How classes and objects are used in Python
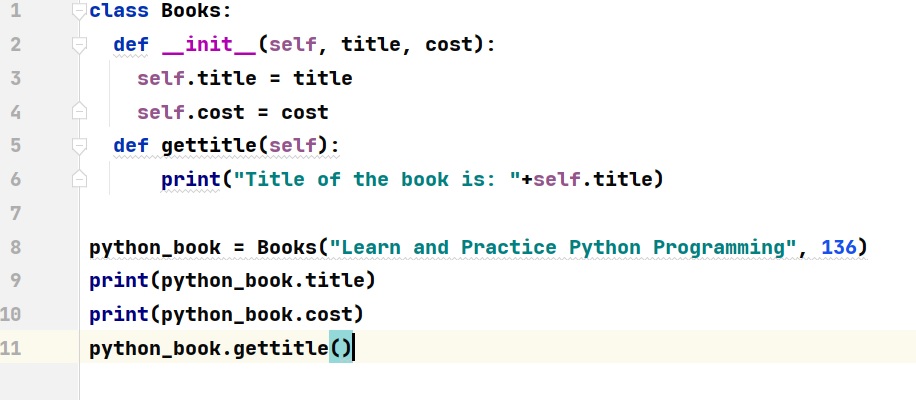
Python is said to be a pure object oriented programming language and almost everything you come across in Python is an object. A class can be defined as a user-defined type that, by having its own methods (functions) and property (variables), define what object will look like. An object is a single instance of a class. You can create many objects from the same class type.
Creating a class in Python
class keyword is used in Python to create a class, an example is shown below. We create a class named Books with a property named total_count:
class Books:
total_count = 5
Creating an object
class is just a blueprint. In order to use the class, we need to create an object. In below example, lets create an object called learn_python and using this we will print the value of total_count:
class Books:
total_count = 5
python_book = Books()
print(python_book.total_count)
The init() Function
Let’s look at an important member of the class. We are talking about init() function. Infact all classes have this function, which is always called an object of the class in being created. This function is called automatically so you dont have to specifically call this. We use the init() function to initialize values or properties that needs to be assigned to the object immediately when created. In the below example, we create a class Books and using the init() function, we have automatically assigning title and the cost to the object:
class Books:
def __init__(self, title, cost):
self.title = title
self.cost = cost
python_book = Books("Learn and Practice Python Programming", 136)
print(python_book.title)
print(python_book.cost)
Object Functions
A class will also have object functions or methods. Functions that are defined in objects, belong to object (and not to the class). It means that the objects will use these functions and the result returned will be specific to the objects. These are characterised by self keyword as first parameter being passed. Let’s add a function to our class Books which will print the title of the book. This is called object function because it knows which objects are referencing and the title value is linked to that object only.
Example:
class Books:
def __init__(self, title, cost):
self.title = title
self.cost = cost
def gettitle(self):
print("Title of the book is: "+self.title)
python_book = Books("Learn and Practice Python Programming", 136)
print(python_book.title)
print(python_book.cost)
python_book.gettitle()
The self parameter used in object functions is to show the reference to the current object associated to the class.
Please note, it does not have to be named self, we can use any name we like it but whatever name we choose, that has to be the first parameter of the object functions.
We will end our discussion here. We will develop a mini application using classes in next article.