Learning Python with Sahasra – 2023
print("HELLO")
# 1. INSTALLATION - getting your computer ready
# a. you can practice online at Google Colab (colab.reaserch.google.com)
# b. install all software on your machine -
## i. install Python : python.org and download the version of your choice
## ii. you can program on Python but its very easy to do
## iii. Another software to make it user friendly - IDE
## integrated development environment - you can practice
## eg Pycharm (jetbrains.com), VS Code , Notebooks like Jupyter, etc
print("Hello how are you?")
print('uyuyuiiugiub7y7 7t6t6vt87tovtotff87yvpyv')
print(5+11)
print('5+11=',5+11)
num1 = 5 #num1 are called variables
num2 = 11 #variable names are case sensitive, name should not start with a digit
#alphabets, numbers and underscore(_) - only these are allowed
print(num1 + num2)
var1 = 5 #let’s take a variable called var1 and make it equal to 5
# number without decimal point – integer
#var1 is a variable of type integer
print(“var1”)
print(var1)
print(type(var1))
var1 = 5.0 #float – -inf to +inf with decimal point
print(type(var1))
var1 = 5j #complex (imaginary) – i
# square root of -25 = sq rt(25* -1) = 5j
print(type(var1))
print(5j * 5j)
# string – text (str)
var1 = “hello”
print(type(var1))
#5th basic data types – bool (boolean – 2 values: True / False)
var1 = True
print(type(var1))
#Functions for explicit conversion – convert data from given type to this:
# int(), float(), complex(), str(), bool()
var1 = int(“5”)
print(type(var1))
quantity =49
cost_pc = 51.89
total_cost = quantity * cost_pc
print(“Total quantity bought is”,quantity,”each one at a cost of”,cost_pc,”so the total cost is”,total_cost)
print(f”Total quantity bought is {quantity} each one at a cost of {cost_pc:.1f} so the total cost is {total_cost}”)
pl=”Rohit”
cn=”India”
pos=”captain”
print(f”Player {pl:<12} plays for {cn:^12} and is {pos:>20} of the team!”)
pl=”Mabwange”
cn=”Zimbabwe”
pos=”Wicket-keeper”
print(f”Player {pl:<12} plays for {cn:^12} and is {pos:>20} of the team!”)
num1 = int(input(“Enter first number to be added: “)) #default input will read as str
