difference_update
print(“Set1: “,set1)
print(“Set2: “,set2)
#union – combining all the elements from the sets
print(set1.union(set2))
print(set1 | set2)
#intersection: only the common elements
print(set1.intersection(set2))
print(set1 & set2)
#difference
print(set1 – set2)
print(set2 – set1)
print(set1.difference(set2))
#symmetric difference
print(set1 ^ set2)
print(set2 ^ set1)
print(set1.symmetric_difference(set2))
set1.union(set2) #union update – set1 will have new values
set1.intersection_update(set2) # set1 will be updated with output values
set1.difference_update(set2)
set1.symmetric_difference_update(set2)
set1.issubset(set2)
# inbuilt functions: print(), input(), ….
# user defined functions
#just created a function called as myquestions()
# required positional argument
def myquestions(choice,a):
print(“Choice =”,choice,“and A =”,a)
if choice==1:
print(“Whats your name?”)
print(“What are you doing here?”)
print(“When will you be done?”)
else:
print(“Whats your Work here?”)
print(“What are your interests?”)
print(“Why dont you stand out?”)
return “Job Done”
myquestions(1,5) #passing while calling
#print(“My Out = “,myout)
print(“Another time let me repeat”)
myout = myquestions(0,4)
print(“My Out = “,myout)
str1 = “HELLO”
output = str1.lower() #returns a value
print(output)
list1 = [5,1,9,6,2,7,3]
output2 = list1.reverse() #doesnt return a value
print(output2)
print(list1)
out=print(“heloo”)
print(“OUT=”,out) #doesnt return
out = input() #returns
print(“OUT=”,out)
# non-required = default argument
# non-positional = keyword argument
def myquestions(choice,a=-99): #default value
print(“Choice =”,choice,“and A =”,a)
if choice==1:
print(“Whats your name?”)
print(“What are you doing here?”)
print(“When will you be done?”)
else:
print(“Whats your Work here?”)
print(“What are your interests?”)
print(“Why dont you stand out?”)
return “Job Done”
myquestions(1,5) #passing while calling
myquestions(1)
myquestions(a=1,choice=5) #keyword argument
def myfavoritefruits(f1,*fruits, **interests): #* and ** for variable number of arguments
# * – as tuple, ** will read as dictionary
if len(fruits)==0:
print(“My favorite fruit is”,f1)
else:
#tuple(list(fruits).append(f1))
print(“My favorite fruits are:”,tuple(set(fruits)))
if len(interests)!=0:
print(“My other interests are: “)
for i,j in interests.items():
print(“Favorite”,i,“is”,j)
myfavoritefruits(“Guava”,“Orange”)
myfavoritefruits(“Orange”)
myfavoritefruits(“mango”,“mango”,“mango”,“apple”, color=“red”,place=“hyderabad”,food=“Biryani”)
def myfun1(x,y):
print(f”x={x}, y={y} and sum is {x+y}“)
myfun1(10,20)
a,b = 5,6
myfun1(a,b)
x,y=2,8
myfun1(x=y,y=x)
myfun1(y,x)
#Scope of a variable
PI = 3.14
def myfun1(x,y):
print(f”x={x}, y={y} and sum is {x+y}“)
global g
print(“Print G =”,g)
h=70
g=20
print(“Local: “,g)
g=100 #Global variable
myfun1(10,20)
#print(“H =”,h)
def my_fun2(n):
all_values = []
for i in range(n):
all_values.append(input(“Enter values: “))
return all_values
val = my_fun2(10)
print(val)
# functions and variables – for class and specific for objects
class Library:
total_books = 0 #class level variable
var2 = –1
name = “ABC International School Library”
def __init__(self,title, author,copies=1): # auto called when object is created
print(“Adding books to the library”)
Library.total_books+=1
self.total_copies = copies #object level variable
self.title = title
self.author = author
def display_detail(self):
print(“================================”)
print(“Title \t\t Author \t\t\t Copies”)
print(“——————————–“)
print(f”{self.title} {self.author} {self.total_copies}“)
print(“================================”)
@classmethod
def print_total(cls):
print(“Total book count is: “,cls.total_books)
# variables – needs to be told if its object level – by default its class level
# methods – needs to be told if its class level – by default its object level
lib1 = Library(“Python Programming”,“Sachin Tendulkar”,10) # __init__ will be called
lib2 = Library(“Machine Learning”,“Virat Kohli”) # __init__ will be called
lib3 = Library(“SQL Programming”, “Dhoni”, 12) # __init__ will be called
print(Library.total_books) #calling using class name
print(lib1.total_books) #calling using object
print(lib2.total_books)
print(Library.total_books)
print(lib1.total_books)
print(lib2.total_books)
print(lib1.total_copies)
print(lib2.total_copies)
print(lib2.author)
print(lib3.author)
lib1.display_detail()
lib1.print_total()
lib3.print_total()
Library.print_total()
def __init__(self,a:int, b=1):
self.num1 = a
self.num2 = b # Protected members are defined as _ (single underscore)
