Lecture Notes – I
text1 = 'Hello' \
'World'
text2 = "hi there"
text3 = '''How are you
where are you
what do you want?'''
text4 = """I am fine"""
#I am just trying to run few programs
print(text1,end=' ') #printing first text print("Hello")
print(text2)
print(text3)
print(text4)
'''this is a
multi line text
please ignore'''
print(type(True))
cost = 60
quantity = 212
total_cost = cost * quantity
print("Cost of each pen is $",cost,"so for ",quantity,"pens, its going to be $",total_cost)
print(f"Cost of each pen is $ {cost} so for {quantity} pens, its going to be $ {total_cost}")
print("Hellon\nhow are you?\nI am fine")
txt1 = "Hello"
a1 = 5
b1 = False
#user input- will provide the value
inp1 = input("Enter your name:")
print(inp1)
inp2 = int(input("Enter your age:"))
print(type(inp2))
print(inp2)
num1 = 10
num2 = 20
#ArithmeticOperations
print(num1 + num2)
print(num1 - num2)
print(num1 * num2)
print(num1 / num2)
print(num1 // num2) #integer division - ignores the decimal part
print(num1 ** num2) #power - exponent
print(num1 % num2) #mod (modulus) - remainder
#Comparison operators: < > <= >= == !=
# :input is anything but output is always bool value
#Logical operations: and or not
# :both input and output are bool value
#comparison operators - output is always Bool
## > < >= <= == !=
num1 = 56
num2 = 56
num3 = 57
print(num1 <= num2) # less than or equal - T
print(num1 <= num3) # T
print(num1 < num2) # F
print(num1 >= num2) #T
print(num1 > num2) #F
print(num1 > num3) #F
print(num1 == num2) #T
print(num1 != num2) #F
#Logical operators
# Today we will do operators and conditions
# I do only operators
#
# Today we will do operators or conditions
# I do only operators
# and or not
num1 = 56
num2 = 56
num3 = 57
print(num1 <= num2 and num1 <= num3 or num1 < num2 and num1 >= num2 or num1 > num2 and num1 > num3 or num1 == num2 and num1 != num2)
#T
#
print()
num1 = int(input("Enter a number: "))
print(type(num1))
#
# 1. Calculate area and perimeter of a rectangle with given sides
# 2. Calculate area and circumference of a circle with radius as input from the user
#conditional statements
num1 = -56
#ask you is it a positive number ?
if num1>0:
print("hello")
print("Yes its positive")
elif num1 <0:
print("Its negative")
else:
print("Its neither positive nor negative")
avg = 76
'''
avg>85 : Grade A
avg>70: Grade B
:avg >60: Grade C
avg >40: Grade D
<40: Grade E
'''
if avg >=85:
print("Grade A")
if avg >= 90:
print("You win special certificate")
if avg>95:
print("You get President's meddal")
elif avg>70:
print("Grade B")
elif avg>60:
print("Grade C")
elif avg>40:
print("Grade D")
else:
print("Grade E")
#get input of 3 numbers and find the largest and the smallest number
#loops - Keep repeating
# FOR - when you know how many times
# WHILE - untill the condition is true
#range(a,b,c): start = a, go upto b (excluding), c = increment
#range(3,19,4) = 3, 7, 11,15,
##range(3,9) (increment by default 1) - 3, 4,5,6,7,8
## range(4) (by default start=0, increment = 1)- 0,1,2,3
for i in range(5):
print("*",end=" ")
print("\n -------------- \n\n")
'''
* * * * *
* * * * *
* * * * *
* * * * *
* * * * *
'''
for j in range(5):
for i in range(5):
print("*",end=" ")
print()
print("\n -------------- \n\n")
'''
* * * * *
* * * * *
* * * * *
* * * * *
* * * * *
'''
for j in range(5):
for i in range(5):
print("*",end=" ")
print()
print("\n -------------- \n\n")
'''
*
* *
* * *
* * * *
* * * * *
'''
for j in range(5):
for i in range(j+1):
print("*",end=" ")
print()
print("\n -------------- \n\n")
'''
* * * * *
* * * *
* * *
* *
*
'''
for j in range(5):
for i in range(5-j):
print("*",end=" ")
print()
print("\n -------------- \n\n")
'''
* * * * *
* * * *
* * *
* *
*
'''
for j in range(5):