print(type(num1))
num2 = int(input(“Enter second number to be added: “))
print(type(num2))
print(num1+num2)
var1 = 5
var2 = 5.0
var3 = "Hello"
var4 = True
var5 = 5j
#arithmetic operations
var1 = 15
var2 = 4
print(var1 + var2) #8
print(var1 - var2) #
print(var1 * var2)
print(var1 / var2)
print(var1 // var2) #integer divison, the integer part of the divison
print(var1 % var2) #modulo - remainder
print(var1 ** var2)
# a,b = 30,6 30 % 20
#Comparison operators: is ?? and answer will be bool
var1 = 15
var2 = 4
var3 = 15
print(var1 > var2)
print(var1 >= var3) #is it greater than or equal to
print(var1 < var2)
print(var2 <= var3)
print(var1 == var2)
print(var1 != var2)
#logical operators: input and output both are bool
#and, or, not
print(var1 == var2 and var1 != var2)
print(False and False) #False
print(False and True) #False
print(True and False) #False
print(True and True) # True
print(False or False) #False
print(False or True) #True
print(True or False) #True
print(True or True) # True
var1 = 15
var2 = 4
var3 = 15
print(var1 > var2 and var1 >= var3 or var1 < var2 or var2 <= var3 and var1 == var2 and var1 != var2)
#T or F
print("Hello \nHow are you \nI am fine thank you")
# \n is for newline
print("\\n is for newline")
print("\\\\n is for newline")
print("Hello",end=" ")
print("How are you",end=" ")
print("I am fine thank you",end="\n")
#Conditional statements
num1=0
if num1 > 0:
print("Positive")
print("This is a positive number")
print("my 3rd line for positive number")
elif num1 < 0:
print("Negative")
print("This is a negative number")
print("my 3rd line for negative number")
else:
print("Its neither positive or nagtive")
print("Thank you for using IF")
num1 = int(input("Enter a number to be tested: "))
if num1 %3==0:
print("Its divisible by 3")
else:
print("Its not divisible by 3")
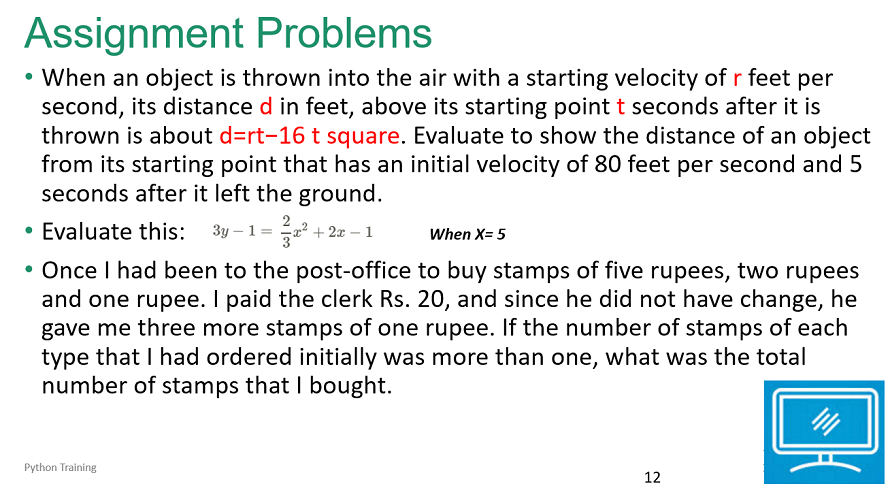
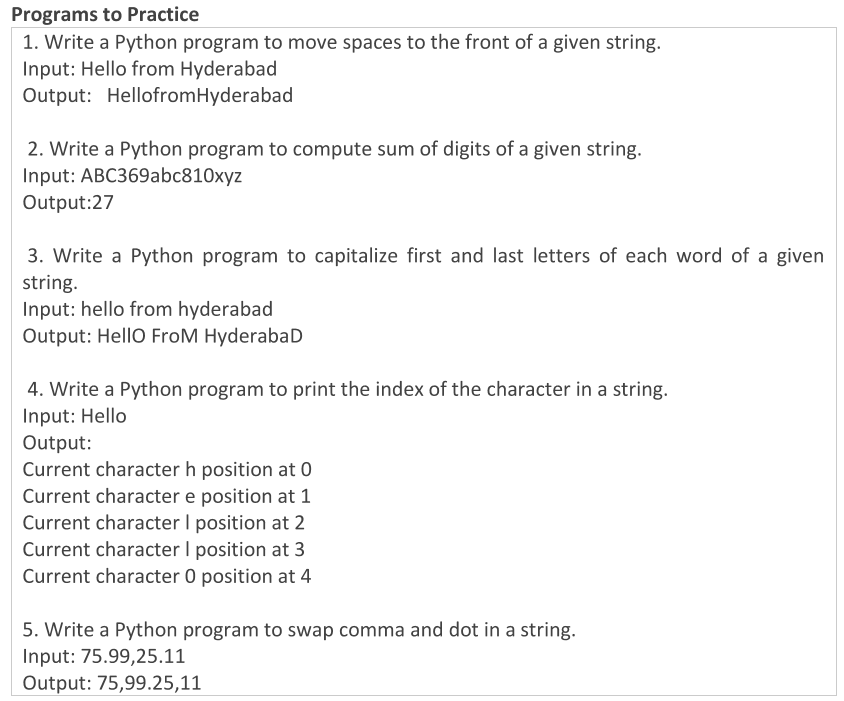
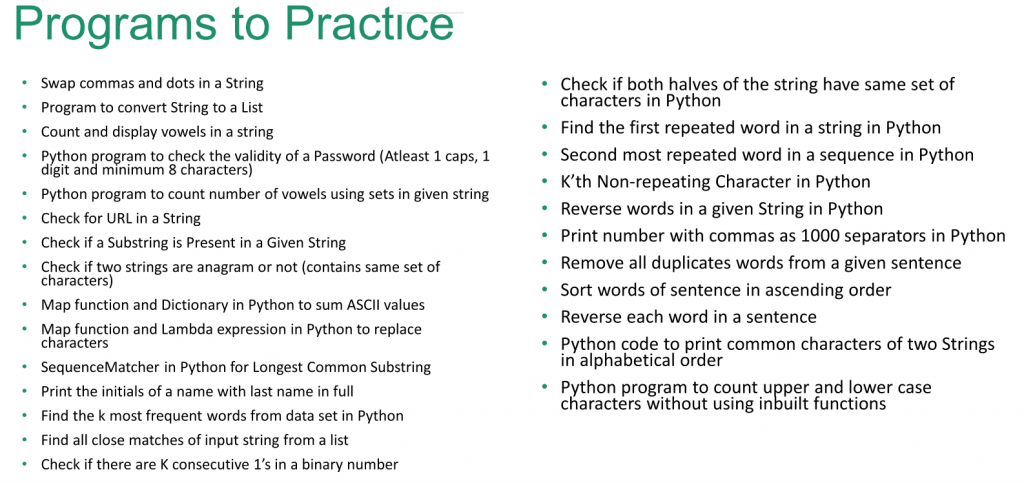
avg = 89
if avg>=50:
print("Result: Pass")
#85: Grade A, 75> Grade B, >60: C, > 50-D
if avg>=85:
print("Grade: A")
if avg>=90:
print("Please collect your merit certificate!")
