Data Analytics Nov 2022- Part 2
DAY 21
select * from HR.Employees;
— explicit conversion
select Last_name, Hire_date, to_char(hire_date, ‘MONTH DD, YYYY’), Salary, to_char(salary,’$999999.9′) from HR.Employees;
select ‘12345.66’, to_number(‘12345.66′,’9999999’) from Dual
select ‘December 11, 2022, 6:00 AM’, To_date(‘December 11, 2022, 6:00 AM’, ‘Month dd, YYYY, HH:MI AM’) from Dual
— Multiple conditions
Select Decode(‘BBC’,’ABC’,’Text Matched to ABC’,DECODE(‘CBC’,’BBC’,’Text Matched to BBC’,’Something else’)) from Dual
Select Decode(Hire_date, ’17-JUN-03′,’FIRST DAT AT WORK’,’OLD TIMER’) from hr.employees
Select last_name, salary, case When Salary <6000 then ‘Grade 1’
When Salary >=6000 and Salary <12000 then ‘Grade 2’
else ‘Grade 3’
End Case
from hr.employees;
— Aggregate Functions – which will aggregate and give single result
select count(*) from hr.employees
select avg(Salary) from hr.employees
select sum(Salary) from hr.employees
select count(*) ‘Total Employees’ , avg(Salary) ‘Average Salary’, sum(Salary) ‘Total Salary’, Min(Hire_date) ‘Oldest Employee’, Max(Hire_date) ‘Newest Employee’ from hr.employees
select count(*) Total , avg(Salary) Average, sum(Salary) “Total Salary”, Min(Hire_date) Oldest , Max(Hire_date) from hr.employees
select DEPARTMENT_ID,JOB_ID, avg(salary) from hr.employees group by Department_ID, JOB_ID HAVING avg(salary) >10000
— JOIN
select convert(int, 12.99) from dual
select cast(12.99 as int) from dual
select * from hr.employees
select * from hr.departments
select * from hr.locations
— Inner join (default)
select department_name, first_name Manager from hr.departments d join hr.employees e on (e.EMPLOYEE_ID = d.manager_id)
select department_name, first_name from hr.departments d , hr.employees e
select department_name, first_name, last_name from hr.departments d join hr.employees e on (e.department_ID = d.department_ID)
select department_name, first_name, last_name from hr.departments d join hr.employees e Using (department_ID) order by DEPARTMENT_NAME DESC
— LEFT /RIGHT OUTER JOIN based on + sign
select department_name, first_name from hr.departments d , hr.employees e where e.department_ID = d.department_ID(+)
— Full outer Join
select department_name DEPARTMENT, x.department_ID, first_name, last_name from hr.departments x full outer join hr.employees e on (e.department_ID = x.department_ID)
Select First_name, department_name, city from hr.departments d, hr.employees e, hr.locations l where d.department_ID = e.department_ID and d.location_ID=l.location_ID
— Lex De Haan
select first_name from hr.employees where employee_ID = (select employee_ID from hr.employees where first_name=’Lex’ and last_name=’De Haan’)
select first_name from hr.employees where employee_ID in (select employee_ID from hr.employees where department_ID = 60)
select first_name from hr.employees where employee_ID in (select employee_ID from hr.employees where department_ID = 60)
UNION
select first_name from hr.employees where employee_ID = (select employee_ID from hr.employees where first_name=’Lex’ and last_name=’De Haan’)
STARTING PYTHON PROGRAMMING
Learn to install different software… follow below links to install software:
Step 1: Video: Click here to download and install Python
DAY 24 : DAY 2 OF PYTHON TUTORIAL
print('Hello')
print(3+4+5)
print('3+4+5=',3+4+5)
# passing any value to functions - arguments
name1 = 'Laxman'
print("name1: ",name1)
print("name1: ",name1)
print("name1: ",name1)
print("name1: ",name1)
print("name1: ",name1)
#variable names: can not start with a number: name1 is valid but 1name is not a valid variable name
# _ is the only special character that is allowed in the variable name
# variable name accepts - alphabets(a-z A-Z) 0-9 and _
n1ame = "Hello"
length = 51
breadth = 23
area = length * breadth
perimeter = 2 * (length + breadth)
#A rectangle with length x and breadth y has area of a and perimeter of p
print('A rectangle with length',length,"and breadth",breadth,"has area of",area,"and perimeter of",perimeter)
# format string - f string
print(f"A rectangle with length {length} and breadth {breadth} has area of {area} and perimeter of {perimeter}")
name1 = 'Laxman' # str - string
print(type(name1))
length = 51 # int - integer: -999, -88,0,9,100
print(type(length))
avg = 55.63 #float - 5.1, -6.9, 0.0, 5.0
print(type(avg))
isRight = True # False : bool - boolean True/False
print(type(isRight))
num_val = 6j # j is the imaginary value (complex) - square root of -1
print(type(num_val)) #complex
length = 53
print(length)
length = True
print(length)
length = "Sachin"
print(length)
length = "54"
breadth = "43"
print(length + breadth)
#<class 'str'> str()
#<class 'int'> int()
#<class 'float'> float()
#<class 'bool'> bool()
#<class 'complex'> complex()
val1 = 500
print("Val1 is ",type(val1))
val1 = str(val1) # val1 = "500"
print("Val1 is ",type(val1))
val2 = "55A"
val2 = int(val2)
#arithmetic operations: + - * / // ** %
val1 = 20
val2 = 8
print(val1 + val2)
print(val1 - val2)
print(val1 * val2)
print(val1 / val2)
print(val1 // val2) # // just the integer
print(val1 ** val2) #power(val1, val2)
print(val1 % val2) #
#comparison operators: Output is always boolean - True / False
val1 = 20
val2 = 8
val3 = 20 # 20 should be assigned to val3
# = assignment operator -assigning the value
print(val1 == val2) # is val1 equal to val2 ?