self.__mul = –1 #we are making it as PRIVATE (__)
self.div = –1
self.square = self.num1 ** 2
self._power = self.num1 ** self.num2 #protected
def add(self):
return self.num1 + self.num2
def subtract(self):
return self.num1 – self.num2
def multiply(self):
self.__mul = self.num1 * self.num2
return self.__mul
def divide(self):
self.div = self.num1 / self.num2
m1 = MathsOps(100)
print(m1.add())
print(m1.subtract())
#print(m1.__mul)
print(“Multiply: “,m1.multiply())
division = m1.divide()
print(“Division = “,division)
print(m1._power) #protected concept is there Python but not implemented
print(m1.square)
def __display1(self): #private
print(“Country = India”)
def _display2(self): #protected
print(“Country is India”)
class University (CountryInfo):
def __init__(self, name,year_estb):
self.uname = name
self.year_estb = year_estb
def display(self): #public
print(f”The university {self.uname} was established in the year {self.year_estb}“)
def generateInfo(self):
print(“Sorry this is not implemented”)
def makeitready(self):
print(“Sorry this is not implemented”)
class CityInfo:
def display(self):
print(“City = Hyderabad”)
class Professors(University, CityInfo):
def __init__(self,name,univ,salary, year_estb):
University.__init__(self,univ, year_estb)
self.name = name
self.salary = salary
def printinfo(self):
print(self.name,“: “,self.salary)
University.display(self)
CityInfo.display(self)
def generateInfo(self):
print(“generating payslip”)
class Students(CityInfo,University):
def __init__(self,name,univ,marks, year_estb):
University.__init__(self,univ,year_estb)
self.name = name
self.marks = marks
def printinfo(self):
print(self.name,“:”,self.marks)
University.display(self)
CityInfo.display(self)
def generateInfo(self):
print(“Marks are being generated”)
u1 = University(“ABC University”, 1968)
u2 = University(“XYZ Management University”, 1998)
u2.display()
p1 = Professors(“Sachin”,“ABC University”,167000,1968)
p1.printinfo()
s1 = Students(“Virat”,“XYZ Management University”, 75,1998)
s1.printinfo()
s1.display()
p1.display()
#p1.__display1() #private members are not accessible outside the class
p1._display2() #protected members are accessible by derived classes
ct1 = CityInfo()
ct1.display()
s1.generateInfo() # foreced the derived class to have implemeted the method
p1.generateInfo() # foreced the derived class to have implemeted the method
# Please do the program from:
# https://designrr.page/?id=206786&token=2174026775&type=FP&h=8743
# Page no. 154
#Android: STORY MANTRA:
# categories -> Python -> Python book
def cityinfoprint(self):
print(“City is Hyderabad”)
class University:
def __init__(self,univ):
self.univ = univ
def printInfo(self):
print(“University is “,self.univ)
def sampleM(self,num1,num2):
print(“Sample M 3”)
class Student(University):
def __init__(self,name,age,univ):
#University.__init__(self, univ)
super().__init__(univ)
self.name=name
self.age = age
def printInfo(self):
print(“Name, Age & University :”,self.name, self.age, self.univ)
super().printInfo()
CityInfo.cityinfoprint(self)
def sampleM(self):
”’
:return:
”’
print(“Sample M 1”)
s1 = Student(“Sachin”, 49,“Mumbai University”)
s1.printInfo()
s1.sampleM()
def myfunction1(a=10,b=20):
”’
This is my sample function to do nothing but to show off
:param a:int is for length
:param b:int for for taking input data as breadth
:return: nothing
”’
return a + b
num1 = 78
num2 = 88
print(num1 + num2) # operator overloading
print([2,46] + [5,9,8]) # operator overloading
print(input.__doc__)
print(print.__doc__)
print(len.__doc__)
print(myfunction1.__doc__)
class Shape:
__metaclass__ = ABCMeta
def __init__(self, shapeType):
self.shapeType = shapeType
@abstractmethod
def area(self):
pass
@abstractmethod
def perimeter(self):
pass
class Rectangle(Shape):
def __init__(self, length,breadth):
Shape.__init__(self,“Rectangle”)
self.length = length
self.breadth = breadth
def perimeter(self):
return 2*(self.length + self.breadth)
def area(self):
return self.length * self.breadth
class Circle(Shape):
def __init__(self, radius):
Shape.__init__(self,“Circle”)
self.rad = radius
def perimeter(self):
return 2* 3.14 * self.rad
def area(self):
return 3.14 * self.rad**2
r1 = Rectangle(10,5)
print(“Perimeter is”,r1.perimeter())
print(“Area is”,r1.area())
c1 = Circle(5)
print(“Perimeter is”,c1.perimeter())
print(“Area is”,c1.area())
class Books:
count = 0
def __init__(self,title):
self.title = title
Books.count+=1
@classmethod
def totalcount(cls):
print(“Total titles in the library = “,cls.count)
def __del__(self):
print(f”The object with title {self.title} is getting destroyed. You cant use it again!”)