if avg>=95:
print("You win President's medal")
elif avg>=75:
print("Grade: B")
elif avg>=60:
print("Grade: C")
else:
print("Grade: D")
else:
print("Result: Fail")
# a & b=> which is greater
a=45
b=95
if a>b:
print("A is greater than B")
elif b>a:
print("B is greater than A")
else:
print("They are equal")
#Assignment: take three numbers a,b,c and arrange them in increasing order without using any inbuilt function.
[Hint: Build on the last example shared]
#INPUT: a,b,c=20,60,30
#OUPUT: 20,30,60 (increasing order)
a,b,c=80,60,30
# a>b, b>c => clear
# a>b, c>b => wrote additional code to find
#b>a,
a, b, c=50,30,30 #increasing order
if a<b:
#print (f"{b} {a}")
if c<b: #b is greatest
if a<c:
print(f"{a}, {c}, {b}")
else:
print(f"{c}, {a}, {b}")
else: #c is greatest
print (f"{a}, {b}, {c}")
else: #b<a
if c<b: #b is greatest
print(f"{c}, {b}, {a}")
else: #b is lowest
if a<c:
print (f"{b}, {a}, {c}")
else:
print(f"{b}, {c}, {a}")
# 30<50=50
# LOOPS - repeation
#FOR loop - when we know exactly how many times to execute
### Range(a,b,c): generates values from a (inclusive) to b (exclusive) at steps of c
# range(2,12,3): 2,5,8,11
# range(3,12,3): 3,6,9
# range(6,10): a&b. c is default 1:
#range(5): b & a,c are deafult 0 and 1 respectively => 0,1,2,3,4
for i in range(5):
print("Hello",i)
for i in range(3,12,3):
print("Hi there", i)
for i in range(2,12,3):
print("Hello there", i)
print()
for i in range(5):
print("*",end=" ")
print()
'''
* * * * *
* * * * *
* * * * *
* * * * *
* * * * *
'''
for j in range(5):
for i in range(5):
print("*",end=" ")
print()
#While Loop = based on condition- executes till its True
#print("Hello")
# loops - for & while
## For loop is used when you know exactly how many times to run the loop
for i in range(2,10,2):
print("* ",i)
print("-------------")
'''
* * * * *
* * * * *
* * * * *
* * * * *
* * * * *
'''
for j in range(5):
for i in range(5):
print("*",end=" ")
print()
print("-------------")
for i in range(5):
print("* "*5)
print("-------------")
'''
*
* *
* * *
* * * *
* * * * *
'''
for j in range(5):
for i in range(j+1):
print("*",end=" ")
print()
print("-------------")
'''
* * * * *
* * * *
* * *
* *
*
'''
for j in range(5):
for i in range(5-j):
print("*",end=" ")
print()
print("-------------")
'''
* * * * *
* * * *
* * *
* *
*
'''
for j in range(5):
for x in range(j):
print(" ",end="")
for i in range(5-j):
print("*",end=" ")
print()
print("--- Assignment ----------")
'''
*
* *
* * *
* * * *
* * * * *
'''
#WHILE
i=0
while i<5:
print("hello")
i+=1
print("Thank you")
cont='y'
while cont=='y' or cont=='Y':
print("Ok, printing some values...")