print(val1 != val3) # is val1 not equal to val3 ?
print(val1 > val2) # T
print(val1 >= val3) # is Val1 greater or equal to val3
print(val1 > val3)
print(val1 < val2)
print(val1 <= val2)
print(val1 < val3)
#logical operator" input is boolean and the output is also a boolean
# prediction: Sachin and Sehwag will open the batting
# actual - Sachin and Laxman opened the batting
print(True and True)
print(False and True)
print(True and False)
print(False and False)
val1,val2,val3 = 20, 8, 20
print(val2 == val3 and val1 <=val2 and val3 >=val1 and val2==val3)
# F
# or - even if one value is TRUE entire result will be True
print(True or True)
print(False or True)
print(True or False)
print(False or False)
print(val2 == val3 or val1 <=val2 or val3 >=val1 or val2==val3)
# T
print(not True)
print(not False)
print(not val2 == val3 or val1 <=val2 or val3 >=val1 or val2==val3)
print(val2 == val3 or val1 <=val2 and val3 >=val1 or val2==val3)
#F
# 2+2*3 =
# ax sq + bx + c = 0, find x when a,b and c are given
# D = bsq - 4ac
# solution 1: (-b - sq root D) /2a
# solution 2: (-b + sq root D) /2a
a,b,c = 6, -17, 12
d = b**2 - 4*a*c
sol1 = (-b - d**0.5)/(2*a)
sol2 = (-b + d**0.5)/(2*a)
print(f"Solutions for the given quadratic equation is {sol1} and {sol2}")
check1 = a*sol1**2 + b*sol1 + c
check2 = a*sol2**2 + b*sol2 + c
print(f"Checking if both the check are zero: {check1} and {check2}")
#Conditions
avg = 30
if avg>=80:
print("You scored Grade A")
elif avg>=70:
print("You scored Grade B")
elif avg>=60:
print("You scored Grade C")
elif avg>=50:
print("You scored Grade D")
elif avg>=40:
print("You scored Grade E")
else:
print("You scored Grade F")
#40%
if avg>=40:
print("You have passed!")
else:
print("Sorry, You have failed!")
if avg >=90:
print("You win President's award")
print("90+ is too good score")
print("Thank You")
#Conditions
avg = 90
#40%
if avg>=40:
print("You have passed!")
if avg >= 80:
print("You scored Grade A")
if avg >= 90:
print("You win President's award")
print("90+ is too good score")
elif avg >= 70:
print("You scored Grade B")
elif avg >= 60:
print("You scored Grade C")
elif avg >= 50:
print("You scored Grade D")
else:
print("You scored Grade E")
print("You have passed!")
else:
print("You scored Grade F")
print("Sorry, You have failed!")
