PYTHON TRAINING SESSIONS SEP 2023
print( “sdfsadgdsgds” )
# inbuilt functions, followed with ()
# are for comments – you are asking Python to ignore the content after #
print(“5+3”)
print(5+3)
#print(sdfsadgdsgds)
print(“5+3=”,5+3,‘and’,“6*4=”,6*4)
# parameters are the values that we give to a function
# what’s your name?
print(“what’s your name?”)
# He asked, “how are you?”
print(‘He asked, “how are you?”‘)
#He asked, “what’s your name?”
print(”’He asked, “what’s your name?””’) # ”’ or “””
# escape character \
# \\ \t \new \’ …
print(“He asked, \”what’s your name?\”“)
value1 = 5
print(value1)
value1 = 10
print(value1)
sdfsadgdsgds = 100
print(sdfsadgdsgds)
# inbuilt functions, followed with ()
# are for comments – you are asking Python to ignore the content after #
print(“5+3”)
print(5+3)
#print(sdfsadgdsgds)
print(“5+3=”,5+3,‘and’,“6*4=”,6*4)
# parameters are the values that we give to a function
# what’s your name?
print(“what’s your name?”)
# He asked, “how are you?”
print(‘He asked, “how are you?”‘)
#He asked, “what’s your name?”
print(”’He asked, “what’s your name?””’) # ”’ or “””
# escape character \
# \\ \t \new \’ …
print(“He asked, \”what’s your name?\”“)
value1 = 5
print(value1)
value1 = 10
print(value1)
sdfsadgdsgds = 100
print(sdfsadgdsgds)
# variables
a= 5
asdfsaddsg = 10
cost = 25
product1, product2 = 100,120
total_cost = product1 + product2
# variable names should begin with alphabet, and it can contain numbers & _
# invalid names: 1var, num.one
a=35
”’
Multi-line comment
Basic data types:
1. integer (int) – non-decimal numbers both -ve and +ve: -99, -66,0,33,999
2. float (float) – decimal numbers both -ve and +ve: -45.8 , -66.0 , 0.0, 5.78984744
3. complex (complex) – square root of -ve numbers: square root of -1 is i (maths) j in Python
4. Boolean (bool) – True [1] or False [0]
5. String (str) – text content
”’
# type() – type of the data
number_ppl = 55
print(“Data type of number_ppl:”, type(number_ppl))
cost_price = 66.50
print(“type(cost_price): “, type(cost_price))
value1 = 9j
print(“type(value1): “,type(value1))
print(“Square of value1 = “, value1 * value1)
bool_val1 = True # False
print(“type(bool_val1): “,type(bool_val1))
text_val1 = “HELLO”
print(“Type of text_val1 = “,type(text_val1))
# Formatting the output
quantity = 31
cost = 19
total_cost = quantity * cost
# total cost of 51 pens which cost 19 will be total_cost rupees
print(“total cost of”,quantity,“pens which cost”,cost,“will be”,total_cost,“rupees”)
# f-string – formatting the string
print(f”total cost of {quantity} pens which cost {cost} will be {total_cost} rupees”)
quantity = 31; total_cost = 900
cost = total_cost / quantity
print(f”total cost of {quantity} pens which cost {cost:.2f} will be {total_cost} rupees”)
player,country,position = “Virat”,“India”,“Opener”;
print(f”Player {player:<15} plays for {country:^10} at {position:>15} position”);
player,country,position = “Mbwangagya”,“Zimbabwe”,“Wicket-keeper”;
print(f”Player {player:<15} plays for {country:^10} at {position:>15} position”);
a= 5
asdfsaddsg = 10
cost = 25
product1, product2 = 100,120
total_cost = product1 + product2
# variable names should begin with alphabet, and it can contain numbers & _
# invalid names: 1var, num.one
a=35
”’
Multi-line comment
Basic data types:
1. integer (int) – non-decimal numbers both -ve and +ve: -99, -66,0,33,999
2. float (float) – decimal numbers both -ve and +ve: -45.8 , -66.0 , 0.0, 5.78984744
3. complex (complex) – square root of -ve numbers: square root of -1 is i (maths) j in Python
4. Boolean (bool) – True [1] or False [0]
5. String (str) – text content
”’
# type() – type of the data
number_ppl = 55
print(“Data type of number_ppl:”, type(number_ppl))
cost_price = 66.50
print(“type(cost_price): “, type(cost_price))
value1 = 9j
print(“type(value1): “,type(value1))
print(“Square of value1 = “, value1 * value1)
bool_val1 = True # False
print(“type(bool_val1): “,type(bool_val1))
text_val1 = “HELLO”
print(“Type of text_val1 = “,type(text_val1))
# Formatting the output
quantity = 31
cost = 19
total_cost = quantity * cost
# total cost of 51 pens which cost 19 will be total_cost rupees
print(“total cost of”,quantity,“pens which cost”,cost,“will be”,total_cost,“rupees”)
# f-string – formatting the string
print(f”total cost of {quantity} pens which cost {cost} will be {total_cost} rupees”)
quantity = 31; total_cost = 900
cost = total_cost / quantity
print(f”total cost of {quantity} pens which cost {cost:.2f} will be {total_cost} rupees”)
player,country,position = “Virat”,“India”,“Opener”;
print(f”Player {player:<15} plays for {country:^10} at {position:>15} position”);