for i in range(j):
print("",end=" ")
for i in range(5-j):
print("*",end=" ")
print()
'''
*
* *
* ** *
* *
'''
sum=0
while True:
num1 = int(input("Enter a number (-999 to stop):"))
if num1==-999:
break #throw us out of the current loop
sum+=num1
print("Sum of the given numbers are: ",sum)
## check if prime number
while True:
num1 = int(input("Enter a number (0 to stop/negative numbers not allowed): "))
if num1 == 0:
break
if num1<0:
print("Invalid number")
continue
isPrime = True
for i in range(2,num1//2+1):
if num1 % i==0:
isPrime=False
break
if isPrime:
print(f"{num1} is a Prime number")
else:
print(f"{num1} is not a Prime number")
## generate if prime number
num1=0
while True:
#num1 = int(input("Enter a number (0 to stop/negative numbers not allowed): "))
num1+=1
if num1 == 0:
break
if num1<0:
print("Invalid number")
continue
isPrime = True
for i in range(2,num1//2+1):
if num1 % i==0:
isPrime=False
break
if isPrime:
print(f"{num1}")
ch=bool(input())
if ch!=False:
break
#read the marks of 5 subjects and print total and average
total = 0
while True:
for i in range(5):
marks = int(input("Enter the marks in subject "+str(i+1)+": "))
total+=marks
print(f"Total marks is {total} and average is {total/5:.2f}")
ch=bool(input())
if ch:
break
### Enter marks for 5 subjects for as long as user wants to repeat
while True:
sum = 0
for i in range(5):
marks = int(input("Enter your marks in subject "+str(i+1)+": "))
sum+=marks
print("Total marks obtained = ",sum)
ch=bool(input("Enter any key to stop:"))
#converting empty string to bool will result in
# False, anything will result in True
if ch:
break
########################
####
str1 = 'HELLO'
str2 = "I am fine"
str3 = '''Where are you going?
How long will you be here?
What are you going to do?'''
str4 = """I am here
I will be here for next 7 days
I am going to just relax and chill"""
print(type(str1),type(str2),type(str3),type(str4))
print(str1)
print(str2)
print(str3)
print(str4)
# What's you name?
str5 = "What's your name?"
print(str5)
#He asked,"Where are you?"
str6 = 'He asked,"Where are you?"'
print(str6)
#He asked,"What's your name?"
#escape sequence \
print('''He asked,"What's your name?"''')
print("He asked,\"What's your name?\"")
print('nnnnn\nnn\tnn')
print("\FOlder\\newfolder")
# \n is used to print newline in python
print("\\n is used to print newline in python")
# \\n will not print newline in python
print("\\\\n will not print newline in python")
str1 = "Hello You"
str2 = "There"
print(str1 + str2)
print(str1 *5)
for i in str1:
print("Hello")
#indexing
print(str1[2])
print("last element: ",str1[4])
print("last element: ",str1[-1])
print("second element: ",str1[-8])
print("ell: ",str1[1:4])
print("ell: ",str1[-8:-5])
print("First 3: ",str1[:3])
print("First 3: ",str1[:-6])
print("Last 3: ",str1[6:])
print("Last 3: ",str1[-3:])
#Methods - exactly same as your functions - only difference is they are linked to a class
import time
str1 = "HELLO"
print(str1.replace("L","X",1))
sub_str = "LL"
str2 = "HELLO HOW WELL ARE YOU LL"
cnt = str2.find(sub_str)
print("Count = ",cnt)
if cnt<0:
print("Sorry, no matching value hence removing")
else:
print("Value found, now replacing")
for i in range(5):
print(". ",end="")
time.sleep(0.5)
print("\n")
print(str2.replace(sub_str,"OOOO"))
out_res = str2.split("LL")
print("Output Result = ",out_res)
out_str = "LL".join(out_res)
print(out_str)
print(str2.title())
print(str2.lower())
print(str2.upper())
str3 = 'hello how well are you ll'
print(str3.islower())
print(str3.isupper())
num1 = input("Enter a number: ")
if num1.isdigit():
num1 = int(num1)
else:
print("Invaid input")
ename = input("Enter your first name: ")
if ename.isalpha():
print("Your name is being saved...")