print("Thank You")
###
'''
1. Check for positive, negative, zero
2. If positive then check for Odd or Even
3. If positive check for if its divisible by 2 and 3
4. If its divisible by both 2 and 3 then check for 5 also
'''
num = 30 # 0 -10 10 9
if num>0:
print("Number is positive")
if num%2==0:
print("Its an even number")
if num%3==0:
print("Its divisible by 2 and 3 both")
if num%5==0:
print("Yes, this is divisible by 5 also")
else:
print("Its divisible by 2 but not 3")
else:
print("Its an odd number")
if num%3==0:
print("Its divisible by 3 only")
else:
print("Its not divisible by 2 or 3")
elif num<0:
print("Number is negative")
else:
print("Number is neither positive nor negative")
###
# arrange the numbers in increasing order
# 5,6,3 => 3 >=5 >=6
a,b,c = 80,60,40
if a<b: #a < b
if a<c: #a <c
if b<c: #b <c
print(f"{a}<={b}<={c}")
else: # a<b, a<c, c<b
print(f"{a}<={c}<={b}")
else: #a < b c<a
print(f"{c}<={a}<={b}")
else: # b< a
if a<c: #a <c
if b<c: #b <c
print(f"{b}<={a}<={c}")
else: #b<a c <a
if b<c:
print(f"{b}<={c}<={a}")
else:
print(f"{c}<={b}<={a}")
#loops -
# 1 to 10
#range(a,b,c) - a: starting value (including), b: ending value (excluding), c: increment
#range(2,11,3) - 2,5,8
#range(a,b) - c= default = 1
#range(3,8) - 3,4,5,6,7
#range(b) a default = 0, c default = 1
#range(4) - 0,1,2,3
for i in range(4):
print("Hello, value of i = ",i)
for i in range(3,8):
print("Hi, value of i = ",i)
for i in range(2,11,3):
print("Hello, value of i = ",i)
if 'H' in "hello":
print("There")
sum = 0
for i in range(1,11):
print(i,end=", ")
sum = sum+i
print("\nSum of the values = ",sum)
val = 1
sum = 0
while val <=10:
print(val)
sum += val
val+=1 #val=val+1
print("Sum = ",sum)
n=5
for i in range(n):
print("*",end=" ")
print()
'''
* * * * *
* * * * *
* * * * *
* * * * *
* * * * *
'''
for j in range(n):
for i in range(n):
print("*",end=" ")
print()
'''
*
* *
* * *
* * * *
* * * * *
'''
for j in range(n):
for i in range(j+1):
print("*",end=" ")
print()
'''
* * * * *
* * * *
* * *
* *
*
'''
for j in range(n):
for i in range(n-j):
print("*",end=" ")
print()
'''
*
* *
* * *
* * * *
* * * * *
'''
for j in range(n):
for k in range(n-j-1):
print(" ",end="")
for i in range(j+1):
print("*",end=" ")
print()
'''
*
* *
* * *
* * * *
* * * * *
'''
for j in range(n):
for k in range(n-j-1):
print(" ",end=" ")
for i in range(j+1):
print("*",end=" ")
print()
## read marks of 5 subjects and find sum and avg
num = int(input("Enter length: "))
print("num = ",num)
print("type of num = ",type(num))
sum=0
for i in range(5):
marks = int(input("Enter the marks in subject "+str(i+1)+": "))
sum+=marks
print(f"The total marks is {sum} and average is {sum/5}")
### WHILE
import random
number = random.randint(1,100)
attempt = 0
ch = input("Do you want to play this game? (y for yes): ")
low = 1
high = 100
while ch=="y":
guess = random.randint(low,high) #int(input("Guess the number (1-100): "))
if guess<1 or guess>100:
print("Invalid guess, try again...")
continue # it will take you to the beginning of the loop
attempt+=1
if guess==number:
print(f"Awesome! You guessed it correctly in {attempt} attempts.")
break #throw you out of the loop
elif guess < number:
print("You have guessed a lower number")
low=guess+1
else:
print("You have guessed a higher number")
high=guess-1
print("Thank you")
'''
take 2 numbers and perform operation based on given choice:
1. To add
2. To multiply
3. Divide
4. Quit
'''
while True:
n1 = int(input("Enter a number: "))
n2 = int(input("Enter another number: "))
print("Give your choice of operation:")
print("1. Addition\n2. Multiplication\n3. Division\n4. Quit")
ch=input("Enter your option: ")
if ch=="1":
print("Addition of numbers: ",n1+n2)
elif ch=="2":
print("Multiplication of numbers: ", n1 * n2)
elif ch=="3":
print("Division of numbers: ", n1 / n2)
elif ch=="4":
break
else:
print("Invalid option! Try Again...")
continue
print("Great work!")
#strings
str1 = 'Hello'
str2 = "Hi"
str3 = '''How are you
Where are you'''
str4 = """I am fine
I am here"""
print(type(str1),type(str2),type(str3),type(str4))
print(str3)
print(len(str3)) #size - total characters in a string
print(str1+" "+(str3 +"\n")*3)
for i in str1:
print(i)
for i in range(len(str1)):
print(str1[i])
str1 = "Hello" #indexing starts from zero
print(str1[0])
print(str1[2])
print(str1[4]) #last element
print(str1[-1]) #last element
print(str1[-3]) #3rd last element
print(str1[1:4]) #start is inclusive /end is exclusive
print(str1[:4])
print(str1[:])
print(str1[-4:-1]) #start is inclusive /end is exclusive
print(str1[:-1])
print(str1[-len(str1):-1])
print(str1[-3:])
print(str1[:])
str1 = "Good Evening Everyone"
print(str1[0])
print(str1[-1])
print(str1[-3:])
# functions that are part of a class are called Methods
str2 = "HELLO HOW ARE YOU"
print(str2.isupper())
print(str1.istitle()) #first character of every word needs to be capital
length = input("Enter the length: ")
if length.isdigit():
length = int(length)
else:
print("Invalid length value, exiting the program")
#username can have only characters and numbers
username = input("Enter username: ")
if username.isalnum():
print("Username accepted")
else:
print("Invalid username.")
