Programming with Hari
print(‘Hello’)
print() #comment is for human being
#sdfsdfdsfsdfdsf
print(‘hello again hhjvjbhjhlbbuihuhuhuhu’)
print(‘5+3’)
print(5+3)
print(“4+6=”,4+6)
# y = x + 5, find y when x is 3
# 5 is constant – you cant change its value
# x, y are variables – their values can change
# y dependent variable because its value depends on x
# x is independent variable because you can assign any value to it
x = 3
y = x+ 5
print(“When X is “,x,“then Y is “,y)
# write a program to calculate area of a rectangle
l = 5
b = 8
area = l * b
print(” Length is”,l,“breadth is”,b,“and area is”,area)
# f-string format string
print(f“Length is {l} and breadth is {b} so the area is {area}“)
#calculate area and circumference of a circle and print the output as:
# Circle with a radius will have area of area and circumference of circumference
# arithematic
val1 , val2 = 25,20
print(val1 + val2)
print(val1 – val2)
print(val1 * val2)
print(val1 / val2)
print(val1 // val2) # integer division
print(val1 % val2) # mod – remainder
print(5 ** 3) #power
#relational operators: > < >= <= == !=
#output is always – True or False
val1, val2, val3 = 25,20,25
print(val1 > val2) #T
print(val1 > val3) #F
print(val1 >= val3) #T
print(val1 < val2) #F
print(val1 < val3) #F
print(val1 <= val3) #T
print(val1 == val2) #F
print(val1 != val3) #F
print(val1 == val3) #T
# Logical operators: input &output are bool: and , or, not
print(“Logical operators”)
print(True and True) #I did both the tasks
print(False and True)
print(True and False)
print(False and False)
print(True or True) #T
print(False or True) #T
print(True or False) #T
print(False or False) #F
print(not True)
print(not False)
r= –5
if r>0:
area = 22/7*(r**2)
print(“area of the circle is”,area)
else:
print(“Invalid data”)
num=4
if num>0:
print(“Positive”)
elif num<0:
print(“Negative”)
else:
print(“Neither positive not negative”)
val1 , val2 = 25,20
print(val1 + val2)
print(val1 – val2)
print(val1 * val2)
print(val1 / val2)
print(val1 // val2) # integer division
print(val1 % val2) # mod – remainder
print(5 ** 3) #power
#relational operators: > < >= <= == !=
#output is always – True or False
val1, val2, val3 = 25,20,25
print(val1 > val2) #T
print(val1 > val3) #F
print(val1 >= val3) #T
print(val1 < val2) #F
print(val1 < val3) #F
print(val1 <= val3) #T
print(val1 == val2) #F
print(val1 != val3) #F
print(val1 == val3) #T
# Logical operators: input &output are bool: and , or, not
print(“Logical operators”)
print(True and True) #I did both the tasks
print(False and True)
print(True and False)
print(False and False)
print(True or True) #T
print(False or True) #T
print(True or False) #T
print(False or False) #F
print(not True)
print(not False)
r= –5
if r>0:
area = 22/7*(r**2)
print(“area of the circle is”,area)
else:
print(“Invalid data”)
num=4
if num>0:
print(“Positive”)
elif num<0:
print(“Negative”)
else:
print(“Neither positive not negative”)
# wap to check if a number is divisible by 3 or not
# wap to check if a number is odd or even
# wap to check if a number is odd or even
# for: when you know how many times you need to repeat something
# while:
# range(=start, < end, =increment) range(3,9,2): 3,5,7
for counter in range(3,9,2):
print(“HELLO, The counter value is”,counter)
##
for i in range(1,101): #when increment is not given, default value is 1
print(i)
#Generate even numbers from 2 to 100 (both inclusive)
n=12
for i in range(n): #when start number is not given, default value is zero
print(“* “,end=“”)
print()
”’
* * * * * * *
* * * * * * *
* * * * * * *
* * * * * * *
* * * * * * *
* * * * * * *
* * * * * * *
”’
for j in range(n):
for i in range(n):