else:
print("Invaid name")
#WAP to count of vowels in a sentence
para1 = "Work, family, and endless to-do lists can make it tough to find the time to catch up. But you'll never regret taking a break to chat with your friend, Frost reminds us. Everything else will still be there later."
sum=0
for l in para1:
if l=='a' or l=='A' or l=='e' or l=='E' or l=='i' or l=='I' or l=='o' or l=='O' or l=='u' or l=='3':
sum+=1
print("Total vowesl = ",sum)
sum=0
for l in para1.lower():
if l=='a' or l=='e' or l=='i' or l=='o' or l=='u':
sum+=1
print("Total vowesl = ",sum)
sum=0
for l in para1.lower():
if l in 'aeiou':
sum+=1
print("Total vowesl = ",sum)
########## LIST
#LIST
#collection of linear ordered items
list1 = [1,2,3,4,5]
print(type(list1))
print("Size = ",len(list1))
print(list1[0])
print(list1[-1])
print(list1[3])
print(list1[:3])
print(list1[-3:])
print(list1[1:4])
for i in list1:
print(i)
print([2,3,4]+[6,4,9])
print([2,3,4]*3)
str2 = "A B C D A B C A B A "
print(str2.count("D"))
print(list1.count(3))
l1 = [2,4,6,8]
print(l1.append(12))
print(l1)
l1[0]=10
print(l1)
l1.insert(2,15)
print(l1)
# Queue: FIFO
# Stack: LIFO
if 16 in l1:
l1.remove(16) #takes in value to remove
l1.remove(15)
print(l1)
l1.pop(1) #index
print(l1)
#################
while False:
print("Queue is: ",l1)
print("1. Add\n2. Remove\n3. Exit")
ch=input("Enter your choice: ")
if ch=="1":
val = input("Enter the value: ")
l1.append(val)
elif ch=="2":
l1.pop(0)
elif ch=="3":
break
else:
print("Try again!")
while False:
print("Stack is: ",l1)
print("1. Add\n2. Remove\n3. Exit")
ch=input("Enter your choice: ")
if ch=="1":
val = input("Enter the value: ")
l1.append(val)
elif ch=="2":
l1.pop(-1)
elif ch=="3":
break
else:
print("Try again!")
l2 = l1 #they become same
l3 = l1.copy()
print("1. List1 = ",l1)
print("1. List2 = ",l2)
print("1. List3 = ",l3)
l1.append(33)
l2.append(44)
l3.append(55)
print("2. List1 = ",l1)
print("2. List2 = ",l2)
print("2. List3 = ",l3)
l1.extend(l3)
print(l1)
print(l1.count(6))
sum=0
marks=[]
for i in range(3):
m = int(input("Enter marks in subject "+str(i+1)+": "))
marks.append(m)
sum+=m
print("Sum is ",sum, "and average is ",sum/3)
print("Marks obtained is ",marks)
#THREE STUDENTS AND THREE SUBJECTS:
allmarks=[]
for j in range(3):
sum=0
marks=[]
for i in range(3):
m = int(input("Enter marks in subject "+str(i+1)+": "))
marks.append(m)
sum+=m
print("Sum is ",sum, "and average is ",sum/3)
print("Marks obtained is ",marks)
allmarks.append(marks)
print("All the marks are: ",allmarks)
# All the marks are: [[88, 66, 77], [99, 44, 66], [44, 99, 88]]
# find the highest marks of each subject
#
num = 51
isPrime = True
for i in range(2,num):
if num%i==0:
isPrime = False
break
if isPrime:
print("Its a Prime number")
else:
print("Its not a prime number")
###
start1,end1= 500,5000
for j in range(start1,end1+1):
isPrime = True
for i in range(2, j):
if j % i == 0:
isPrime = False
break
if isPrime:
print(j,end=", ")
# while loop
#While loop
i=1
while i<=10:
print(i)
i+=1
ch=True
while ch:
num=int(input("Enter a number: "))
if num%2==0:
print("Its an even number!")