str1 = "Hello"
print(str1.isalpha())
str3 = " "
print(str3.isspace())
# enter name: alpha and space
name = input("Enter your name")
isValid = True
for ch in name:
if ch.isalpha() or ch.isspace():
pass
else:
isValid = False
break
if isValid:
print("Valid Name")
else:
print("Invalid Name")
str1 = "HOw arE YOU ONE doING ToDaY"
print(str1.lower())
print(str1.upper())
print(str1.capitalize()) #Only the first character is caps
print(str1.title()) #first letter of every word is caps
split_cont = str1.split()
print("Split: ", split_cont)
split_cont = str1.title().split("o")
print("Split: ", split_cont)
print("o".join(split_cont))
str2 = "How Are You Doing Are You Ok Are You Coming"
print(str2.replace("You","You'll",2))
print(str2)
# Strings are IMMUTABLE
#str2[0] = "h" - not possible
str2 = "h"+str2[1:]
print(str2)
print(str2.count("ou",4,10))
print(str2.find("ou",10,22))
DAY 29 – DECEMBER 22 2022
#LIST
l1 = [20,40,20.5,True,"Hello",[2,4,6]]
print(len(l1))
print(l1[1])
print(l1[-1][1])
print(type(l1[-1][1]))
print(type(l1))
l2 = [10,20,30]
print(l1+l2*2)
for i in l2:
print(i)
for i in range(len(l2)):
print(i, "--> ",l2[i])
l2.append(40) #append will add at the end
l2.insert(1,50) #index, value
l2.pop(0) #remove element at the given index
l2.remove(30) #delete the given value
l2[1] = 70
print(l2)
l3 = l2 #both are pointing to same list - Deep copy
l4 = l2.copy() #Shallow copy
print("Set 1")
print("l2: ",l2)
print("l3: ",l3)
print("l4: ",l4)
l2.append(45)
l3.append(70)
l3.append(55)
l4.append(65)
print("Set 2")
print("l2: ",l2)
print("l3: ",l3)
print("l4: ",l4)
print("printing IDs: ")
print("l2: ",id(l2))
print("l3: ",id(l3))
print("l4: ",id(l4))
total_count = l2.count(70)
print(total_count)
print(l2.reverse())
l2.sort()
print(l2)
#l2 = l2 + l3
l2.extend(l2+l3)
print(l2)
print(l2.index(50)) #index of the first matching value
ind = l2.index(50)
print(l2.index(50,ind+1))
marks_masterlist = []
while True:
sum=0
marks_list = [] #student ID, 5 values marks, 7th Sum, 8 avg
id_st = input("Enter the Student ID: ")
marks_list.append(id_st)
for i in range(5):
marks = int(input("Enter the marks in subject "+str(i+1)+": "))
marks_list.append(marks)
sum+=marks
#print("List of marks: ",marks_list)
#print(f"Total marks obtained is {sum} and average is {sum/5}")
avg = sum/5
marks_list.append(sum)
marks_list.append(avg)
marks_masterlist.append(marks_list)
ch=input("Enter N to stop, Enter to continue: ")
if ch:
break
print("Master list:\n",marks_masterlist)
master_list = [['1000', 99, 88, 77, 66, 55, 385, 77.0],
['1002', 33, 44, 55, 66, 77, 275, 55.0],
['1003', 77, 55, 99, 66, 88, 385, 77.0]]
print(len(master_list[0]))
#average of all the students:
for i in range(len(master_list)):
print(master_list[i][0], ":",master_list[i][-1])
#highest marks in each subject
for subcode in range(1,6):
max=-99
for i in range(len(master_list)):
#print(master_list[i][1])
if max <master_list[i][subcode]:
max = master_list[i][subcode]
print(f"Maximum marks in Subject {subcode} is ",max)
#Tuples - linear ordered immutable collection
#List - linear ordered mutable collection
t1 = ()
t1 = (5,)
t1 = (5,6,7)
print(type(t1))
t1 = list(t1)
t1.append(8)
t1 = tuple(t1)
#packing & unpacking
a,b,c,d = t1
print(a)
print(t1[0])
print(16 in t1)
a = (2,99,15)
b = (2,99,15)
#Dictionary - non-linear unordered mutable collection
d1 = {}
print(type(d1))
d1 = {2:"Hello","Two": 999}
print(d1["Two"])
t1 = {True:"Sunday"}
d1.update(t1)
print(d1)
#items: list of tuple of key,value
#keys : list of keys
#values: list of values
print(d1.items())
for i in d1.items():
print(i)
print(d1[2])
for i in d1.items():
if i[0]==2:
print(i[1])
d2 = d1 # points to each other - no new copy created
d3 = d1.copy() # at this point they are copy
t2= {99:"Ninety Nine"}
d1.update(t2)
print(d1)
print(d2)
print(d3)
d1.popitem()
d1.pop(True)
print(d1)
#to get the value when key is given:
a= d1.get(2)
print(a)