print(“* “,end=“”)
print()
”’
*
* *
* * *
* * * *
”’
for j in range(n):
for i in range(j+1):
print(“* “,end=“”)
print()
”’
* * * *
* * *
* *
*
”’
for j in range(n):
for i in range(n-j):
print(“* “,end=“”)
print()
”’
* * * *
*
*
* * * *
”’
for j in range(n):
if j==0 or j==n-1:
for i in range(n):
print(“* “,end=“”)
print()
else:
for k in range(n-j-1):
print(” “,end=” “)
print(“*”)
#while
cont = “y”
while cont==“y”:
print(“Hello from While”)
cont = input(“Do you want to continue: hit y for yes anyother key for no:”)
# while:
# range(=start, < end, =increment) range(3,9,2): 3,5,7
for counter in range(3,9,2):
print(“HELLO, The counter value is”,counter)
##
for i in range(1,101): #when increment is not given, default value is 1
print(i)
#Generate even numbers from 2 to 100 (both inclusive)
n=12
for i in range(n): #when start number is not given, default value is zero
print(“* “,end=“”)
print()
”’
* * * * * * *
* * * * * * *
* * * * * * *
* * * * * * *
* * * * * * *
* * * * * * *
* * * * * * *
”’
for j in range(n):
for i in range(n):
print(“* “,end=“”)
print()
”’
*
* *
* * *
* * * *
”’
for j in range(n):
for i in range(j+1):
print(“* “,end=“”)
print()
”’
* * * *
* * *
* *
*
”’
for j in range(n):
for i in range(n-j):
print(“* “,end=“”)
print()
”’
* * * *
*
*
* * * *
”’
for j in range(n):
if j==0 or j==n-1:
for i in range(n):
print(“* “,end=“”)
print()
else:
for k in range(n-j-1):
print(” “,end=” “)
print(“*”)
#while
cont = “y”
while cont==“y”:
print(“Hello from While”)
cont = input(“Do you want to continue: hit y for yes anyother key for no:”)
num = 10
while num >0:
print(“hello”)
num=num-1
ch=‘y’
while ch==‘y’ or ch==“Y”:
print(“I am fine”)
ch=input(“Do you want to continue (y for yes):”)
while True:
print(“How are you?”)
ch=input(“Enter n to stop:”)
if ch==“n”:
break #that will throw you out of the loop
# guessing game
num = 45
while True:
guess = int(input(“Guess the number (1-100): “))
if guess<1 or guess>100:
continue
if num==guess:
print(“Congratulations… You have guessed it correctly!”)
break
elif num<guess:
print(“Incorrect! You have guessed higher number”)
else:
print(“Incorrect! You have guessed lower number”)
## Menu Options
print(“WELCOME TO MY LIBRARY APPLICATION”)
while True:
print(“1. Issue the Book”)
print(“2. Return the Book”)
print(“3. Add new Book to the library”)
print(“4. Remove a book from the library”)
print(“5. Exit”)
choice = input(“Enter you choice: “)
if choice==“1”:
print(“Given book has been issued!”)
elif choice==“2”:
print(“Book has been returned to the library!”)
elif choice==“3”:
print(“Added the book to the library!”)
elif choice==“4”:
print(“Removed the book from the library database!”)
elif choice==“5”:
print(“Have a good day!”)
break
else:
print(“Invalid option! Try again”)
continue
while num >0:
print(“hello”)
num=num-1
ch=‘y’
while ch==‘y’ or ch==“Y”:
print(“I am fine”)
ch=input(“Do you want to continue (y for yes):”)
while True:
print(“How are you?”)
ch=input(“Enter n to stop:”)
if ch==“n”:
break #that will throw you out of the loop
# guessing game
num = 45
while True:
guess = int(input(“Guess the number (1-100): “))
if guess<1 or guess>100:
continue
if num==guess:
print(“Congratulations… You have guessed it correctly!”)
break
elif num<guess:
print(“Incorrect! You have guessed higher number”)
else:
print(“Incorrect! You have guessed lower number”)
## Menu Options
print(“WELCOME TO MY LIBRARY APPLICATION”)
while True:
print(“1. Issue the Book”)
print(“2. Return the Book”)
print(“3. Add new Book to the library”)
print(“4. Remove a book from the library”)
print(“5. Exit”)
choice = input(“Enter you choice: “)
if choice==“1”:
print(“Given book has been issued!”)