player,country,position = “Mbwangagya”,“Zimbabwe”,“Wicket-keeper”;
print(f”Player {player:<15} plays for {country:^10} at {position:>15} position”);
Read and Practice the flowchart and Algorithm:
# Operators in Python
# Arithematic Operators: + – * / // (int division), ** (power) % (modulus)
a = 13
b = 10
print(a+b)
print(a-b)
print(a*b)
print(a / b) # 1.3 – output is always float
print(a // b) # only integer – 1
print(a ** b) # 13 to the power of 10
# % – mod – gives you the remainder
print(a % b)
# relational /conditional operators: < > <= >= == !=
# asking question: is it ??? – output is always a bool value (T/F)
print(“Relational: “)
a,b,c = 10,10,13
print(a < b) # is a less than b ? F
print(b > c) # F
print(a<=b) # T
print(b >= c) # F
print(a==b) # is equal to ? – T
print(a!=b) # F
# Logical operators: and or not
# input and output – both are bool
”’
Prediction 1: Rohit and Ishan will open the batting – F
Prediction 2: Rohit or Ishan will open the batting – T
Actual: Rohit and Gill opened the batting
When using AND – Everything condition has to be true to be True
When using OR – Even one condition is true, result will be True
Not taken only 1 input: Not True = False, Not F=T
”’
a,b,c = 10,10,13
print(a < b and b > c or a<=b and b >= c or a==b and a!=b)
# F
## Input() – is used to read values from the user
# int() float() str() complex() bool()
num1 = input(“Enter a number to add: “)
num1 = int(num1) #explicit conversion
print(num1, “and the data type is”,type(num1))
num2 = int(input(“Enter second number: “))
s = num1 + num2 #implicit conversion
print(“Sum of two numbers: “,s)
## A program to convert Celcius to Fahrenheit
c = float(input(“Enter the degree celcius value: “))
f = (9*c/5) + 32
print(“Equivalent temperature in Fahrenheit = “,f)
## Program to find area and perimeter of a rectangle
l = int(input(“Enter length of the rectangle: “))
b = int(input(“Enter breadth of the rectangle: “))
a = l * b
p = 2*(l+b)
print(f”Rectangle with length {l} and breadth {b} will have area as {a} and perimeter as {p}“)
”’
ASSIGNMENT:
1. WAP to convert cm to inches
2. WAP to calculate area and circumference of a circle
3. WAP to calculate average of three given values
4. Input the maximum and minimum temperature recorded in a day and calculate
the average temperature for the day.
5. Convert the distance entered in metres to kilometres.
6. Write a program in C to interchange two integer numbers a and b.
7. WAP to input a,b,c and calculate z as a*b-c
8. WAP to input a two digit number and then interchange the values at 1s and 10s place:
e.g. 65 -> 56 , 83 -> 38
”’
# Arithematic Operators: + – * / // (int division), ** (power) % (modulus)
a = 13
b = 10
print(a+b)
print(a-b)
print(a*b)
print(a / b) # 1.3 – output is always float
print(a // b) # only integer – 1
print(a ** b) # 13 to the power of 10
# % – mod – gives you the remainder
print(a % b)
# relational /conditional operators: < > <= >= == !=
# asking question: is it ??? – output is always a bool value (T/F)
print(“Relational: “)
a,b,c = 10,10,13
print(a < b) # is a less than b ? F
print(b > c) # F
print(a<=b) # T
print(b >= c) # F
print(a==b) # is equal to ? – T
print(a!=b) # F
# Logical operators: and or not
# input and output – both are bool
”’
Prediction 1: Rohit and Ishan will open the batting – F
Prediction 2: Rohit or Ishan will open the batting – T
Actual: Rohit and Gill opened the batting
When using AND – Everything condition has to be true to be True
When using OR – Even one condition is true, result will be True
Not taken only 1 input: Not True = False, Not F=T
”’
a,b,c = 10,10,13
print(a < b and b > c or a<=b and b >= c or a==b and a!=b)
# F
## Input() – is used to read values from the user
# int() float() str() complex() bool()
num1 = input(“Enter a number to add: “)
num1 = int(num1) #explicit conversion
print(num1, “and the data type is”,type(num1))
num2 = int(input(“Enter second number: “))
s = num1 + num2 #implicit conversion
print(“Sum of two numbers: “,s)
## A program to convert Celcius to Fahrenheit
c = float(input(“Enter the degree celcius value: “))
f = (9*c/5) + 32
print(“Equivalent temperature in Fahrenheit = “,f)
## Program to find area and perimeter of a rectangle
l = int(input(“Enter length of the rectangle: “))
b = int(input(“Enter breadth of the rectangle: “))
a = l * b
p = 2*(l+b)
print(f”Rectangle with length {l} and breadth {b} will have area as {a} and perimeter as {p}“)
”’
ASSIGNMENT:
1. WAP to convert cm to inches
2. WAP to calculate area and circumference of a circle
3. WAP to calculate average of three given values
4. Input the maximum and minimum temperature recorded in a day and calculate
the average temperature for the day.