else:
print("Its an odd number")
choice=input("Do you want to continue (press n to stop):")
if choice=='n':
ch=False
while True:
num=int(input("Enter a number: "))
if num%2==0:
print("Its an even number!")
else:
print("Its an odd number")
choice=input("Do you want to continue (press n to stop):")
if choice=='n':
break
import random
n=1
total_attempts = 0
max=0
min=99999999
for i in range(n):
comp_num = random.randint(1,100)
print("Random: ",comp_num)
attempt = 0
while True:
guess = int(input("Guess the number: "))
#guess = random.randint(1, 100)
attempt+=1
if guess == comp_num:
print("Congratulations! You have guessed it correctly. Attempts = ",attempt)
total_attempts+=attempt
if attempt>max:
max=attempt
if attempt <min:
min=attempt
break
elif comp_num > guess:
print("Sorry! You have guessed low!")
else:
print("Sorry! You have guessed high")
print("Avg attempts = ",total_attempts/n)
print("Max attempts =",max,"and Minimum attempts = ",min)
'''
Avg attempts = 99.384
Max attempts = 529 and Minimum attempts = 1
'''
#######
import random
n=500
total_attempts = 0
max=0
min=99999999
for i in range(n):
comp_num = random.randint(1,100)
print("Random: ",comp_num)
attempt = 0
low,high=1,100
while True:
#guess = int(input("Guess the number: "))
guess = random.randint(low, high)
attempt+=1
if guess == comp_num:
print("Congratulations! You have guessed it correctly. Attempts = ",attempt)
total_attempts+=attempt
if attempt>max:
max=attempt
if attempt <min:
min=attempt
break
elif comp_num > guess:
print("Sorry! You have guessed low!")
low= guess+1
else:
print("Sorry! You have guessed high")
high=guess-1
print("Avg attempts = ",total_attempts/n)
print("Max attempts =",max,"and Minimum attempts = ",min)
'''
Avg attempts = 7.522
Max attempts = 15 and Minimum attempts = 1
'''
txt1 = "HELLO"
txt2 = 'How are yOU?'
# what's your name?
print("what's your name?")
print('He asked,"Where are you?"')
#He asked,"What's your name?"
print('''He asked,"What's your name?"''')
print("He asked,\"What's your name?\"")
txt3 = '''How are you?
Where are you?
what do you want?'''
txt4 = """I am fine
I am here
I want nothing"""
print(txt4)
for ch in txt1:
print(ch)
print(txt1+" "+txt2)
print(txt1*10)
#indexing
print(txt1[0])
print(txt1[4])
length = len(txt1)
print("Total characters = ", length)
print(txt1[length-1])
print(txt1[-1])
print(txt1[length-2])
print(txt1[length-3])
print(txt1[-3])
print(txt1[0]+txt1[1]+txt1[2])
print(txt1[0:3])
print(txt1[0:4])
print(txt1[1:4])
print(txt1[2:5])
print(txt1[-3:])
print(txt1[:])
#METHODS ARE INBULT FUNCTIONS OF A CLASS
print(txt1.lower())
print(txt2.upper())
#String - class
# functions specific to classes are called methods
str1 = "O I see I am fine see you are NICE"
str2 = "hello hi"
print(str1.islower())
print(str2.islower())
str3 = str2.upper()
print(str3)
print(str3.isupper())
num1 = input("Enter a number: ")
if num1.isdigit():
num1=int(num1)
else:
print("Invalid number as input, setting it it zero")
num1 = 0
print("Num1 = ",num1)
#create username with only alphabets and numbers
username = input("Enter your username: ")
if username.isalnum():
print("Username accepted")
else:
print("Invalid Username!")