elif choice==“2”:
print(“Book has been returned to the library!”)
elif choice==“3”:
print(“Added the book to the library!”)
elif choice==“4”:
print(“Removed the book from the library database!”)
elif choice==“5”:
print(“Have a good day!”)
break
else:
print(“Invalid option! Try again”)
continue
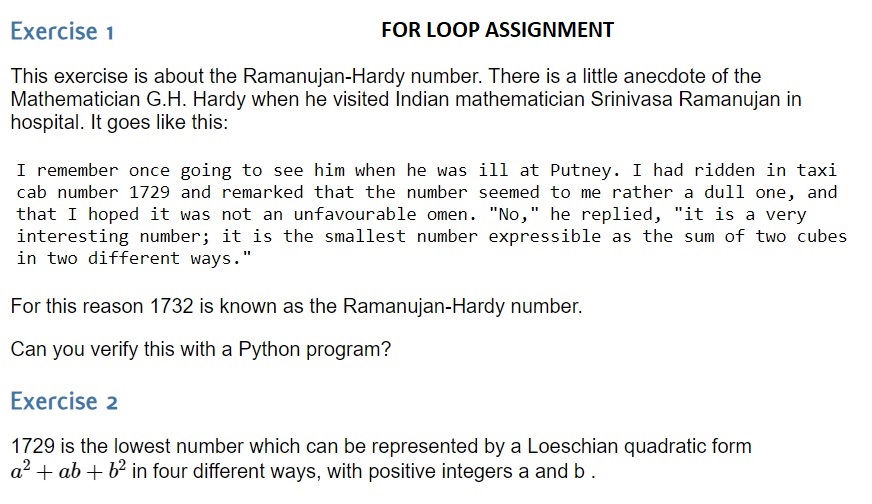
####
str1 = 'HELLO'
str2 = "I am fine"
str3 = '''Where are you going?
How long will you be here?
What are you going to do?'''
str4 = """I am here
I will be here for next 7 days
I am going to just relax and chill"""
print(type(str1),type(str2),type(str3),type(str4))
print(str1)
print(str2)
print(str3)
print(str4)
# What's you name?
str5 = "What's your name?"
print(str5)
#He asked,"Where are you?"
str6 = 'He asked,"Where are you?"'
print(str6)
#He asked,"What's your name?"
#escape sequence \
print('''He asked,"What's your name?"''')
print("He asked,\"What's your name?\"")
print('nnnnn\nnn\tnn')
print("\FOlder\\newfolder")
# \n is used to print newline in python
print("\\n is used to print newline in python")
# \\n will not print newline in python
print("\\\\n will not print newline in python")
str1 = "Hello You"
str2 = "There"
print(str1 + str2)
print(str1 *5)
for i in str1:
print("Hello")
#indexing
print(str1[2])
print("last element: ",str1[4])
print("last element: ",str1[-1])
print("second element: ",str1[-8])
print("ell: ",str1[1:4])
print("ell: ",str1[-8:-5])
print("First 3: ",str1[:3])
print("First 3: ",str1[:-6])
print("Last 3: ",str1[6:])
print("Last 3: ",str1[-3:])
#Methods - exactly same as your functions - only difference is they are linked to a class
import time
str1 = "HELLO"
print(str1.replace("L","X",1))
sub_str = "LL"
str2 = "HELLO HOW WELL ARE YOU LL"
cnt = str2.find(sub_str)
print("Count = ",cnt)
if cnt<0:
print("Sorry, no matching value hence removing")
else:
print("Value found, now replacing")
for i in range(5):
print(". ",end="")
time.sleep(0.5)
print("\n")
print(str2.replace(sub_str,"OOOO"))
out_res = str2.split("LL")
print("Output Result = ",out_res)
out_str = "LL".join(out_res)
print(out_str)
print(str2.title())
print(str2.lower())
print(str2.upper())
str3 = 'hello how well are you ll'
print(str3.islower())
print(str3.isupper())
num1 = input("Enter a number: ")
if num1.isdigit():
num1 = int(num1)
else:
print("Invaid input")
ename = input("Enter your first name: ")
if ename.isalpha():
print("Your name is being saved...")