5. Convert the distance entered in metres to kilometres.
6. Write a program in C to interchange two integer numbers a and b.
7. WAP to input a,b,c and calculate z as a*b-c
8. WAP to input a two digit number and then interchange the values at 1s and 10s place:
e.g. 65 -> 56 , 83 -> 38
”’
# Conditions
# passed or failed based on the avg marks
print(“Pass”)
print(“Fail”)
avg = 55
if avg >=50: # if executes only if the condition is True
print(“Pass”)
print(“Congratulations”)
else: # above condition is False then else
print(“Fail”)
print(“Please try again next time”)
print(“Second option”)
”’
avg >= 80: Grade A: IF
avg>=70: Grade B ELIF
avg >=60: Grade C ELIF
avg >=50: Grade D ELIF
avg <50 (else): Grade E : ELSE
avg > 90: print(“Awesome”)
”’
avg =95
if avg>=80:
print(“Grade A”)
print(“Chocolate”)
if avg >= 90:
print(“Awesome”)
elif avg>=70:
print(“Grade B”)
print(“Chocolate”)
elif avg >=60:
print(“Grade C”)
elif avg >=50:
print(“Grade D”)
else:
print(“Grade E”)
avg = 85
# if 70 <= avg
if avg>=70:
if 70 < avg:
print(“Grade A”)
if avg >=90:
print(“Awesome”)
else:
print(“Grade B”)
print(“Chocolate”)
elif avg>=60:
print(“Grade C”)
elif avg >=50:
print(“Grade D”)
else:
print(“Grade E”)
a,b,c = 40,40,40
if a>=b and a>=c:
print(“A is greatest”)
if b>=c and b>=a:
print(“B is greatest”)
if c>=a and c>=b:
print(“C is greatest”)
# passed or failed based on the avg marks
print(“Pass”)
print(“Fail”)
avg = 55
if avg >=50: # if executes only if the condition is True
print(“Pass”)
print(“Congratulations”)
else: # above condition is False then else
print(“Fail”)
print(“Please try again next time”)
print(“Second option”)
”’
avg >= 80: Grade A: IF
avg>=70: Grade B ELIF
avg >=60: Grade C ELIF
avg >=50: Grade D ELIF
avg <50 (else): Grade E : ELSE
avg > 90: print(“Awesome”)
”’
avg =95
if avg>=80:
print(“Grade A”)
print(“Chocolate”)
if avg >= 90:
print(“Awesome”)
elif avg>=70:
print(“Grade B”)
print(“Chocolate”)
elif avg >=60:
print(“Grade C”)
elif avg >=50:
print(“Grade D”)
else:
print(“Grade E”)
avg = 85
# if 70 <= avg
if avg>=70:
if 70 < avg:
print(“Grade A”)
if avg >=90:
print(“Awesome”)
else:
print(“Grade B”)
print(“Chocolate”)
elif avg>=60:
print(“Grade C”)
elif avg >=50:
print(“Grade D”)
else:
print(“Grade E”)
a,b,c = 40,40,40
if a>=b and a>=c:
print(“A is greatest”)
if b>=c and b>=a:
print(“B is greatest”)
if c>=a and c>=b:
print(“C is greatest”)
# WAP to input a number and check if its Odd or even
num = int(input(“Enter a number: “))
if num<0:
print(“Its negative”)
elif num>0:
print(“Its positive”)
else:
print(“Its neither positive nor negative”)
if num %2==0:
print(“Its even number”)
else:
print(“Its odd”)
print(” ============= “)
if num<0:
print(“Its negative”)
else:
if num==0:
print(“Its neither positive nor negative”)
print(“Its even number”)
else:
print(“Its positive”)
if num%2==0:
print(“Its even number”)
else:
print(“Its odd”)
”’
Loops: Repeating a certain block of code multiple times
1. You know how many times to repeat : FOR loop
2. When you have a condition to repeat: WHILE loop
range(=start, <stop, =step)
range(5,17,4): 5, 9, 13
range(=start, <stop) – default step is 1
range(4,9) : 4, 5,6,7,8
range(<stop) : start = 0, step = 1
range(5): 0, 1,2, 3, 4
”’
for i in range(5,17,4):
print(“HELLO”)
print(“Value of i:”,i)
for i in range(4,9):
print(“HELLO AGAIN!”)
print(“Value of i:”,i)
for i in range(5):
print(“How are you?”)
print(“Value of i:”,i)
## While: should have condition and loop repeats only when the condition is true
cont = “yes”
i=0
while cont==“yes”:
print(“HELLO : “,i)
i+=5 # i = i + 5
if i>50:
cont = “No”
num = int(input(“Enter a number: “))
if num<0:
print(“Its negative”)
elif num>0:
print(“Its positive”)
else:
print(“Its neither positive nor negative”)
if num %2==0:
print(“Its even number”)
else:
print(“Its odd”)
print(” ============= “)
if num<0:
print(“Its negative”)
else:
if num==0:
print(“Its neither positive nor negative”)
print(“Its even number”)
else:
print(“Its positive”)
if num%2==0:
print(“Its even number”)
else:
print(“Its odd”)
”’
Loops: Repeating a certain block of code multiple times
1. You know how many times to repeat : FOR loop
2. When you have a condition to repeat: WHILE loop
range(=start, <stop, =step)
range(5,17,4): 5, 9, 13
range(=start, <stop) – default step is 1
range(4,9) : 4, 5,6,7,8
range(<stop) : start = 0, step = 1
range(5): 0, 1,2, 3, 4
”’
for i in range(5,17,4):
print(“HELLO”)
print(“Value of i:”,i)
for i in range(4,9):
print(“HELLO AGAIN!”)
print(“Value of i:”,i)
for i in range(5):
print(“How are you?”)