b1 = Books(“Python Programming”)
b2 = Books(“SQL Programming”)
b3 = Books(“Machine Learning”)
b4 = Books(“Tableau book”)
print(b1.title)
b1.totalcount()
print(b3.title)
b3.totalcount()
b4.totalcount()
print(b4.title)
del b4
input()
b4.totalcount()
def myfun1(a,b):
print(a+b)
return a+b
def myfun2():
print(“I do nothing”)
class Sample:
def __init__(self):
print(“Object created”)
def printinfo(self):
print(“Some output here”)
if __name__ ==“__main__”:
myfun1(99,87)
myfun1(99,7)
s1 = Sample()
=====================
from p11 import myfun1
#RocketScience.myfun2()
print(myfun1(5,10))
import random
random.random()
########### FILE HANDLING
#mode of file handling: r (read), w (write-delete old content and write new), a (Append)
## r+ (read & write), w+ (write & read), a+ (append and read)
filename = “abc.txt”
fileobj = open(filename,“r+”)
content = ”’This is a sample content
story about a king
and a queen
who lived in a jungle
so I am talking about
Lion the kind of jungle”’
fileobj.write(content)
content2 = [‘THis is sample line 1\n‘,‘line 2 content \n‘,‘line 3 content \n‘]
fileobj.writelines(content2)
fileobj.seek(20)
output = fileobj.read()
print(“Content from the file:\n“,output)
fileobj.seek(10)
output = fileobj.read()
fileobj.seek(10)
content3 = fileobj.read(15)
content4 = fileobj.readline()
print(“Content from the file:\n“,output)
fileobj.seek(0)
content5 = fileobj.readlines()
print(“Content from the file:\n“,content5)
fileobj.close()
## Exception handling
#SyntaxError : print(“Hello)
#logical error: you make error in the logic – very difficult to find
#runtime errors (exceptions):
num1 = int(input(“Enter a number: “))
# ValueError exception
a=“k”
b=10
c=-1
try:
c = b/d
except ZeroDivisionError:
print(“Denominator is zero hence stopping the program from executing”)
except TypeError:
print(“Invalid numbers, hence exiting…”)
except NameError:
print(“One of the values has not been defined. Try again”)
except Exception:
print(“Not sure but some error has occurred, we need to stop”)
else:
print(“Answer is”,c)
finally:
print(“We have completed division process”)
#
class InvalidLength(Exception):
def __init__(self,value=0):
self.value = value
length, breadth = –1,-1
while True:
try:
length = int(input(“Enter length: “))
except ValueError:
print(“Invalid number, try again…”)
else:
#assert length > 0, “Rectangle with this diamension is not possible”
if length <=0:
try:
raise InvalidLength
except InvalidLength:
print(“Invalid value for Length hence resetting the value to 1”)
length=1
break
while True:
try:
breadth = int(input(“Enter breadth: “))
except ValueError:
print(“Invalid number, try again…”)
else:
assert breadth>0,“Rectangle with this diamension is not possible”
break
area = length * breadth
print(“Area of the rectangle is”,area)
## ### ##
# datetime, date, time
from datetime import datetime, timedelta
import time
from pytz import timezone
curr_time = datetime.now()
print(“Current time is”,curr_time)
print(“Current time is”,curr_time.strftime(“%d / %m /%Y”))
print(curr_time.year, curr_time.day, curr_time.date())
for i in range(5):
time.sleep(1) # sleep for 2 seconds
print(“Time left:”,5-i,“seconds”)
print(“Good Morning”)
print(“Current time is”,datetime.now())
print(“Date 2 days back was”,(curr_time-timedelta(days=2)).strftime(“%d/%m/%Y”))
print(“UTC Time is”,datetime.now(timezone(‘UTC’)))
print(“UTC Time is”,datetime.now(timezone(‘US/Eastern’)))
print(“UTC Time is”,datetime.now(timezone(‘Asia/Kolkata’)))
Download link:
https://dev.mysql.com/downloads/installer/
Create table employees.Employees( EMPID INT Primary Key auto_increment, FNAME VARCHAR(55) NOT NULL, LNAME VARCHAR(55), DOB DATE, EMAIL VARCHAR(35) unique, PHONE VARCHAR(11), DOJ DATE Default(‘2021-07-20’), — YYYY-MM-DD SALARY FLOAT(2), DEPTID INT, Foreign Key (DEPTID) References Departments(DID), Constraint U_UC_LN_DOB Unique(LNAME,DOB), CHECK(Salary 0.0) ) — Constraints: Primary Key, Foreign Key, Not Null, Unique, Check, Default
— Modifying a table – Table is already created and in use – ALTER TABLE
— ADD or DELETE (DROP) or MODIFY or RENAME a Column
— DDL command to delete is DROP
— DDL command to modify is ALTER
use employees;
ALTER table employees ADD BONUS Float(3);
ALTER table employees ADD dummy Float(3);
ALTER TABLE Employees DROP COLUMN dummy;
ALTER TABLE EMPLOYEES MODIFY COLUMN BONUS float(4)
ALTER TABLE EMPLOYEES RENAME COLUMN BONUS to BONUS_PCT;