else:
print("Invaid name")
#WAP to count of vowels in a sentence
para1 = "Work, family, and endless to-do lists can make it tough to find the time to catch up. But you'll never regret taking a break to chat with your friend, Frost reminds us. Everything else will still be there later."
sum=0
for l in para1:
if l=='a' or l=='A' or l=='e' or l=='E' or l=='i' or l=='I' or l=='o' or l=='O' or l=='u' or l=='3':
sum+=1
print("Total vowesl = ",sum)
sum=0
for l in para1.lower():
if l=='a' or l=='e' or l=='i' or l=='o' or l=='u':
sum+=1
print("Total vowesl = ",sum)
sum=0
for l in para1.lower():
if l in 'aeiou':
sum+=1
print("Total vowesl = ",sum)
#Strings – indexing
str1 = “Hello”
print(“=>”, str1[0]+str1[2]+str1[4])
#methods in Python
print(str1.isdigit())
num1 = input(“Enter a number: “)
if num1.isdigit():
num1 = int(num1)
print(num1)
else:
print(“Sorry you have entered a invalid number”)
username = “abcd3456”
if username.isalnum():
print(“Valid username”)
else:
print(“Username is not valid, enter again!”)
username=“abcd”
print(“alpha: “,username.isalpha())
print(“isalnum: “,username.isalnum())
print(“isdigit: “, username.isdigit())
txt1 = “Hello how are you are you are doing”
print(“lower: “,txt1.islower()) #isupper(), istitle()
print(“title: “,txt1.title())
print(“title: “,txt1.istitle())
# islower() -> lower(), isupper() -> upper(), istitle() -> title()
print(“=> “,txt1.split())
print(“=> “,txt1.split(“o”))
txt_list1 = [‘Hello’, ‘how’, ‘are’, ‘you’, ‘doing’]
txt_list2 = [‘Hell’, ‘ h’, ‘w are y’, ‘u d’, ‘ing’]
print(“Join = “, ” “.join(txt_list1))
print(“Join = “, “o”.join(txt_list2))
print(“”,txt1.count(“are”,10,25))
print(“”,txt1.count(“hello”))
print(” “,txt1.replace(“are”,“is”))
str1 = “Hello”
print(“=>”, str1[0]+str1[2]+str1[4])
#methods in Python
print(str1.isdigit())
num1 = input(“Enter a number: “)
if num1.isdigit():
num1 = int(num1)
print(num1)
else:
print(“Sorry you have entered a invalid number”)
username = “abcd3456”
if username.isalnum():
print(“Valid username”)
else:
print(“Username is not valid, enter again!”)
username=“abcd”
print(“alpha: “,username.isalpha())
print(“isalnum: “,username.isalnum())
print(“isdigit: “, username.isdigit())
txt1 = “Hello how are you are you are doing”
print(“lower: “,txt1.islower()) #isupper(), istitle()
print(“title: “,txt1.title())
print(“title: “,txt1.istitle())
# islower() -> lower(), isupper() -> upper(), istitle() -> title()
print(“=> “,txt1.split())
print(“=> “,txt1.split(“o”))
txt_list1 = [‘Hello’, ‘how’, ‘are’, ‘you’, ‘doing’]
txt_list2 = [‘Hell’, ‘ h’, ‘w are y’, ‘u d’, ‘ing’]
print(“Join = “, ” “.join(txt_list1))
print(“Join = “, “o”.join(txt_list2))
print(“”,txt1.count(“are”,10,25))
print(“”,txt1.count(“hello”))