print(“Value of i:”,i)
## While: should have condition and loop repeats only when the condition is true
cont = “yes”
i=0
while cont==“yes”:
print(“HELLO : “,i)
i+=5 # i = i + 5
if i>50:
cont = “No”
for i in range(1,11):
print(i,end=” : “)
print()
a,b,c,d,e = 50,60,50,60,70
total = a + b+c+d+e
total2 =0
for i in range(5):
val = int(input(“Enter the number: “))
total2 +=val # total2 = total2 + val
print(“Total sum of all the values from the loop = “,total2)
# * * * * *
for i in range(5):
print(“*”,end=” “)
print()
”’
* * * * *
* * * * *
* * * * *
* * * * *
* * * * *
”’
for j in range(5):
for i in range(5):
print(“*”, end=” “)
print()
”’
*
* *
* * *
* * * *
* * * * *
”’
for j in range(5):
for i in range(j+1):
print(“*”, end=” “)
print()
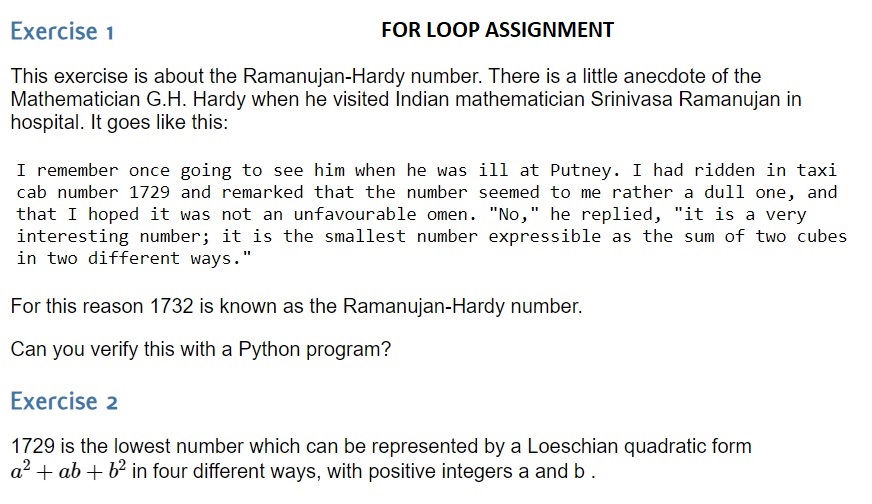
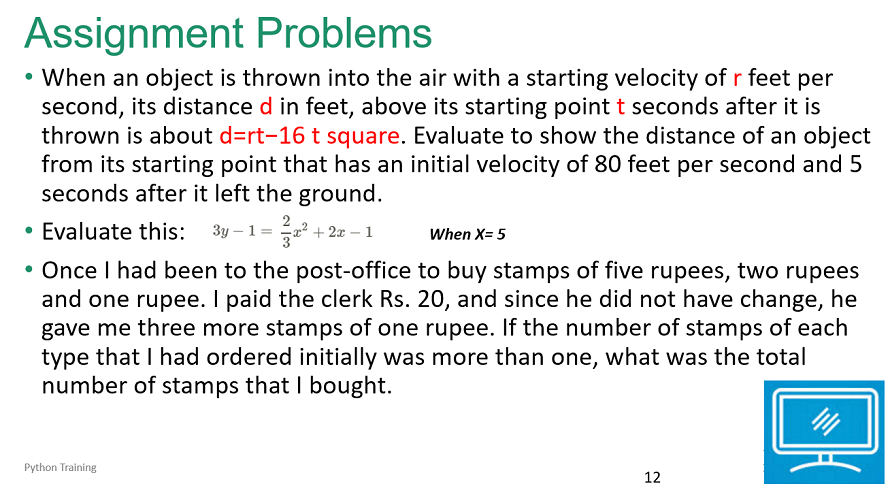
## For loop
”’
*
* *
* * *
* * * *
* * * * *
”’
for i in range(5):
for j in range(i+1):
print(“*”,end=” “)
print()
”’
* * * * *
* * * *
* * *
* *
*
”’
for i in range(5):
for j in range(5-i):
print(“*”,end=” “)
print()
”’
* * * * *
* * * *
* * *
* *
*
”’
for i in range(5):
for j in range(i):
print(” “,end=“”)
for j in range(5-i):
print(“*”,end=” “)
print()
”’
Assignment:
*
* *
* * *
* * * *
* * * * *
”’
”’
Option 1: implementing While using a condition
”’
cont =“Y”
while cont==“Y”:
num1 = int(input(“Enter a number: “))
num2 = int(input(“Enter a number: “))
print(“Choose your option:”)
print(“1. Addition”)
print(“2. Subtraction”)
print(“3. Multiplication”)
print(“4. Division”)
print(“99. Exit”)
ch=input(“Enter your choice of operation:”)
if ch==“1”:
print(“Addition = “,num1 + num2)
elif ch==“2”:
print(“Subtraction = “, num1 – num2)
elif ch==“3”:
print(“Multiplication = “, num1 * num2)
elif ch==“4”:
print(“Division = “, num1 / num2)
elif ch==“99”:
#pass
cont=“N”
else:
print(“Invalid Input”)
print(“option1: going for one more iteration…”)
”’
Option 2: implementing While using True
”’
print(“Running option 2:”)
while True:
num1 = int(input(“Enter a number: “))
num2 = int(input(“Enter a number: “))
print(“Choose your option:”)
print(“1. Addition”)
print(“2. Subtraction”)
print(“3. Multiplication”)
print(“4. Division”)
print(“99. Exit”)
ch=input(“Enter your choice of operation:”)
if ch==“1”:
print(“Addition = “,num1 + num2)
elif ch==“2”:
print(“Subtraction = “, num1 – num2)
elif ch==“3”:
print(“Multiplication = “, num1 * num2)
elif ch==“4”:
print(“Division = “, num1 / num2)
elif ch==“99”:
break # it breaks the loop
else:
print(“Invalid Input”)
print(“option2: going for one more iteration…”)
”’
Assignments:
1. Generate Prime numbers between given values
2. WAP to calculate average and grade for the given number of students
3. Calculate area for given choice of shape till user wants