print(str1.upper())
print(str1.lower())
print(str1.title())
str1 = "O I see I am fine I see I you are NICE"
print("i" in str1)
print(str1.find('I'))
print(str1.count('I'))
print(str1.find('I',3))
print(str1.find('I',9))
char = "ee"
num_pos = str1.count(char)
print("Total times char is present = ",num_pos)
print("Their positions are: ",end=" ")
start_pos = 0
for i in range(num_pos):
nxt_val = str1.find(char,start_pos)
print(nxt_val,end= " , ")
start_pos = nxt_val+1
print(str1.replace("ee","EE"))
words = str1.split("I")
print(words)
str6 = "I".join(words)
print(str6)
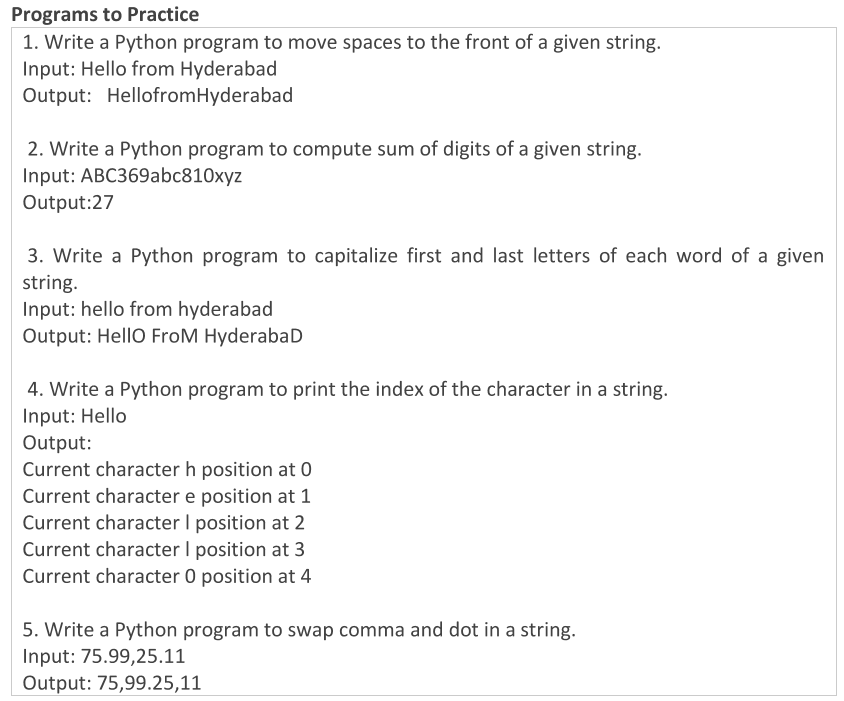
#LIST
l1 = [5,4,"Hello",99,False,100,[20,10, 5]]
print(type(l1))
print(len(l1))
l2 =l1[-1]
print(l2[1])
print(l1[-1][2])
print(l1[1:4]) #4, "hello" 99
print("Only Integer values fom List")
for i in l1:
if str(i).isdigit():
print(i)
print(l1+l2)
print(l2*5)
list1 = [2,4,6,8,10]
list1.insert(2,33)
list1.remove(10) # remove asks
print(list1)
# let's simulate Queue behavior: FIFO
list2 = []
while True:
print("1. Add Value to the Stack")
print("2. Remove Value from the Stack")
print("3. Exit from the Stack")
ch=input("Enter the operation you want to perform: ")
if ch=="1":
value = input("Enter the member to be added: ")
list2.append(value)
print("Printing the stack:",list2)
elif ch=="2":
list2.pop(0) # position is entered
print("Printing the stack:", list2)
elif ch=="3":
print("Removing all the values from the queue and terminating it!")