print(” “,txt1.replace(“are”,“is”))
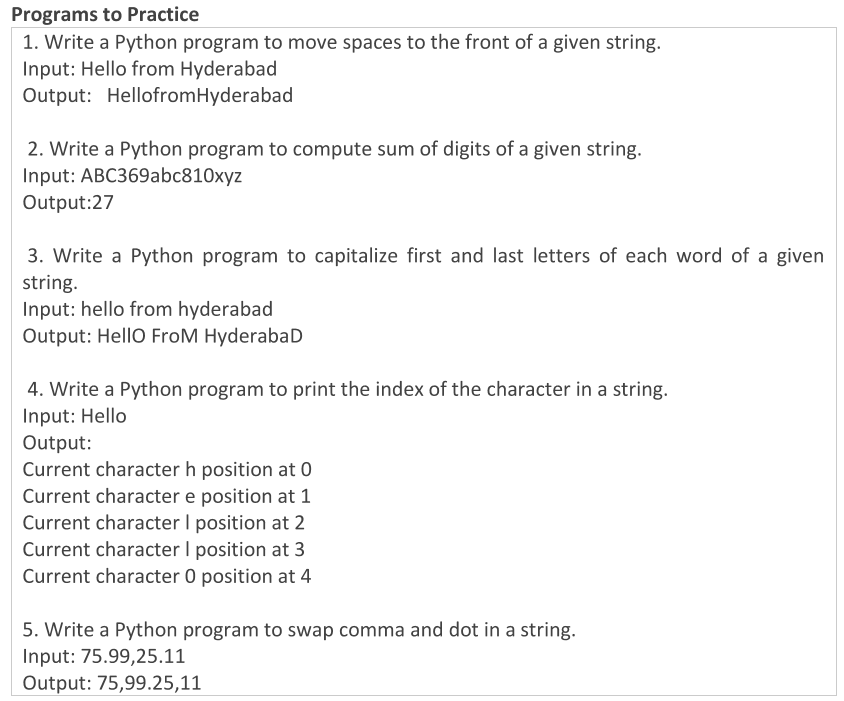
COMPLETE THE PYTHON COURSE FROM HERE:
https://www.netacad.com/
Direct course link: https://www.netacad.com/courses/programming/pcap-programming-essentials-python
# List: linear ordered mutable collection
list1 = [5,6.1,“Hello”,True,[2,4,9]]
# mutable means you can edit the list
list1[1] = 6.9
print(“List 1 = “,list1)
# non mutables (immutable) objects cant be edited eg. String
str1 = “Hello”
#str1[1]= “Z” #TypeError: ‘str’ object does not support item assignment
print(“You can iterate through the list:”)
for i in list1:
print(i)
list2 = [3,6,9,12]
list3 = [4,8,12,16]
print(“list2 + list3: “,list2 + list3)
print(“last member = “,list1[-1])
print(“First 4 values = “,list1[:4])
print(“Number of elements in List 1 = “,len(list1))
### Methods in List ###
list2 = [3,6,9,12]
list2.append(18)
print(“List 2 after append: “,list2)
list2.append(15)
print(“List 2 after append 2: “,list2)
# add the element at a specific position
list2.insert(1,24)
print(“List 2 after insert: “,list2)
## removing
list2.pop(2) #pop takes the index value
print(“List 2 after pop: “,list2)
list2.remove(18) #remove takes the value of the element to remove
print(“List 2 after remove: “,list2)
list4 = list2
list5 = list2.copy()
print(“1. List 2 = “,list2)
print(“1. List 4 = “,list4)
print(“1. List 5 = “,list5)
list2.append(30)
list2.append(40)
print(“2. List 2 = “,list2)
print(“2. List 4 = “,list4)
print(“2. List 5 = “,list5)
print(“10 in the list = “,list2.count(10))
list2.reverse()
print(“List after reverse = “,list2)
list2.sort() #sort in the ascending order
print(“Sorted list = “,list2)
list2.sort(reverse=True) #sort in the descending order
print(“Sorted list = “,list2)
list2.clear()
print(“List after clear = “,list2)
list1 = [5,6.1,“Hello”,True,[2,4,9]]
# mutable means you can edit the list
list1[1] = 6.9
print(“List 1 = “,list1)
# non mutables (immutable) objects cant be edited eg. String
str1 = “Hello”
#str1[1]= “Z” #TypeError: ‘str’ object does not support item assignment
print(“You can iterate through the list:”)
for i in list1:
print(i)
list2 = [3,6,9,12]
list3 = [4,8,12,16]
print(“list2 + list3: “,list2 + list3)
print(“last member = “,list1[-1])
print(“First 4 values = “,list1[:4])
print(“Number of elements in List 1 = “,len(list1))
### Methods in List ###
list2 = [3,6,9,12]
list2.append(18)
print(“List 2 after append: “,list2)
list2.append(15)
print(“List 2 after append 2: “,list2)
# add the element at a specific position
list2.insert(1,24)
print(“List 2 after insert: “,list2)
## removing
list2.pop(2) #pop takes the index value
print(“List 2 after pop: “,list2)
list2.remove(18) #remove takes the value of the element to remove