cont = input("Do you want to continue? (y for yes)")
cont='y'
while cont=='y' or cont=='Y':
sum=0
for i in range(3):
marks = int(input("Enter the marks: "))
sum+=marks
print("Total marks is",sum,"and average is",sum/3)
cont = input("Do you want to continue? (y for yes)")
import turtle
#screen = turtle.getscreen()
plot = turtle.Turtle() #creates screen
screen = turtle.Screen()
turtle.Screen().bgcolor("yellow")
plot.forward(100)
turtle.color("red")
plot.right(90)
plot.forward(100)
plot.right(90)
plot.forward(100)
plot.right(90)
plot.forward(100)
##
plot.right(45)
plot.forward(100)
plot.right(45)
plot.forward(100)
plot.right(135)
plot.forward(100)
plot.right(45)
plot.forward(100)
plot.backward(100)
plot.left(45)
plot.backward(100)
plot.left(45)
plot.forward(100)
plot.right(45)
plot.forward(100)
## circle
plot.circle(50)
width = screen.window_width()
height = screen.window_height()
plot.penup()
plot.goto(width/2,height/2)
plot.pendown()
plot.dot(30,"red")
turtle.mainloop() #keep displaying the screen
# Forward, Backward, Left, Right
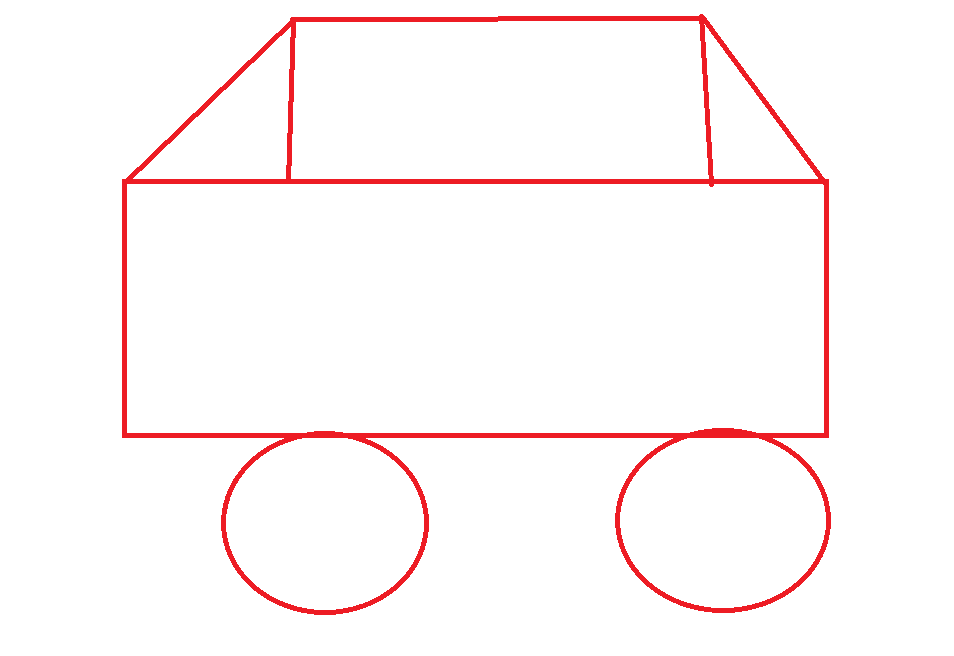
#When the value is = to 16 and you want to add 5, you add 5 units to 16
num1 = 18
num2 = 5
num3 = num1 + num2
num4 = num1 * num2
num5 = num1 / num2
num6 = num1 - num2
# 3 square + 4 square + 5 square + 6 square + 7 square
num1 = 3
num2 = 4
num3 = 5
num4 = 6
num5 = 7
result = num1 * num1 + num2*num2 + num3*num3 + num4*num4 + num5*num5
print ("Final result = ",result)
#read 2 values from the user and display val1 square + val2 square
val1 = input("Enter val1: ")
val1 = int(val1)
val2 = int(input("Enter val2: "))
#when you read using input by default values are of type string
print("Type = ", type(val1))
print("Result=", val1*val1+val2*val2)
#solve: 2x.x + 9x - 5 = 0
# 1. Calculate Descriminant: b.b - 4.a.c = 81+40=121
# 2. Root of D = 121 ** 0.5 = +/- 11
# 3. x = (-b +/-D) / 2.a = (a) (-9+11)/4 = 0.5 (b) (-9-11)/4 = -5
print("Solving a quadratic equation for x:")
a=4
b=2
c=-6
D = b**2 - 4*a*c
print("Finding Discriminant = ",D)
if D==0:
print("This equation has only 1 solution and x = ",(-b/(2*a)))
elif D<0:
print("This equation has imaginary solution")
else:
sol1 = (-b+D**0.5)/(2*a)
sol2 = (-b - D ** 0.5) / (2 * a)
print(f"Solutions are: {sol1} and {sol2}")
## x.x + 4.5x - 2.5 = 0 / (x+p)(x+q): p.q = -2.5 and p+q = 4.5
#If the number given divides into 2 whole numbers, then it is even. If it is divided by two and it is a decimal or fraction, then it is odd.