list2.clear()
break
else:
print("Invalid options")
#STACK - FILO
list2 = []
while True:
print("1. Add Value to the Queue")
print("2. Remove Value from the Queue")
print("3. Exit from the queue")
ch=input("Enter the operation you want to perform: ")
if ch=="1":
value = input("Enter the member to be added: ")
list2.append(value)
print("Printing the queue:",list2)
elif ch=="2":
list2.pop(0)
print("Printing the queue:", list2)
elif ch=="3":
print("Removing all the values from the queue and terminating it!")
list2.clear()
break
else:
print("Invalid options")
#WAP to input marks in 3 subjects for 3 students
# [[1,2,3], [10, 20,30], [5,0,15]]
num_students = 3
num_subjects = 3
all_data=[]
for i in range(num_students):
t_list = []
for j in range(num_subjects):
val = input("Enter the marks in subject "+str(j+1)+" and student "+str(i+1)+": ")
t_list.append(val)
all_data.append(t_list)
print("Display all data: ",all_data)
l1 = [1,3,6,5,7,7,7]
#List is mutable
l1[2]=50
print(l1)
count = l1.count(7)
print("Count = ", count)
print("Index = ", l1.index(7))
#deep copy v shallow copy
print("1. Before")
print(l1)
l2 = l1 # deep copy
l3 = l1.copy()#shallow copy
print("1. AFTER")
print("Values in Chapter 1 and Room 1:", l1)
print("Values in Chapter 1 and Room 1:", l2)
print("Values in Chapter 1 and Room 1:", l3)
l1.append(50)
l3.insert(100,200)
l2. insert(45,20)
print("2. AFTER")
print("Values in Chapter 1 and Room 1:", l1)
print("Values in Chapter 1 and Room 1:", l2)
print("Values in Chapter 1 and Room 1:", l3)
l1 = [10,40,60,20,40,80]
l1.sort()
print("Sort: ",l1)
l1.reverse()
print("Reverse: ",l1)
l3=[3]
l3.extend(l1) # l3 = l3 + l1
print(l3)
#l3 = l1 +l2
#Assignment: Read marks in 3 subjects for 3 students and find the highest marks in each subject
START PLANNING ON BELOW PROJECT WORK
Ignore the libraries to be used instructions above, instead, use Connecting to Database and save and retrive data from the database. You can use SQLITE3 or MYSQL or any database of your choice. You need to implement all the features mentioned.