4. Generate fibonacci series numbers till user says so
”’
”’
*
* *
* * *
* * * *
* * * * *
”’
for i in range(5):
for j in range(i+1):
print(“*”,end=” “)
print()
”’
* * * * *
* * * *
* * *
* *
*
”’
for i in range(5):
for j in range(5-i):
print(“*”,end=” “)
print()
”’
* * * * *
* * * *
* * *
* *
*
”’
for i in range(5):
for j in range(i):
print(” “,end=“”)
for j in range(5-i):
print(“*”,end=” “)
print()
”’
Assignment:
*
* *
* * *
* * * *
* * * * *
”’
”’
Option 1: implementing While using a condition
”’
cont =“Y”
while cont==“Y”:
num1 = int(input(“Enter a number: “))
num2 = int(input(“Enter a number: “))
print(“Choose your option:”)
print(“1. Addition”)
print(“2. Subtraction”)
print(“3. Multiplication”)
print(“4. Division”)
print(“99. Exit”)
ch=input(“Enter your choice of operation:”)
if ch==“1”:
print(“Addition = “,num1 + num2)
elif ch==“2”:
print(“Subtraction = “, num1 – num2)
elif ch==“3”:
print(“Multiplication = “, num1 * num2)
elif ch==“4”:
print(“Division = “, num1 / num2)
elif ch==“99”:
#pass
cont=“N”
else:
print(“Invalid Input”)
print(“option1: going for one more iteration…”)
”’
Option 2: implementing While using True
”’
print(“Running option 2:”)
while True:
num1 = int(input(“Enter a number: “))
num2 = int(input(“Enter a number: “))
print(“Choose your option:”)
print(“1. Addition”)
print(“2. Subtraction”)
print(“3. Multiplication”)
print(“4. Division”)
print(“99. Exit”)
ch=input(“Enter your choice of operation:”)
if ch==“1”:
print(“Addition = “,num1 + num2)
elif ch==“2”:
print(“Subtraction = “, num1 – num2)
elif ch==“3”:
print(“Multiplication = “, num1 * num2)
elif ch==“4”:
print(“Division = “, num1 / num2)
elif ch==“99”:
break # it breaks the loop
else:
print(“Invalid Input”)
print(“option2: going for one more iteration…”)
”’
Assignments:
1. Generate Prime numbers between given values
2. WAP to calculate average and grade for the given number of students
3. Calculate area for given choice of shape till user wants
4. Generate fibonacci series numbers till user says so
”’
Below programs to be done later
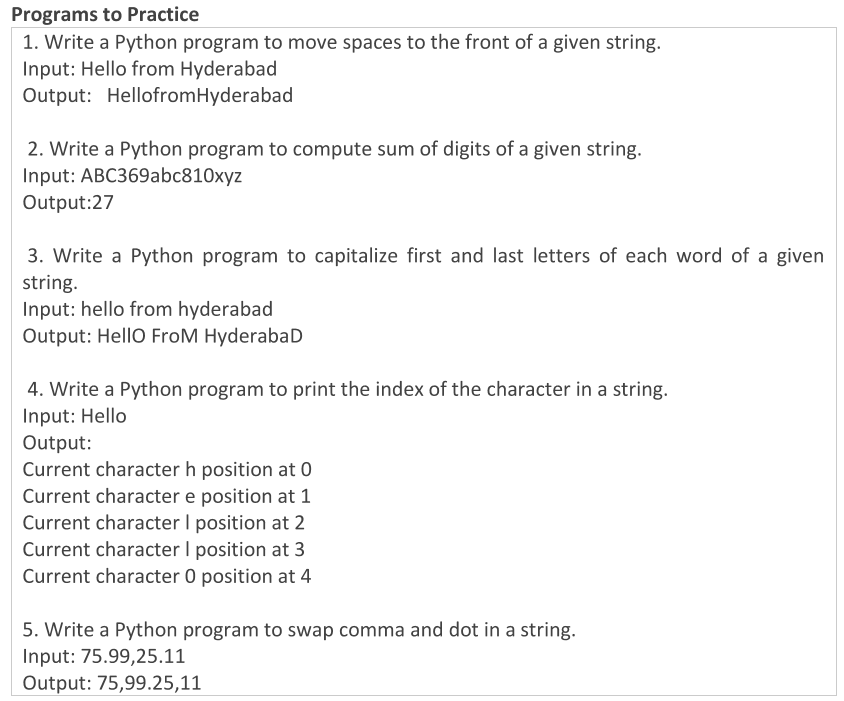
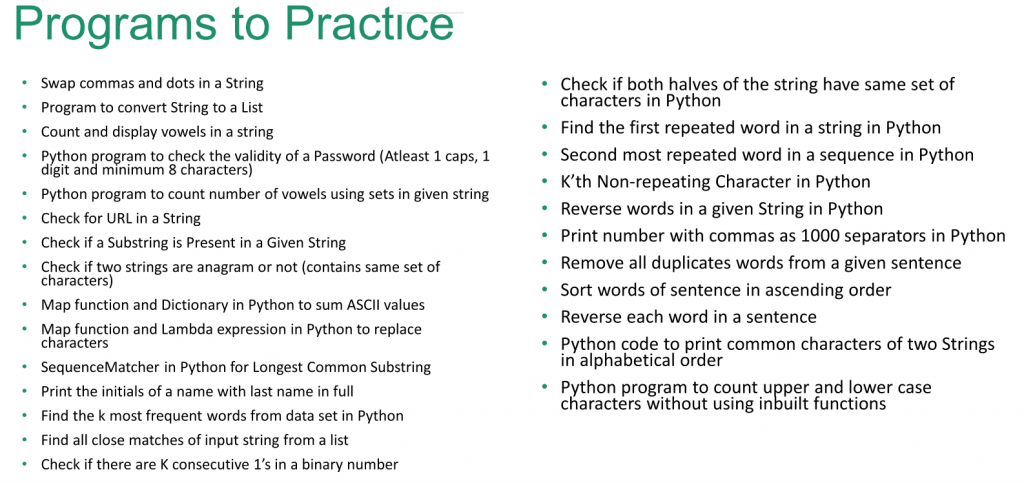
num= 5
if num<0:
print(“Positive”)
elif num>0:
pass
else:
pass
import random
number = random.randint(1,100)
count = 0
while True:
guess=int(input(“Guess the number (1-100):”))
count+=1
if guess <1 or guess>100:
print(“Invalid guess, try again”)
continue
elif number==guess:
print(f”You have correctly guessed the number in {count} attempts”)
break
elif number < guess:
print(“You have guessed a higher number. Try again…”)
else:
print(“You have guessed a lower number. Try again…”)
print(“Hello”)
print(“How are you?”)
print(“Hello”, end=“. “)
print(“How are you?”)
###############
# String
var1 = “HELLO”
var2 = ‘how are you there?’