#print(d1[888])
d1.clear()
print(d1)
master_data = {}
student = 0
while True:
student+=1
roll = input("Enter the Roll Number for Student "+str(student)+": ")
sum=0
list1 = []
for i in range(3):
m = int(input("Enter marks for subject "+str(i+1)+ " for student "+str(student)+" :"))
sum+=m
list1.append(m)
master_data.update({roll:list1})
ch=input("Hit Enter to continue else to stop: ")
if ch:
break
print("All data: \n",master_data)
#{'1': [5, 5, 5], '2': [8, 8, 8]}
#Set - - linear un-ordered mutable collection
# Lion Tiger Elephant Deer Panther
# P D E T L
# PDE Jaguar Camel
# total how many were named: 7 - Union
# Common between S1 & S2 : 3 - Intersection
#Remove animals from S1 as told by S2, what is left: 2 L & T - Minus (S1-S2)
# S2 - S1 : J & C
# Symmetric Difference: L, T, J , C
# 1. L 2 L 3 L 4L 5L
#SET
set1 = {1,2,3,4,5}
set2 = {3,4,5,6,7,8}
print(type(set1))
#union - giving you all data (minus dup)
print(set1.union(set2)) #max - sum of the # of elements
print(set1 | set2) #min - # of the elements in bigger set
print(set1.intersection(set2)) #max: size of lower set
print(set1 & set2) #min : no elements repeated
#if no elements are repeated we call them disjoint set
seta={1,2,3}
setb={1,2,3}
setc = {1,2,3,4,5}
#setc is superset of seta, seta as subset of setc
#seta = setb = union = intersection: seta is superset as well as subset of setb
print(set1.difference(set2))
print(set1-set2)
print(set2-set1)
print(set1.symmetric_difference_update(set2))
print(set1 ^ set2)
print(set1)
#update() -union update
#intersection_update() set1 = set1 & set2
#difference_update()
#symmetric_difference_update()
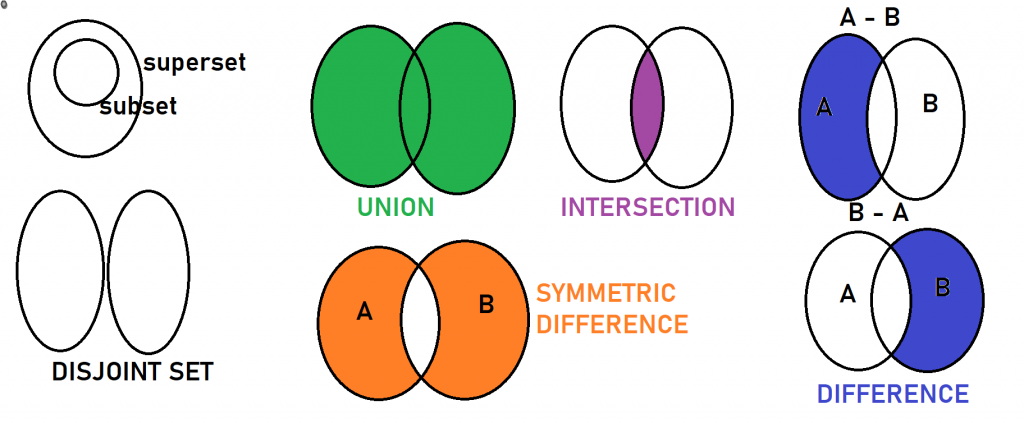
# Functions
##print()
##type()
def myquestions():
print("How are you",end="? ")
print("Hows going")
print("Where are you")
def myadd(a,b): #arguments #positional #required
print("A: ",a)
print("B: ", b)
sum = a+b
#return sum
#default arguments
def myadd2(a=5,b=0,c=4,d=4,e=5): #arguments #positional #a required b as default
print("A: ",a)
print("B: ", b)
sum = a+b
myquestions()
result = myadd(55,65) #passing the parameter to the function definition
print(result)
myadd2(55,35)
myadd2(55)
myadd2()
#keywords = removes restriction of positional
a=5
b=6
myadd(b=b,a=a) #not being positional - keyword
#required positional default keyword
#global and local scope of variable
def mytest():
global a
print("Inside mytest 2 a = ", a)
a=5 #local variable
#always local takes preference over global
print("Inside mytest 1 a = ",a)
a=10 #global variable
print("printing in main a =",a)
mytest()
#Functions
#DocString - are multi line comments added as first line in function definition
def myfun1(a,b): #required positional argument
'''
This is a sample function to learn about function. This will perform some basic math ops
:param a: this is a number that will take part in math operation
:param b: this is another number that will take part in math operation
:return: a tuple with 3 values - each for addition, multiplication and division
'''
s = a+b
m = a*b
d = a/b
result = [s,m,d] #packing
print(type(result))
return result
def myfun2(a=10,b=20): #default arguments
s = a+b
m = a*b
d = a/b
result = [s,m,d] #packing
print(type(result))
return result
def myfun3(roll,*marks,**attr): # *var -all arguments, **var will read all your keyword arg
print("Roll No:",roll)
print("Marks obtained: ",marks)
print(type(marks))
print("Attributes of student: ",attr)
print(type(attr))
if __name__ =='__main__':
output1 = myfun1(10,5)
print("Output: ",output1)
output1 = myfun2()
print("Output: ",output1)
output1 = myfun2(b=10,a=20)
print("Output: ",output1)
output1 = myfun3(1001, 65,76,87,56,78,76,78,name="Sachin",sports="Cricket",city="Mumbai")
print(help(print)) #doc string
print(print.__doc__)
print(myfun1.__doc__)
#######################
import p1 #import module - file containing functions
out1 = p1.myfun2(b=10,a=20)