#TUPLE - immutabe form of List
t1 = (2,4,6,8,10,12,14,16,18,20) #packing
print(type(t1))
print(t1[4])
t2=()
print(type(t2))
t3=(3,)
print(type(t3))
for i in t1:
print(i, end=" , ")
print()
t1 = list(t1)
print(type(t1))
t1.append(99)
t1=tuple(t1)
t2 = (3,6,9,12)
a,b,c,s = t2
print(a)
t4=(5,99)
t5=(5,99)
if t4>t5:
print(t4, "greater")
elif t5>t4:
print(t5, "greater")
else:
print("Both are same")
##Dictionary: there is no defaut order
# each member has its own key
dict1 ={"Name": "Rohit","Sport":"Cricket",1:"Captain",1:100}
print(type(dict1))
print(dict1['Name'])
print(dict1[1])
#Immutable: String, Tuple
#Mutable: List, Dictuinary, Sets
t_dict = {'City':'Mumbai'}
dict1.update(t_dict)
print(dict1)
dict2 = dict1
dict3 = dict1.copy()
print("1. Dictionaries")
print(dict1)
print(dict2)
print(dict3)
dict1.pop(1)
dict3.popitem()
print("2. Dictionaries")
print(dict1)
print(dict2)
print(dict3)
print("Items (Key,value): ",dict1.items())
print("Values: ",dict1.values())
print("Keys: ",dict1.keys())
dict1.clear()
print(dict1)
marks_dict={}
for i in range(2):
name1 = input("Enter the students name: ")
t_list = []
for j in range(3):
m=int(input("Enter marks: "))
t_list.append(m)
marks_dict.update({name1:t_list})
print("Marks details: ")
for i,j in marks_dict.items():
print(f"{i} obtained marks: {j}")
list_temp= ['Maths','Science','Social Studies']
all_marks = {}
student1 = {}.fromkeys(list_temp,-9)
all_marks.update(student1)
print("All marks: \n",all_marks)
for i in all_marks.keys():
marks = int(input("Enter the marks for "+i+" : "))
all_marks[i]=marks
print("All marks are: ",all_marks)
#Basic data types
val1 = 5 #int
print(type(val1))
val1 = '5' #str
print(type(val1))
val1 = 5.0 #float
print(type(val1))
val1 = True #bool - True / False
print(type(val1))
val1 = 5j #complex - j (square root of -1)
print(type(val1))
val1 = 4
## comparison operators - takes num as input but gives bool as output
## logical operators: take bool value as input and gives bool as output
# AND: All the values have to be True to be True else its False
# True and True = True
# T and T and T and T and T and T and F = False
# OR: All the values have to be False to be False otherwise its True
## True or False = True
## control structure with logical operators, you mean multiple conditions
val1 = 185
if val1>0 and val1 <100:
print("Value is between 0 and 100")
## Basic graphics, sets, functions,
list1 = [5, 5,5,8.3, False,"Hello",[1,6,9]]
tup1 = (5, 8.3, False,"Hello",[1,6,9]) #list and tuple have auto index: 0...
dict1 = {5:"Hello","Name":"Sachin"}
# SET -
set1 = {"Apple","Banana"}
print(type(set1))
print(set1)
# sets - union, intersection, difference, symmetric difference
set1 = {2,4,6,8}
set2 = {1,3,5,7,6,8}
# union - it puts all the values together
set3 = set1.union(set2)
print("Set 3 = ", set3)
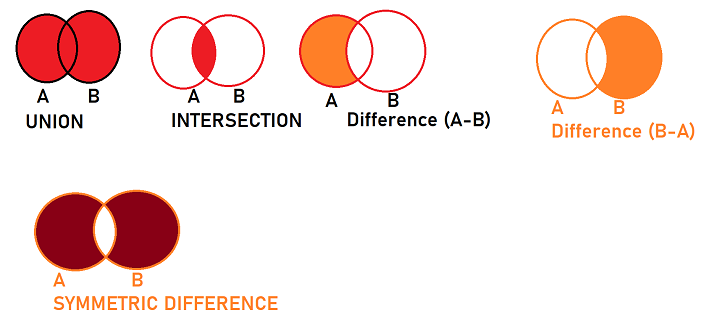
#SETS
set1 = {1,2,3,10,20}
set2 = {1,2,3, 50,60}
print("UNION: ")#union
set3 = set1.union(set2)
print(set3)
print(set1 | set2)
print("INTERSECTION: ")#
set3 = set1.intersection(set2)
print(set3)
print(set1 & set2)
print("DIFFERENCE: Set1 - Set2")#
set3 = set1.difference(set2)
print(set3)
print(set1 - set2)
print("DIFFERENCE: Set2 - Set1")#
set3 = set2.difference(set1)
print(set3)
print(set2 - set1)
print("SYMMETRIC DIFFERENCE")#
set3 = set2.symmetric_difference(set1)
print(set3)
print(set2 ^ set1)
#### update, difference_update, intersection_update, symmetric_difference_update)
#without update, the operations result in new sets but with update, existing set is updated
### Functions - giving a name to a block of code
def mystatements(): # user defined functions
print("Whats your name?")