# triple quotes can have multiline of text
var3 = ”’I am fine”’
var4 = “””I am here”””
print(var1,var2,var3,var4)
print(type(var1),type(var2),type(var3),type(var4))
var3 = ”’I am fine
I am doing well
I am alright”’
var4 = “””I am here
I am there
I am everywhere”””
print(var3)
print(var4)
# len() – gives you number of characters in a string
print(len(“hello”))
v1 = “hello”
print(len(v1))
if num<0:
print(“Positive”)
elif num>0:
pass
else:
pass
import random
number = random.randint(1,100)
count = 0
while True:
guess=int(input(“Guess the number (1-100):”))
count+=1
if guess <1 or guess>100:
print(“Invalid guess, try again”)
continue
elif number==guess:
print(f”You have correctly guessed the number in {count} attempts”)
break
elif number < guess:
print(“You have guessed a higher number. Try again…”)
else:
print(“You have guessed a lower number. Try again…”)
print(“Hello”)
print(“How are you?”)
print(“Hello”, end=“. “)
print(“How are you?”)
###############
# String
var1 = “HELLO”
var2 = ‘how are you there?’
# triple quotes can have multiline of text
var3 = ”’I am fine”’
var4 = “””I am here”””
print(var1,var2,var3,var4)
print(type(var1),type(var2),type(var3),type(var4))
var3 = ”’I am fine
I am doing well
I am alright”’
var4 = “””I am here
I am there
I am everywhere”””
print(var3)
print(var4)
# len() – gives you number of characters in a string
print(len(“hello”))
v1 = “hello”
print(len(v1))
#string
var1 = “HELLO”
var2 = “THERE”
# operations
print(var1 + var2)
print((var1+” “) * 5)
for i in var1:
print(i)
print(” indexing – [] , position starts from 0″)
var1 = “HELLO”
print(var1[0])
print(var1[4])
#backward index – starts from minus 1
print(var1[-1], var1[-5])
print(var1[len(var1)-1], var1[-1])
# strings are immutable – you cant edit/ you can replace
var1 = “hELLO there”
print(var1)
#var1[0] = ‘H’ TypeError: ‘str’ object does not support item assignment
# : to read continuous set of values
# need 2 to 4 element
print(var1[1:4])
print(“First 3 elements: “, var1[0:3],var1[:3], var1[-5:-2], var1[-len(var1):-len(var1)+3])
print(“Last 3 elements: “,var1[-3:])
print(“Content: “,var1, var1[:])
var1 = “HELLO”
var2 = “THERE”
# operations
print(var1 + var2)
print((var1+” “) * 5)
for i in var1:
print(i)
print(” indexing – [] , position starts from 0″)
var1 = “HELLO”
print(var1[0])
print(var1[4])
#backward index – starts from minus 1
print(var1[-1], var1[-5])
print(var1[len(var1)-1], var1[-1])
# strings are immutable – you cant edit/ you can replace
var1 = “hELLO there”
print(var1)
#var1[0] = ‘H’ TypeError: ‘str’ object does not support item assignment
# : to read continuous set of values
# need 2 to 4 element
print(var1[1:4])
print(“First 3 elements: “, var1[0:3],var1[:3], var1[-5:-2], var1[-len(var1):-len(var1)+3])
print(“Last 3 elements: “,var1[-3:])
print(“Content: “,var1, var1[:])
str1 =“Hello”
print(str1.isalpha())
print(str1.isdigit())
print(str1.isalnum())
print(str1.istitle())
val1 = input(“Enter a number: “)
if val1.isdigit():
val1 = int(val1)
else:
print(“You have not entered a valid number”)
#
val1 = “heLLo”
print(“lowe:”,val1.lower())
print(“upper: “,val1.upper())
# Indian citizen and over 18 yrs of age
check =0
age = int(input(“Enter your age: “))
citizen = input(“Enter your country of citizenship:”)
citizen = citizen.upper()
if age>=18 and citizen == “INDIA”:
print(“You are eligible to vote in India”)
else:
print(“You are not eligible to vote in India”)
#
str1 = “How are you?”
print(str1.isalpha())
print(“Count: “,str1.count(“o”))
if str1.count(“o”,3,10)>0:
print(“Index: “,str1.index(“o”,3,10))
else:
print(“Value o not in the given range”)
print(“FIND: “,str1.find(“o”,3,10))
print(“FIND: “,str1.find(“o”,3,7)) # -1 means value not found
str2 = ” h e l l o “
print(“Len 1: “,len(str2))
str2 = str2.strip()
print(str2)
print(“Len 2: “,len(str2))
str3 = “I am fine how are you are you fine or more than fine what?”
print(str3.split()) #output of split is a list
print(str3.split(“a”))
val2 = str3.split()
print(“Data type of val2:”,type(val2))
print(“Join = “,“…”.join(val2))
val4 = str3.replace(“fine”,“FINE”,2)
print(“Replace:”,val4)
print(str1.isalpha())
print(str1.isdigit())
print(str1.isalnum())
print(str1.istitle())
val1 = input(“Enter a number: “)
if val1.isdigit():
val1 = int(val1)
else:
print(“You have not entered a valid number”)
#
val1 = “heLLo”
print(“lowe:”,val1.lower())
print(“upper: “,val1.upper())
# Indian citizen and over 18 yrs of age
check =0
age = int(input(“Enter your age: “))
citizen = input(“Enter your country of citizenship:”)
citizen = citizen.upper()
if age>=18 and citizen == “INDIA”:
print(“You are eligible to vote in India”)
else:
print(“You are not eligible to vote in India”)
#
str1 = “How are you?”
print(str1.isalpha())
print(“Count: “,str1.count(“o”))
if str1.count(“o”,3,10)>0:
print(“Index: “,str1.index(“o”,3,10))
else:
print(“Value o not in the given range”)
print(“FIND: “,str1.find(“o”,3,10))
print(“FIND: “,str1.find(“o”,3,7)) # -1 means value not found
str2 = ” h e l l o “
print(“Len 1: “,len(str2))
str2 = str2.strip()
print(str2)
print(“Len 2: “,len(str2))
str3 = “I am fine how are you are you fine or more than fine what?”