print(“List 2 after remove: “,list2)
list4 = list2
list5 = list2.copy()
print(“1. List 2 = “,list2)
print(“1. List 4 = “,list4)
print(“1. List 5 = “,list5)
list2.append(30)
list2.append(40)
print(“2. List 2 = “,list2)
print(“2. List 4 = “,list4)
print(“2. List 5 = “,list5)
print(“10 in the list = “,list2.count(10))
list2.reverse()
print(“List after reverse = “,list2)
list2.sort() #sort in the ascending order
print(“Sorted list = “,list2)
list2.sort(reverse=True) #sort in the descending order
print(“Sorted list = “,list2)
list2.clear()
print(“List after clear = “,list2)
# Tuples – linear ordered immutable collection
t1 = (4,6,7,8)
print(type(t1))
for i in t1:
print(i)
t1 = list(t1)
t1.append(10)
t1 = tuple(t1)
t1 = (4,6,7,8)
print(type(t1))
for i in t1:
print(i)
t1 = list(t1)
t1.append(10)
t1 = tuple(t1)
# Dictionary : key:value pair
dict1 = {}
print(type(dict1))
dict1 = {3:“Sachin”,“Second”:2,True:False,“Flag”:True}
# 3 ways – keys, values, items
for k in dict1.keys(): #keys
print(“Key = “,k,” and value is”,dict1[k])
for values in dict1.values():
print(“Value is”,values)
# iterate through items:
for item in dict1.items():
print(item,“: key is”,item[0],“and value is”,item[1])
# only a dictionary can be added to a dictionary
temp = {“City”:“Mumbai”}
dict1.update(temp)
print(“Dictionary 1: “,dict1)
#dictionary – mutable [list is mutable, tuple and string – immutable]
dict1[3]=“Tendulkar”
print(“Dictionary 1: “,dict1)
#copy
dict2 = dict1
dict3 = dict1.copy()
print(“1. Dict1 = “,dict1)
print(“1. Dict2 = “,dict2)
print(“1. Dict3 = “,dict3)
dict1.update({“Team”:“India”})
dict3.update({“Runs”: 12908})
dict2.update({“Wickets”: 432})
print(“2. Dict1 = “,dict1)
print(“2. Dict2 = “,dict2)
print(“2. Dict3 = “,dict3)
dict1.clear()
print(dict1)
dict1 = {}
print(type(dict1))
dict1 = {3:“Sachin”,“Second”:2,True:False,“Flag”:True}
# 3 ways – keys, values, items
for k in dict1.keys(): #keys
print(“Key = “,k,” and value is”,dict1[k])
for values in dict1.values():
print(“Value is”,values)
# iterate through items:
for item in dict1.items():
print(item,“: key is”,item[0],“and value is”,item[1])
# only a dictionary can be added to a dictionary
temp = {“City”:“Mumbai”}
dict1.update(temp)
print(“Dictionary 1: “,dict1)
#dictionary – mutable [list is mutable, tuple and string – immutable]
dict1[3]=“Tendulkar”
print(“Dictionary 1: “,dict1)
#copy
dict2 = dict1
dict3 = dict1.copy()
print(“1. Dict1 = “,dict1)
print(“1. Dict2 = “,dict2)
print(“1. Dict3 = “,dict3)
dict1.update({“Team”:“India”})
dict3.update({“Runs”: 12908})
dict2.update({“Wickets”: 432})
print(“2. Dict1 = “,dict1)
print(“2. Dict2 = “,dict2)
print(“2. Dict3 = “,dict3)
dict1.clear()
print(dict1)