#If the number is divided by 5 and the value is a whole number, than it is a multiple of 5
# if <> - elif <> - else
num = -35
if num>0:
print("Its positive")
print("Its inside num > 0 condition")
else:
print("Its not positive")
print("What else")
print("This is another statement")
# In a block (only IF, IF-ELSE or IF-ELIF-ELSE)
num2 = -10
if num2==0:
print("Its zero")
elif num2<0:
print("Its negative")
else:
print("Its positive")
# WAP to check if a number is odd or even (I will do now)
#Modify the above program to give a message is number is less than 0
# Msg - "Invalid number
num3 = -11
if num3 %2==0:
print("Its even number")
else:
print("Its odd number")
if num >0:
if num%2==0:
print(“Its positive and even”)
if num%4==0:
print(“Number is divisible by 4 also”)
else:
print(“Its negative and odd”)
elif num<0:
print(“Its negative”)
else:
print(“Neither positive nor negative”)
#loops
# for loop
#range(start, stop, increment)
#range(5,25,5) = 5,10,15,20
#counter = 51
#print(counter in range(5,25,5))
for counter in range(5,25,5):
print(counter)
##range(5,10) = (start=5,end=10, default increment=1) 5,6,7,8,9
#range(5) = (default start=0, end=5, default increment=1) = 0,1,2,3,4
num=10
for j in range(num):
for i in range(num):
print(“*”,end=” “)
print()
for j in range(num):
for i in range(j+1):
print(“*”,end=” “)
print()
for j in range(num):
for i in range(10-j):
print(“*”,end=” “)
print()
num=5
for j in range(1,11):
for i in range(1,11):
print(f”{i:<2} * {j:<2} = {j*i:<2}“,end=” “)
print()
# while
sum=0
for i in range(5):
num = int(input(“Enter the number: “))
sum=sum+num
print(“Sum is “,sum)
#WAP to input numbers from the user till they want and find sum
sum=0
ch=‘y’
while ch==‘y’:
num = int(input(“Enter the number: “))
sum = sum + num
ch=input(“Enter y to accept more values, anyother key to stop:”)
print(“Sum is”,sum)
#Another implementation:
#WAP to input numbers from the user till they want and find sum
sum=0
while True:
num = int(input(“Enter the number: “))
sum = sum + num
ch=input(“Enter y to accept more values, anyother key to stop:”)
if ch!=‘y’:
break #command that will throw you out of the loop
print(“Sum is”,sum)
var1 = 5 #let’s take a variable called var1 and make it equal to 5
# number without decimal point – integer
#var1 is a variable of type integer
print(“var1”)
print(var1)
print(type(var1))
var1 = 5.0 #float – -inf to +inf with decimal point
print(type(var1))
var1 = 5j #complex (imaginary) – i
# square root of -25 = sq rt(25* -1) = 5j
print(type(var1))
print(5j * 5j)
# string – text (str)
var1 = “hello”
print(type(var1))
#5th basic data types – bool (boolean – 2 values: True / False)
var1 = True
print(type(var1))
#Functions for explicit conversion – convert data from given type to this:
# int(), float(), complex(), str(), bool()
var1 = int(“5”)
print(type(var1))
quantity =49
cost_pc = 51.89
total_cost = quantity * cost_pc
print(“Total quantity bought is”,quantity,”each one at a cost of”,cost_pc,”so the total cost is”,total_cost)
print(f”Total quantity bought is {quantity} each one at a cost of {cost_pc:.1f} so the total cost is {total_cost}”)
pl=”Rohit”
cn=”India”
pos=”captain”
print(f”Player {pl:<12} plays for {cn:^12} and is {pos:>20} of the team!”)
pl=”Mabwange”
cn=”Zimbabwe”
pos=”Wicket-keeper”
print(f”Player {pl:<12} plays for {cn:^12} and is {pos:>20} of the team!”)
num1 = int(input(“Enter first number to be added: “)) #default input will read as str
print(type(num1))
num2 = int(input(“Enter second number to be added: “))
print(type(num2))
print(num1+num2)