print(str3.split()) #output of split is a list
print(str3.split(“a”))
val2 = str3.split()
print(“Data type of val2:”,type(val2))
print(“Join = “,“…”.join(val2))
val4 = str3.replace(“fine”,“FINE”,2)
print(“Replace:”,val4)
# LIST – mutable linear ordered collection
l1 = []
print(type(l1))
l1 = [21,“Hello”, True,5.4]
print(l1[-1])
print(l1[1:3])
print(“Size of the list = “,len(l1))
l1[3] = [5.4, 6.9,8.0]
print(l1[:])
print(“Iterating over a list:”)
for i in l1:
print(i)
print(“Iterating over a list: done”)
a= l1[-1]
print(a[1])
print(“Type of 1st element: “, type(l1[0]))
## properties related to lists
l2 = [4,5,6]
l3=[“a”,“b”,“c”]
l4 = l2 + l3
print(l4)
l5 = l4 *3
print(l5)
#list methods
l2 = [4,5,6,5,6,6]
print(“Count of 5: “,l2.count(5))
search_val = 6
if l2.count(search_val) >0:
print(“Index: “,l2.index(search_val,3,5))
else:
print(“Something is wrong”)
# add elements to existing list
l2 = [4,5,6]
# append() : to add at the end of the list, and
# insert() : to add at any given position
l2.append(10)
l2.append(20)
print(l2)
l2.insert(1,8)
l2.insert(1,3)
print(l2)
”’
WAP to input marks of 5 students in 5 subjects and save them as list of marks
e.g. [[. . . . .],[. . . . .],[. . . . .],[. . . . .],[. . . . .]]
”’
# to delete elements from list, we use pop(index) and remove(value)
l2.remove(20)
print(“remove 20 from the list: “,l2)
l2.pop(3) #4th element in the list is deleted
print(“removing 4th element from the list: “,l2)
#reverse()
l2.reverse()
print(“L2 after reverse: “,l2)
#sort()
l2.sort() #default is increasing order
print(“L2 after sort: “,l2)
l2.sort(reverse=True) #sorting in reverse order- decreasing order
print(“List after reverse sorting:”,l2)
# extend() : l2 = l2 + l3
l3 = [10,30,20]
l2.extend(l3)
print(“L2 after extend: “,l2)
#copy() and =
l5 = l2 # they are pointing to each other
l6 = l2.copy() # creates a duplicate copy
print(“1. L2 = “,l2)
print(“1. L5 = “,l5)
print(“1. L6 = “,l6)
l2.append(77)
l5.append(88)
l6.append(99)
print(“2. L2 = “,l2)
print(“2. L5 = “,l5)
print(“2. L6 = “,l6)
#clear to remove all the value
l2.clear()
print(“L2 afer clear: “,l2)
del l2
#print(“After delete: “,l2) # NameError: name ‘l2’ is not defined.
# read marks of 3 students for 3 subjects
# [[],[],[]]
num_students = 3
num_subjects = 3
subjects = [‘Maths’,‘Physics’,‘Chemistry’]
students = [‘Rohit’,‘Surya’,‘Virat’]
master = []
for j in range(num_students):
print(“Accepting marks for the student “,students[j])
marks = []
for i in range(num_subjects):
m = int(input(“\tEnter the marks in “+subjects[i]+“: “))
marks.append(m)
master.append(marks)
print(“Marks = “,master)
print(“Marks for each student:”)
for i in range(num_students):
print(master[i])
”’
Extend the above program to find overall topper and each subject topper with marks
[[45, 56, 89], [98, 56, 45], [90, 87, 65]]
”’
# [[],[],[]]
num_students = 3
num_subjects = 3
subjects = [‘Maths’,‘Physics’,‘Chemistry’]
students = [‘Rohit’,‘Surya’,‘Virat’]
master = []
for j in range(num_students):
print(“Accepting marks for the student “,students[j])
marks = []
for i in range(num_subjects):
m = int(input(“\tEnter the marks in “+subjects[i]+“: “))
marks.append(m)
master.append(marks)
print(“Marks = “,master)
print(“Marks for each student:”)
for i in range(num_students):
print(master[i])
”’
Extend the above program to find overall topper and each subject topper with marks
[[45, 56, 89], [98, 56, 45], [90, 87, 65]]
”’
# Tuple: linear ordered immutable collection
l1 = [45,55,65]
l1[0] = 35
print(“L1: “,l1)
t1 = (45,55,65)
print(“1. type of t1:”,type(t1))
#t1[0] = 35 #’tuple’ object does not support item assignment
t1 = list(t1) # you convert tuple data to list
print(“2. type of t1:”,type(t1))
t1[0] = 35
t1 = tuple(t1)
print(“3. type of t1:”,type(t1))
print(“T1 = “,t1)
print(t1.count(35))
print(t1.index(35))
t2 = ()
print(“A. type = “,type(t2))
t2 = (2,)
print(“B. type = “,type(t2))
t2 = (2,6)
print(“B. type = “,type(t2))
t1 = (45,55,65) #packing
print(“Len = “,len(t1))
a,b,c = t1 #unpacking
print(a,b,c)
#compare the values
t3 = (4,5)
t4 = (4,1,99)
# dzat ear
print(“Last element: “,t4[-1])
for i in t4:
print(i)
print(” Dictionary – unordered mutable collection – with key:value pair”)
d1 = {}
print(“D1 type = “,type(d1))
d1 = {5:“Hello”, “Hello”:“Sachin Tendulkar”,“Runs”:25000, 5:“Mumbai”}
print(d1[5])
print(d1[“Hello”])
#keys have to be unique
# keys, values, items
print(“Keys: “,d1.keys())
print(“Values: “,d1.values())
print(“Items: “,d1.items())
for k,v in d1.items():
print(“K:V =”,k,“:”,v)
for k in d1.keys():
print(“K =”,k)
for v in d1.values():
print(“V =”,v)
d2 = {3:“Three”,6:“Six”,9:“Nine”}
d3 = {1:“Three”,2:“Six”,4:“Nine”}
d2.update(d3) # merge two dictionaries
print(d2)
d2.update(d2)
print(d2)
# Dictionary
dict1 = {}
temp_dict ={1:“One”,2:“Two”}
dict1.update(temp_dict) #dict1 = dict1 + temp
print(dict1)
”’
Assignment:
Read marks of 5 subjects for 5 students and save the data along with student’s names