print(out1)
out1 = p1.myfun1(10,20)
print(out1)
#annoynomous or oneline or lambda
lf1 = lambda x,y:x+y
print(lf1(10,90))
# recursion - function calling itself
def myfun_facto(n):
if n==0:
return 1
return n * myfun_facto(n-1) #stack
print(myfun_facto(5))
# 5! = 5 * 4 * 3 * 2 * 1
# 5! = 5 * 4!
#Class & Object
class Person:
population=0
def __init__(self,name):
#this function should be called automatically when you create an object
Person.population += 1
self.name = name
def display_info(self):
print(f"Hello How are you? My name is {self.name}")
@classmethod
def display_pop(cls):
print("Population is ",cls.population)
o1=Person("Sachin") #auto calling of __init__
o2=Person("Kapil") #auto calling of __init__
o3=Person("Sunil") #auto calling of __init__
o4=Person("Dhoni") #auto calling of __init__
o5=Person("Virat") #auto calling of __init__
o1.display_info()
o2.display_info()
o3.display_info()
#o4.display_info()
#o5.display_info()
print(type(o1))
print(o1.population)
print(o3.population)
print(o1.name)
print(o3.name)
l1 = [3,4,5]
l1.append(5)
#Inheritance
#Encapsulation
#Polymorphism
#Abstraction
################
class School:
def __init__(self,schoolname):
self.schoolname = schoolname
def display_school(self):
print("School name is: ",self.schoolname)
self.__display_state()
def display_loc(self):
print("We are located in the City")
def __display_state(self):
print("We are located in the State")
class Student(School):
def __init__(self,sname, stname):
School.__init__(self,sname)
self.sname = sname
self.stname = stname
def display_student(self):
print("Student name is: ",self.stname)
def display_loc(self):
print("Calling from STUDENT Class: We are located in the City")
class MySelf:
def MySelfMethod(self):
School.display_loc(self)
s1 = School("ABC International School")
s1.display_school()
st1 = Student("XYZ Intermational School","Sachin")
st1.display_school()
st1.display_student()
st1.display_loc()
#st1.__display_state()
m1 = MySelf()
m1.MySelfMethod()
#Encapsulation:
# public - the members are available to be accessed by anyone
# protected (_membername) members can be called only
# its own and derived class: Practically not implemented in Python
# private options (__membername) - cant be accessed outside the class
from abc import ABCMeta, abstractmethod
class Shape(object):
__metaclass__ = ABCMeta
def __init__(self):
print("Shape is created")
@abstractmethod
def area(self):
print("I will do nothing")
def information(self):
print("I belong to class Shape")
class Rectangle(Shape):
def __init__(self):
print("rectangle is created")
class Circle(Shape):
def __init__(self):
print("circle is created")
s1 = Shape()
c1 = Circle()
c1.area()
s1.area()
c1.information()
PYTHON PROJECT WORK
import sqlite3
con_str = sqlite3.connect("ies1.db")
creat_tab ='''Create Table Students(
Roll Integer primary key,
name text,
fees real,
admission_date date)'''
#con_str.execute(creat_tab)
insrt1 = '''Insert into Students(ROll,Name) values(1001,'Sachin')'''
#con_str.execute(insrt1)
insrt1 = '''Insert into Students(ROll,Name) values(1002,'Kapil')'''
#con_str.execute(insrt1)
insrt1 = '''Insert into Students(ROll,Name) values(1003,'Sunil')'''
#con_str.execute(insrt1)
insrt1 = '''Insert into Students(ROll,Name) values(1004,'Kohli')'''
#con_str.execute(insrt1)
insrt1 = '''Insert into Students(ROll,Name) values(1005,'Dhoni')'''
#con_str.execute(insrt1)
select_q = "Select * from Students"
cursor_obj = con_str.execute(select_q)
rows = cursor_obj.fetchall()
for row in rows:
print(row)
#update
update_q = '''Update Students set fees=110 where roll=1003'''
con_str.execute(update_q)
con_str.commit()
#Delete
delete_q = '''Delete from Students where roll=1004'''
con_str.execute(delete_q)
##insert based on user input
roll_no = int(input("Enter the roll number: "))
name_st = input("Enter the name: ")
insrt1 = '''Insert into Students(ROll,Name) values(?,?)'''