print("Where are you going?")
print("How are things?")
mystatements()
mystatements()
mystatements()
#below is an example of udf which doesnt take any argument nor does it return any value
def add_2_num():
num1 = input("Enter a number: ")
num2 = input("Enter another number: ")
sum = num1 + num2
print("total value is",sum)
#add_2_num()
#this is an example of function returning a value (still no arguments)
def add_2_num_ret():
num1 = input("Enter a number: ")
num2 = input("Enter another number: ")
sum = num1 + num2
#print("total value is",sum)
return sum
#result = add_2_num_ret()
#print("After return value is", result)
#example of function that takes argument
#TYPE 1 Required positional arguments
def add_2_num_arg(num1, num2):
sum = num1 + num2
#print("total value is",sum)
return sum
#TYPE 2: Default (opp of Required) positional arguments
def add_2_num_arg1(num1, num2="19"): #num2 is default
sum = num1 + num2
#print("total value is",sum)
return sum
#values are mapped from left to right
output = add_2_num_arg1("15","89")
print("Result from add_2_num_arg1: ",output)
output = add_2_num_arg1("15")
print("Result from add_2_num_arg1: ",output)
# TYPE 3: Keyword arguments (non-Positional)
output = add_2_num_arg1(num2 = "15",num1 = "89")
print("Result from add_2_num_arg1: ",output)
# TYPE 4: Variable length arguments
def myfun1(*var1, **var2):
print("Var 1 = ",var1) #Tuple
print("Var 2 = ", var2) #Dictionary
myfun1(2,4,99,"hello",False,[2,3,4],num2 = "15",num1 = "89")
#WAP to count positive numbers entered by a user
def checkPositive(*num): #variable length arguments
total=0
for i in num:
if i >0:
total+=1
return total
def checkPositive2(num): #variable length arguments
if num >0:
print("Positive")
else:
print("Not Positive")
def checkPositive3(num): # variable length arguments
if num > 0:
return 1
else:
return 0
val1 = [13,35,90,-9,0,8]
tot = checkPositive(13,35,90,-9,0,8,-8,-6,6)
print("Number of positive values = ",tot)
val2 = -9
result = checkPositive3(val2)
if result==1:
print("Number is positive")
else:
print("Number is not positive")
total = 0
val1 = [13,35,90,-9,0,8]
for i in val1:
total += checkPositive3(i)
print("Total positive values = ",total)
#######################
def myfactorial(n):
if n<=1:
return 1
else:
return n * myfactorial(n-1)
result = myfactorial(-5)
print("Factorial is ",result)
# return 8 * 7 * 6 * 5 * 4 * 3 * 2 * 1
# 2 = 2 * 1!
# 1 * 0!
val = 5
aList = [2,4,5,3,5,6,7,5]
count = 0
for item in aList:
if item==val:
count=count+1
print("Count = ",count)
'''
import random
a = random.random() # random value between 0 and 1
print(a)
b = random.randint(1,3)
print(b)
a <- 5 #assignment in APSC exam language, in Python: a =5
a = 5 # is a equal to 5? in Python a ==5
aList <- [2,4,6,8]
DISPLAY aList[1]
a <- aList[3]
aList[2] <- 10 # [2,10,6,8]
INSERT(aList, 2,10) # [2,10,4,6,8]
APPEND(aList,20) # [2,10,4,6,8,20]
REMOVE(aList,4) # [2,10,4,8,20]
LENGTH(aList) #len(aList) - python
# In Python we say:
for item in alist:
pass
#in APSC lang
FOR EACH item in aLists
{
}
def fun1(n1,n2):
return 1
PROCEDURE fun1(n1,n2)
{
RETURN (1)
}
'''