”’
print(dict1[1])
dict1[1]=“Eleven”
print(dict1)
dict2 = dict1
dict3 = dict1.copy()
print(“1. Dict 1 = “,dict1)
print(“2. Dict 2 = “,dict2)
print(“3. Dict 3 = “,dict3)
dict1.update({3:“Three”})
dict3.popitem() # removes the value
print(“1. Dict 1 = “,dict1)
print(“2. Dict 2 = “,dict2)
print(“3. Dict 3 = “,dict3)
dict2.pop(2)
print(“1. Dict 1 = “,dict1)
dict2.clear()
print(dict1)
### SETS
set1 = {}
print(“Type of set1 = “,type(set1))
set1 = {5}
print(“Type of set1 = “,type(set1))
set2 = {2,4,6}
print(“Number of even numbers = “,len(set2))
print(set2)
# unordered linear mutable collection
# convert: set to list, list to set, set to tuple, tuple to set, list to tuple
list1 = [10,10,10,20,20,30]
list1 = list(set(list1))
print(list1)
set2.update({1,4,16})
print(“After update: “,set2)
set2.add(50)
print(“After add: “,set2)
# = and copy() works exactly like list and tuple
# union, intersection, minus, subset, subset
a = {1,3,5,7,8}
b = {2,4,6,8,7}
print(a.isdisjoint(b))
print(“# union”)
print(a.union(b))
print(a|b)
print(“# intersection”)
print(a.intersection(b))
print(a & b)
print(“#difference”)
print(a.difference(b))
print(a-b)
print(b.difference(a))
print(b-a)
print(“#symmetric difference”)
print(a ^b)
print(a.symmetric_difference(b))
# each of the above methods returns a new set
print(a)
print(b)
# if you dont want to create a new and update the first set instead:
# first_set…..update()
# first_set = first_set + ()
a.difference_update(b)
print(a)
dict1 = {}
temp_dict ={1:“One”,2:“Two”}
dict1.update(temp_dict) #dict1 = dict1 + temp
print(dict1)
”’
Assignment:
Read marks of 5 subjects for 5 students and save the data along with student’s names
”’
print(dict1[1])
dict1[1]=“Eleven”
print(dict1)
dict2 = dict1
dict3 = dict1.copy()
print(“1. Dict 1 = “,dict1)
print(“2. Dict 2 = “,dict2)
print(“3. Dict 3 = “,dict3)
dict1.update({3:“Three”})
dict3.popitem() # removes the value
print(“1. Dict 1 = “,dict1)
print(“2. Dict 2 = “,dict2)
print(“3. Dict 3 = “,dict3)
dict2.pop(2)
print(“1. Dict 1 = “,dict1)
dict2.clear()
print(dict1)
### SETS
set1 = {}
print(“Type of set1 = “,type(set1))
set1 = {5}
print(“Type of set1 = “,type(set1))
set2 = {2,4,6}
print(“Number of even numbers = “,len(set2))
print(set2)
# unordered linear mutable collection
# convert: set to list, list to set, set to tuple, tuple to set, list to tuple
list1 = [10,10,10,20,20,30]
list1 = list(set(list1))
print(list1)
set2.update({1,4,16})
print(“After update: “,set2)
set2.add(50)
print(“After add: “,set2)
# = and copy() works exactly like list and tuple
# union, intersection, minus, subset, subset
a = {1,3,5,7,8}
b = {2,4,6,8,7}
print(a.isdisjoint(b))
print(“# union”)
print(a.union(b))
print(a|b)
print(“# intersection”)
print(a.intersection(b))
print(a & b)
print(“#difference”)
print(a.difference(b))
print(a-b)
print(b.difference(a))
print(b-a)
print(“#symmetric difference”)
print(a ^b)
print(a.symmetric_difference(b))
# each of the above methods returns a new set
print(a)
print(b)
# if you dont want to create a new and update the first set instead:
# first_set…..update()
# first_set = first_set + ()
a.difference_update(b)
print(a)
# Functions
”’
Inbuilt functions: print() type() input()…
User defined functions: we are going to define
oneline function: lambda
function definition
function calling
”’
# defining the function – giving three statements a name
def sometext():
print(“WHo are you?”)
print(“What are you doing?”)
print(“Where are you going?”)
def sometext1():
print(“WHo are you?”)
print(“What are you doing?”)
print(“Where are you going?”)
return 100
def sometext2(name):
print(“WHo are you , Mr”,name)
print(“What are you doing, Mr”,name)
print(“Where are you going, Mr”,name)
def addition(a,b):
c = a + b
return c
sometext()
print(“doing something else”)
sometext()
print(“doing something else”)
sometext()
print(“Printing sometext(): “,sometext())
print(“Printing sometext1(): “,sometext1())
list1 = []
print(list1.append(5))
sometext2(“Sachin”)
print(“Addition of 5 and 10 is:”,addition(5,10))
”’
Inbuilt functions: print() type() input()…
User defined functions: we are going to define
oneline function: lambda
function definition
function calling
”’
# defining the function – giving three statements a name
def sometext():
print(“WHo are you?”)
print(“What are you doing?”)
print(“Where are you going?”)
def sometext1():
print(“WHo are you?”)
print(“What are you doing?”)
print(“Where are you going?”)
return 100
def sometext2(name):
print(“WHo are you , Mr”,name)
print(“What are you doing, Mr”,name)
print(“Where are you going, Mr”,name)
def addition(a,b):
c = a + b
return c
sometext()
print(“doing something else”)
sometext()
print(“doing something else”)
sometext()
print(“Printing sometext(): “,sometext())
print(“Printing sometext1(): “,sometext1())
list1 = []
print(list1.append(5))
sometext2(“Sachin”)
print(“Addition of 5 and 10 is:”,addition(5,10))