dy_val = (roll_no,name_st)
con_str.execute(insrt1,dy_val)
con_str.commit()
##########################
### WORKING WITH FILES ##
##########################
file_con = open("abc1.txt",mode="a") #default is read
# r read - only read, w write - you can write, a append - you can only append
# r+ , w+ , a+
content = '''Twinkle Twinkle Little Star
How I wonder what you are'''
if file_con.writable():
file_con.write(content)
fh=open("file1.txt","a")
story='''Once upon a time, there lived a deer in the forest. He was a gentle creature who loved to explore the forest and make new friends. He often encountered various animals on his explorations, including a lion.
The deer and the lion were wary of each other when they first met, but soon they developed a mutual respect and even a friendship. The lion was impressed with the deer's courage and wisdom, while the deer admired the lion's strength and nobility.
The two began to meet often, and they would often go on adventures together. The lion would show the deer the best spots to find food and the deer would help the lion hunt. They both enjoyed the company of each other, and the friendship between them grew stronger.
One day, the deer and the lion were out exploring the forest when they came upon a group of hunters. The deer was terrified and wanted to run away, but the lion saw the hunters and told the deer to stay put. The lion then charged at the hunters, roaring and showing his full strength. The hunters were so scared that they ran away, leaving the deer and the lion unharmed.
The deer was so grateful for the lion's bravery that he promised to always be there for his friend. From that day on, the deer and the lion remained inseparable. They went on countless adventures together, and they were always there to help each other when they needed it.'''
fh.write(story)
fh.close()
fh=open("file1.txt","r")
#content = fh.read(200)
#print(content)
fh.seek(100)
content = fh.read(300)
#print(content)
fh.seek(0)
print(fh.readline(50000))
print(fh.readlines())
fh.close()
############### JSON
#JavaScript Object Notation - json
json_str = '''
{"Name":"Sachin",
"Sport":"Cricket",
"Fav Shots":["Cover Drive","Square Cut"],
"Teams":[
{
"TName":"Mumbai",
"Games": "Ranji"
},
{
"TName":"Mumbai Indians",
"Games": "IPL"
}
]}
'''
print(json_str)
print(type(json_str))
import json
# 4 methods: load(), loads(), dump(), dumps()
json_obj = json.loads(json_str)
print(type(json_obj))
print(json_obj["Teams"])
json_dumps = json.dumps(json_obj,indent=4,sort_keys=True)
print(json_dumps)
fp=open("json_file.json","w")
json.dump(json_obj,fp,indent=4)
fp.close()
import json
##
fp=open("json_file.json","r")
file_content = json.load(fp)
print(file_content)
print(type(file_content))
fp.close()
##### Exceptions
print("Hello")
print(5-3)
#runtime errors - Exceptions
try:
num1 = int(input("Enter a number: ")) # ValueError exception
50 / 0 # ZeroDivisionError exception
except ValueError:
print("You have not entered a valid number hence setting it to zero")
except ZeroDivisionError:
print("Sorry cant divide by zero")
except Exception:
print("Some error has occured")
else: #if there is no error then do else
print("Number you have entered is ",num1)
finally: #will execute in both cases - error or no error
print("Demo program for exception handling")
#50/0 #ZeroDivisionError exception
# try - except - else -finally
import numpy as np
x = range(16) #0...15
x=np.reshape(x,(4,4))
print(x[1:3,:3])
y = range(11,27)
y=np.reshape(x,(4,4))
print(y)
print(x+y)
print(x-y)
print(x*y) #element multiplication
print(x@y) #matrix multiplication
print(x/y)
### Pandas
import pandas as pd
data = [["Apple",1200,2100,900,870],
["Oranges",500,900,300,400],
["Banana",132,456,768,333],
["Mangoes", 1111,3333,2222,4444]]
print(data)
headers=['Fruit',"Q1 2022","Q2 2022","Q3 2022","Q4 2022"]
inx = ["Fruit 1","Fruit 2","Fruit 3","Fruit 4"]
data_df = pd.DataFrame(data,columns=headers,index=inx)
print(data_df)
import pandas as pd
#you can read csv files using read_csv irrepective of the location
usage_df = pd.read_csv("C:/Users/Hp/Downloads/Dataset-master/Dataset-master/user_usage.csv")
print(usage_df)
device_df = pd.read_csv("C:/Users/Hp/Downloads/Dataset-master/Dataset-master/user_device.csv")
print(device_df)
#merge these two datasets
result_df = pd.merge(usage_df,device_df, on="use_id",how="right")
print(result_df)
#########################################
######### EXAMPLE: DATA CLEANING ######
#########################################
#missing data?
#data correct? type, range
#
import pandas as pd
hotel_df = pd.read_csv("C:/Users/Hp/Downloads/Dataset-master/Dataset-master/hotel_bookings.csv")
print(hotel_df)
import matplotlib.pyplot as plt
import seaborn as sns
plt.style.use('ggplot')
cols = hotel_df.columns[:30]
colors = ["#085838","#f4deb4"]
sns.heatmap(hotel_df[cols].isnull(), cmap=sns.color_palette(colors))
plt.show()
import pandas as pd
hotel_df = pd.read_csv("C:/Users/Hp/Downloads/Dataset-master/Dataset-master/hotel_bookings.csv")
print(hotel_df)
#Data cleaning
## 1. missing values - 1)remove the values 2)replace the missing values
## 2. Incorrect data - 1) extreme values, not possible, different data type - make it null
import matplotlib.pyplot as plt
import seaborn as sns
cols = hotel_df.columns[:30]
colors = ["#2b80ff","#05f6d5"]
sns.heatmap(hotel_df[cols].isnull(),cmap=sns.color_palette(colors))
#plt.show()
#dropping column company since it has more than 95% values missing
hotel_df = hotel_df.drop(['company'],axis=1) #axis=1 for column
#check % missing
import numpy as np
for col in hotel_df.columns:
pct_missing = np.mean(hotel_df[col].isnull())
print(f"{col} has {pct_missing*100}%")
#calculate total missing values for each row
for col in hotel_df.columns:
missing = hotel_df[col].isnull()
num_missing = np.sum(missing)
if num_missing>0:
hotel_df[f'{col}_ismissing'] =missing
ismissing_col = []
for col in hotel_df.columns:
if 'ismissing' in col:
ismissing_col.append(col)
#total the values of missing cols for each row:
hotel_df['num_missing'] = hotel_df[ismissing_col].sum(axis=1)
print("Missing data: ",ismissing_col)
ind_missing = hotel_df[hotel_df['num_missing']>12].index
#drop the rows which has more than 12 missing values
hotel_df=hotel_df.drop(ind_missing,axis=0) #axis=0 for rows
#check again after rows and col drop
import numpy as np
for col in hotel_df.columns:
pct_missing = np.mean(hotel_df[col].isnull())
if pct_missing>0.0:
print(f"{col} has {pct_missing*100}%")
'''
children has 2.0498257606219004%
babies has 11.311318858061922%
meal has 11.467129071170085%
country has 0.40879238707947996%
deposit_type has 8.232810615199035%
agent has 13.68700576330250
'''
hotel_df['meal'] = pd.Categorical(hotel_df['meal'])
hotel_df['country'] = pd.Categorical(hotel_df['country'])
hotel_df['deposit_type'] = pd.Categorical(hotel_df['deposit_type'])
med = hotel_df['children'].median()
hotel_df['children'] = hotel_df['children'].fillna(med)
med = hotel_df['babies'].median()
hotel_df['babies'] = hotel_df['babies'].fillna(med)
med = hotel_df['agent'].median()
hotel_df['agent'] = hotel_df['agent'].fillna(med)
non_num_cols = hotel_df.select_dtypes(exclude=np.number)
for col in non_num_cols:
missing = hotel_df[col].isnull()
num_missing = np.sum(missing)
if num_missing > 0:
#replace the missing values with mode
top = hotel_df[col].describe()['top']
hotel_df[col] = hotel_df[col].fillna(top)
print("After update the missing columns are:")
#check again for missing values
for col in hotel_df.columns:
missing = hotel_df[col].isnull()
num_missing = np.sum(missing)
if num_missing > 0:
print(f"{col} has total missing values {pct_missing}")
#####
hotel_df['children'] = hotel_df['children'].fillna(-999)
hotel_df['country'] = hotel_df['country'].fillna("NOT AVAILABLE")
This is a practice excercise which requires you to clean and merge dataset belong to different categories but having same kind of information.
1. Clean the data
2. Add category
3. Merge the data
4. Now prepare viz